filmov
tv
JavaScript Algorithms - 7 - Fibonacci Sequence
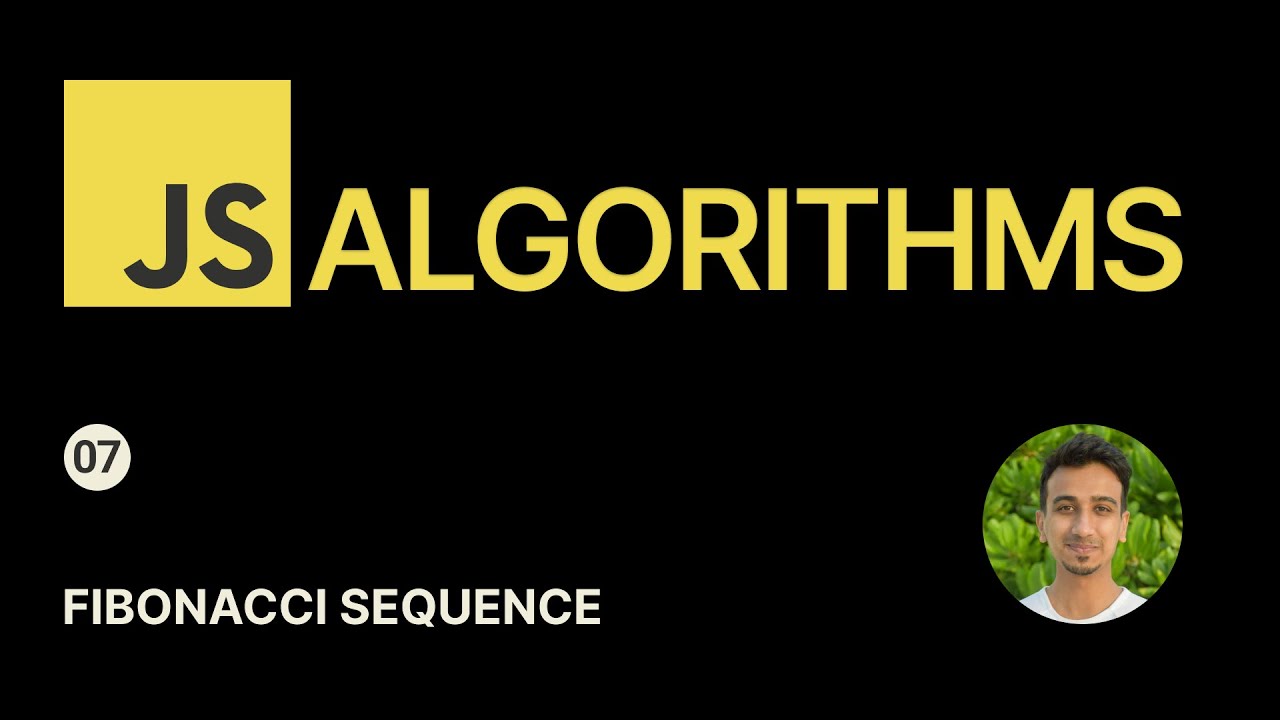
Показать описание
📱 Follow Codevolution
Fibonacci Sequence
JavaScript Algorithms
Algorithms with JavaScript
JavaScript Algorithms - 7 - Fibonacci Sequence
Javascript Algorithms : #7 : Factorial of a Number
JavaScript Algorithms - 7 Fibonacci Sequence #javascript
Top 10 Javascript Algorithms to Prepare for Coding Interviews
Javascript Freecodecamp Algorithm #7: Search and Replace
JavaScript Algorithms Crash Course - Learn Algorithms & 'Big O' from the Ground Up!
Top 7 Algorithms for Coding Interviews Explained SIMPLY
JavaScript Algorithms - 1 - Introduction
151.1 Reverse Words in a String (Theory) | #7 | Array And String Playlist | #leetcode #dsa #strings
JavaScript Algorithms for Beginners
I gave 127 interviews. Top 5 Algorithms they asked me.
Graph Search Algorithms in 100 Seconds - And Beyond with JS
JavaScript Algorithms and Data Structures Masterclass (Part 1)
Best Way to Create a Selection Sort Algorithm - JavaScript
Data Structures and Algorithms in JavaScript - Full Course for Beginners
Introduction to Big O Notation and Time Complexity (Data Structures & Algorithms #7)
10 MOST POPULAR JAVASCRIPT ALGORITHMS
JavaScript: 7 String Methods
Day-7: JavaScript Algorithms and Data Structures #javascriptframework #freecodecamp
How to ACTUALLY Master Data Structures FAST (with real coding examples)
JavaScript Algorithms - 5 - Objects and Arrays Big-O
Bubble Sort - Coding Interview Question - JavaScript
Learning JavaScript Algorithms and Data Structures at freeCodeCamp - Part 7
JavaScript Algorithms - 8 - Factorial of a Number
Комментарии