filmov
tv
Premature Optimization
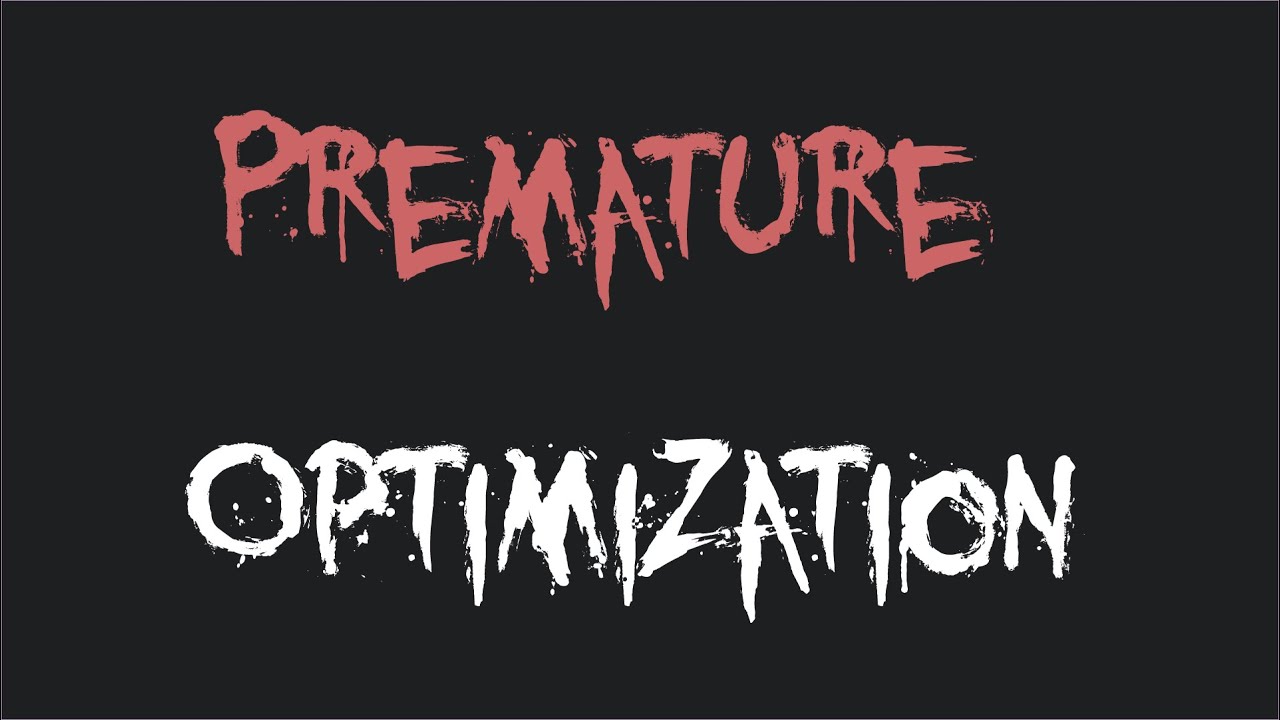
Показать описание
When should you optimize your code?
Premature Optimization
Premature Optimization
Premature optimization is the root of all evil | Donald Knuth and Lex Fridman
I HATE 'premature optimization is the root of all evil' (sometimes)
Prof. Hegarty talks about Premature Optimization (Donald Knuth) and KISS principle
Profressor Paul Hegarty talks about Premature Optimization (Knuth's Law)
Paul Hegarty on Premature Optimization
How to avoid premature optimization in software development
STOP Making These 3 HUGE Mistakes in Software Development
Designing for Performance - is Not as Hard as You Think !
Quick Tips to Avoid Overengineering: The Pitfalls of Premature Optimization
Fastware - Premature optimization?
Handmade Hero | Premature Optimization
Avoid Premature Optimization By Generating Test Data
Harmful GOTOs, Premature Optimizations and Programming Myths (...) - Alvaro Videla
230. Premature Optimization vs Careful Planning - How To Optimize Your Application
Root of all Evil? - Optimization Disagreement - Premature?
Premature Optimization in Scala
Why Premature Optimization Is Considered the Root of All Evil and How to Avoid It
Why are so many programmers against optimization?
How Premature Optimization Helped me Avoid This Training Mistake
Brian Shirai - Types As Premature Optimization
Premature Optimization
Calvinism and Premature Optimization
Комментарии