filmov
tv
Python function annotation specify possible parameter types with or
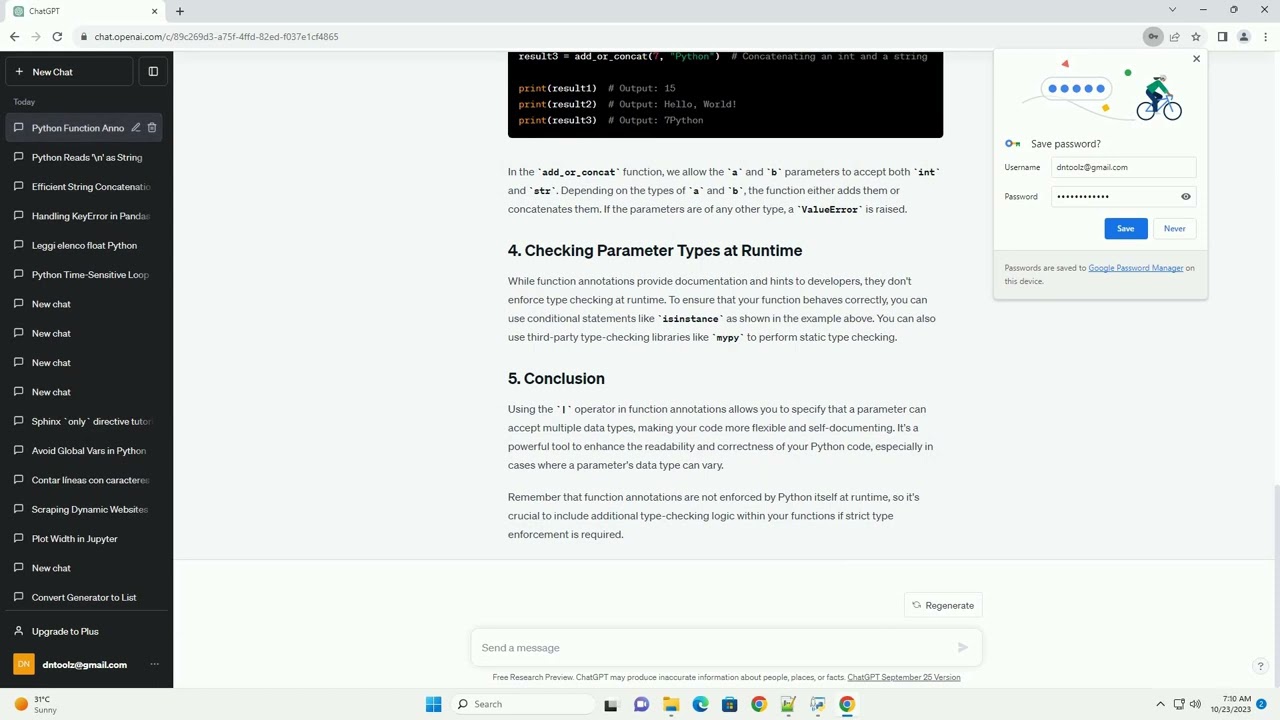
Показать описание
Function annotations in Python provide a way to specify the expected data types of function parameters and return values. Starting from Python 3.0, you can use the vertical bar | (OR) to indicate that a parameter can accept multiple data types. This allows you to be more flexible when defining the expected input for your functions. In this tutorial, we will explore how to use the | operator in function annotations with code examples.
Before we dive into using the | operator, let's review basic function annotations in Python. Function annotations are optional, but they provide valuable information about the types your functions expect and return. You can annotate both parameters and return values using colons after the parameter name and the - symbol before the return value type.
In the example above, we have a function greet that takes a name parameter of type str and returns a str. This provides clarity about the expected data types, making your code more self-documenting.
To specify that a parameter can accept multiple data types, you can use the | (OR) operator within the annotation. For example, if you want a parameter to accept both integers and floats, you can do the following:
In the calculate_tax function, the income parameter can accept both int and float. This flexibility can be quite useful in scenarios where the function should work with different numeric types.
Let's work through a practical example to see how | annotations can be used in a real-world scenario:
In the add_or_concat function, we allow the a and b parameters to accept both int and str. Depending on the types of a and b, the function either adds them or concatenates them. If the parameters are of any other type, a ValueError is raised.
While function annotations provide documentation and hints to developers, they don't enforce type checking at runtime. To ensure that your function behaves correctly, you can use conditional statements like isinstance as shown in the example above. You can also use third-party type-checking libraries like mypy to perform static type checking.
Using the | operator in function annotations allows you to specify that a parameter can accept multiple data types, making your code more flexible and self-documenting. It's a powerful tool to enhance the readability and correctness of your Python code, especially in cases where a parameter's data type can vary.
Remember that function annotations are not enforced by Python itself at runtime, so it's crucial t
Before we dive into using the | operator, let's review basic function annotations in Python. Function annotations are optional, but they provide valuable information about the types your functions expect and return. You can annotate both parameters and return values using colons after the parameter name and the - symbol before the return value type.
In the example above, we have a function greet that takes a name parameter of type str and returns a str. This provides clarity about the expected data types, making your code more self-documenting.
To specify that a parameter can accept multiple data types, you can use the | (OR) operator within the annotation. For example, if you want a parameter to accept both integers and floats, you can do the following:
In the calculate_tax function, the income parameter can accept both int and float. This flexibility can be quite useful in scenarios where the function should work with different numeric types.
Let's work through a practical example to see how | annotations can be used in a real-world scenario:
In the add_or_concat function, we allow the a and b parameters to accept both int and str. Depending on the types of a and b, the function either adds them or concatenates them. If the parameters are of any other type, a ValueError is raised.
While function annotations provide documentation and hints to developers, they don't enforce type checking at runtime. To ensure that your function behaves correctly, you can use conditional statements like isinstance as shown in the example above. You can also use third-party type-checking libraries like mypy to perform static type checking.
Using the | operator in function annotations allows you to specify that a parameter can accept multiple data types, making your code more flexible and self-documenting. It's a powerful tool to enhance the readability and correctness of your Python code, especially in cases where a parameter's data type can vary.
Remember that function annotations are not enforced by Python itself at runtime, so it's crucial t