filmov
tv
Binary Search Algorithm | Lecture-45 | Java and DSA Foundation course
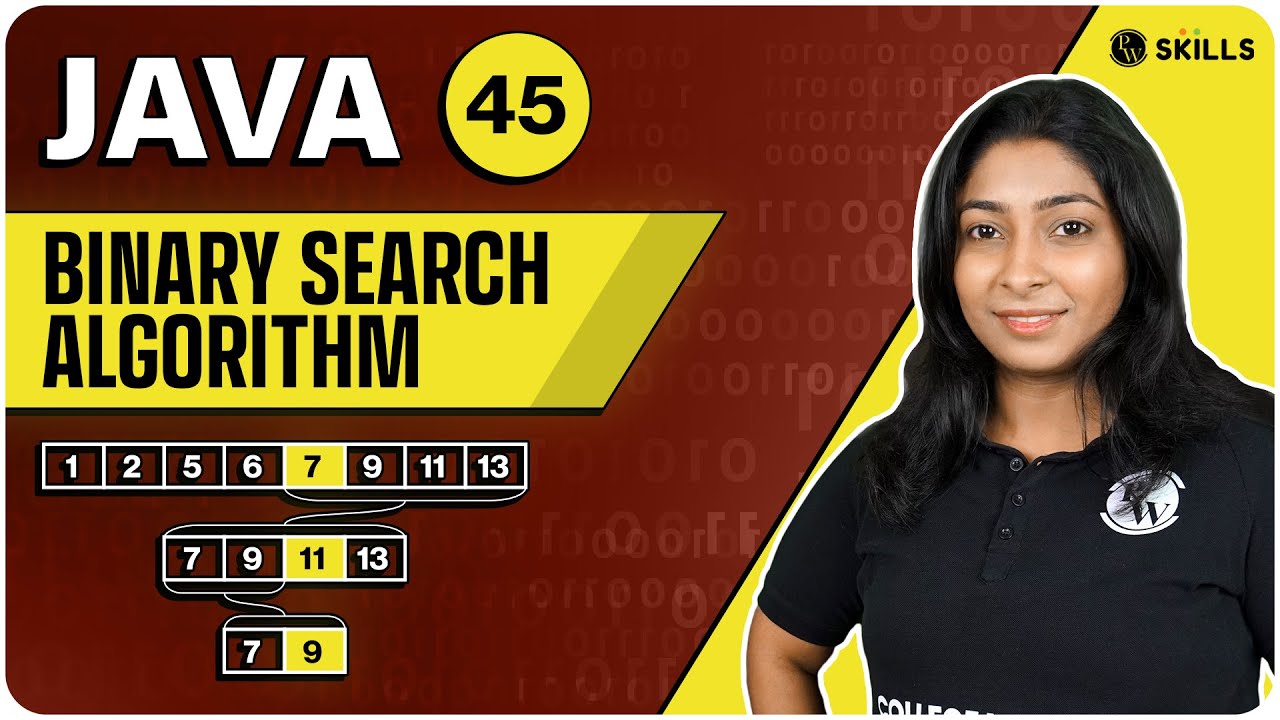
Показать описание
In this video we will dive into the world of Binary Search in Java. We'll cover the basics of the algorithm, including its implementation in Java and the steps involved in the process. In addition, we'll also be solving important problems related to binary search. Whether you're a beginner or an experienced Java programmer, this video is perfect for anyone who wants to learn about binary search or improve their skills. So grab your notepad, sit back, and let's get started on our journey of understanding Binary Search in Java. Happy Learning. See you all in the lecture.
PW Skills is announcing the launch of the following programs,
Binary Batch:- Java-with-DSA-&-System-Design (Java with DSA & System Design)
Sigma Batch:- Full-Stack-Web-Development (MERN Stack)
Impact Batch:- Data-Science-Masters (Full Stack Data Science)
TIME STAMPS:
00:00 - Intro
00:54 - Today's checklist
01:35 - Binary Search Algorithm
04:46 - Binary Search Algorithm Example
15:15 - Binary Search Algorithm Code
21:31 - Recursive binary search
26:05 - Recursive binary search Code
29:50 - Recursive binary search analysis
37:24 - Time complexity analysis
48:01 - PROBLEM 01: Find the first occurence of a given element.
55:05 - PROBLEM 01: Find the first occurence of a given element. Code.
59:15 - PROBLEM 02: Find the square root.
01:11:56 - PROBLEM 02: Find the square root. Code
01:15:50 - Summary
01:16:21 - Follow up questions
01:16:54 - Next Lecture
01:17:05 - Outro
#Coding #Java #Tutorial #Binary #Search #BinarySearch #ProblemSolving #Problem #Solving #PWSkills #CollegeWallah #Solution #DSA #DataStructure #Algorithm
PW Skills is announcing the launch of the following programs,
Binary Batch:- Java-with-DSA-&-System-Design (Java with DSA & System Design)
Sigma Batch:- Full-Stack-Web-Development (MERN Stack)
Impact Batch:- Data-Science-Masters (Full Stack Data Science)
TIME STAMPS:
00:00 - Intro
00:54 - Today's checklist
01:35 - Binary Search Algorithm
04:46 - Binary Search Algorithm Example
15:15 - Binary Search Algorithm Code
21:31 - Recursive binary search
26:05 - Recursive binary search Code
29:50 - Recursive binary search analysis
37:24 - Time complexity analysis
48:01 - PROBLEM 01: Find the first occurence of a given element.
55:05 - PROBLEM 01: Find the first occurence of a given element. Code.
59:15 - PROBLEM 02: Find the square root.
01:11:56 - PROBLEM 02: Find the square root. Code
01:15:50 - Summary
01:16:21 - Follow up questions
01:16:54 - Next Lecture
01:17:05 - Outro
#Coding #Java #Tutorial #Binary #Search #BinarySearch #ProblemSolving #Problem #Solving #PWSkills #CollegeWallah #Solution #DSA #DataStructure #Algorithm
Комментарии