filmov
tv
Integer to Roman - Leetcode 12 - Python
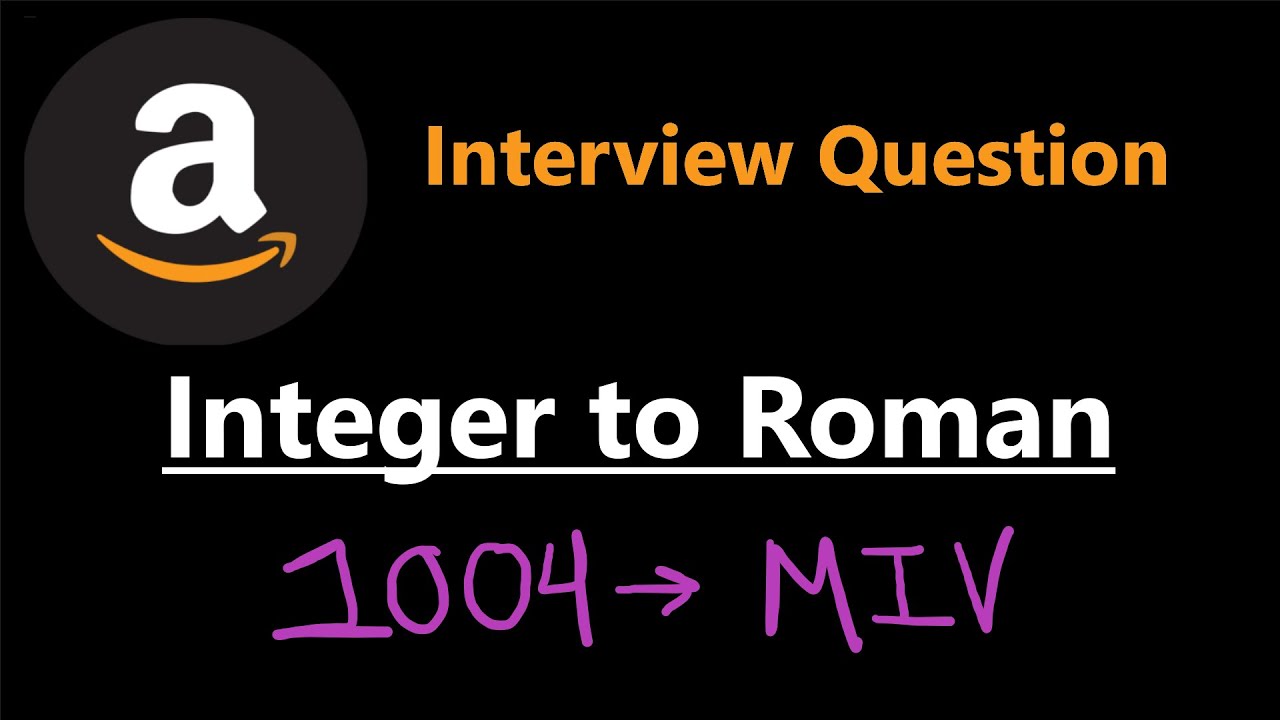
Показать описание
0:00 - Read the problem
1:40 - Drawing Explanation
6:25 - Coding Explanation
leetcode 12
#amazon #interview #python
Disclosure: Some of the links above may be affiliate links, from which I may earn a small commission.
Integer to Roman - Leetcode 12 - Python
Integer to Roman | Leetcode #12
LeetCode 12 | Integer to Roman (Java)
Leetcode - Integer to Roman (Python)
Roman to Integer | Leetcode -13 | Algorithms Made Easy
Roman to Integer - Leetcode 13 - Python
Integer to Roman | Live Coding with Explanation | Leetcode - 12
Convert Integer to Roman Numeral | Integer to Roman LeetCode | Programming Tutorials
Integer to Roman 🔥 | Leetcode 12 (Medium) | Java
Convert Integer to Roman numeral - LeetCode Interview Coding Challenge [Java Brains]
LeetCode 12 Integer To Roman | Strings | C++ [ Algorithm + Code explanation]
Integer to Roman - Leetcode 12 | Python | Hindi
Integer to Roman -(Amazon, Facebook, Microsoft, Twitter, Apple, Google..): Explanation ➕ Live Coding...
LeetCode Coding Problem | Integer to Roman
12. Integer to Roman | Integer To Roman Leetcode | Integer to Roman | Integer To Roman Leetcode C++
DSA Phir se with Sumeet | Leetcode 12 | Integer to Roman
Roman to Integer - Leetcode 13 - Arrays & Strings (Python)
Integer to Roman Python Solution - LeetCode #12
13. Roman to Integer. Решаем Leetcode на Python под бодрый фонк
[Решение на Java] 12. Integer to Roman. LeetCode задача для Amazon, Apple, Facebook, Microsoft и др....
Integer to Roman - LeetCode 12 - Coding Interview Questions
Amazon Coding Interview Question - Integer to Roman (LeetCode)
Leetcode - Roman to Integer (Python)
Integer To Roman - Convert From Java To Swift | LeetCode
Комментарии