filmov
tv
Roman to Integer - Leetcode 13 - Python
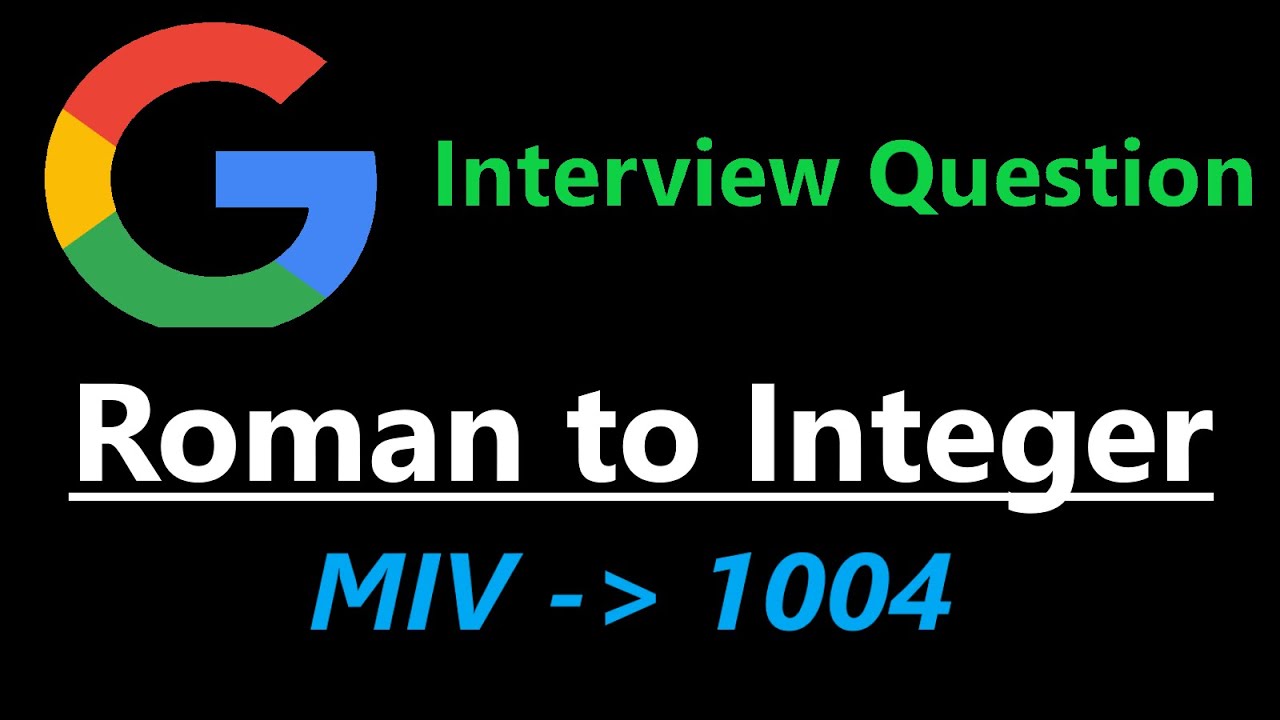
Показать описание
0:00 - Read the problem
1:40 - Drawing Explanation
5:34 - Coding Explanation
leetcode 13
#roman #integer #python
Disclosure: Some of the links above may be affiliate links, from which I may earn a small commission.
Roman to Integer - Leetcode 13 - Python
Roman to Integer | Leetcode -13 | Algorithms Made Easy
Roman to Integer - LeetCode 13 | Java
Roman to Integer - Leetcode 13 - Arrays & Strings (Python)
Leetcode - Roman to Integer (Python)
Integer to Roman - Leetcode 12 - Python
Convert Roman numeral to Integer - LeetCode Interview Coding Challenge [Java Brains]
Roman To Integer | Leetcode 13
Roman to Integer - LeetCode 13 - JavaScript
Roman To Integer - LeetCode 13 - Coding Interview Questions
LeetCode 13 | ROMAN TO INTEGER | C++ [ Approach and Code Explanation]
13. Roman to Integer || Java || Leetcode || Hindi
Integer to Roman | Leetcode #12
Roman to Integer | LeetCode 13 | Easy
LeetCode #13: Roman To Integer
Python Programming Practice: LeetCode #13 -- Roman to Integer
Leetcode Roman to Integer | Python
Leetcode | 13. Roman to Integer | Easy | Java Solution
Roman To Integer | Leetcode #13 | Live Code Explanation
13. Roman to Integer | LEETCODE | Top Interview Questions | EASY
Solving 'Roman to Integer' from LeetCode ( Easy ) - Problem solving in JS
GFG POTD: 04/10/2023 | Roman Number to Integer | Problem of the Day GeeksforGeeks
Roman Numbers to Integer Leetcode - Java Solution
Roman To Integer #LeetCode in #Javascript (#Arabic)
Комментарии