filmov
tv
Optimizing 3x Nested Loop to Prevent MemoryError with Large Datasets
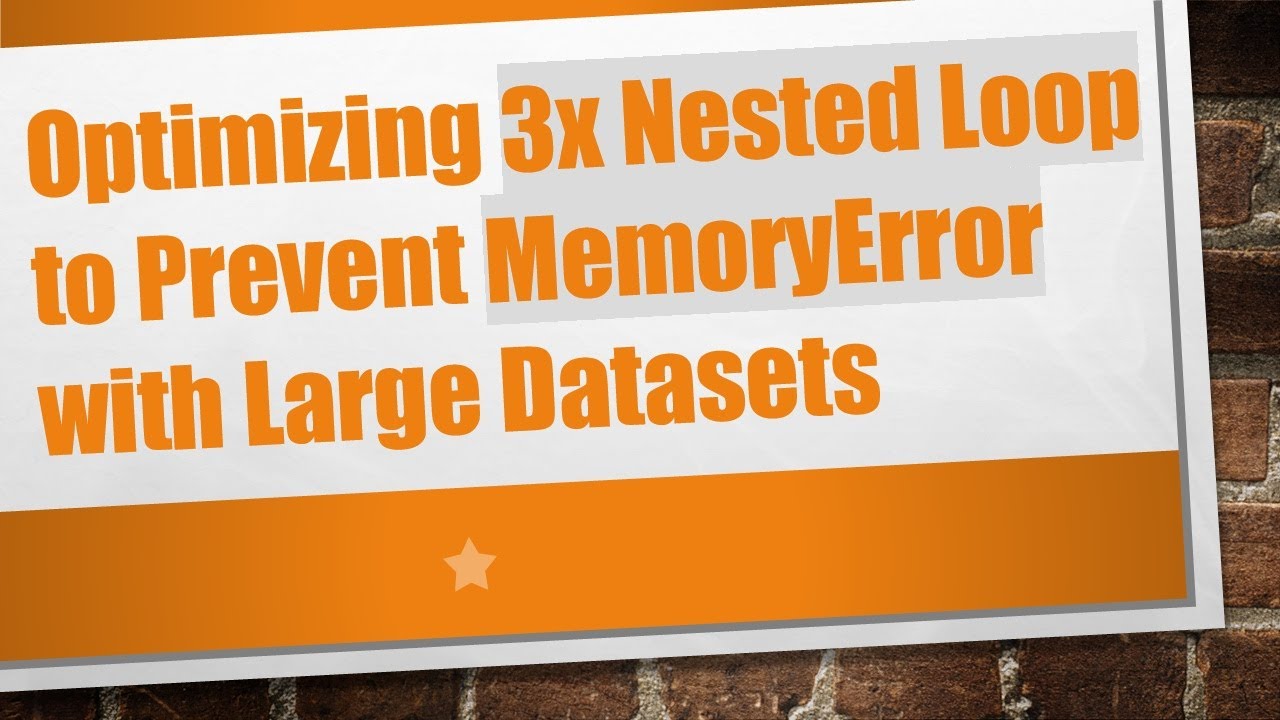
Показать описание
Learn how to efficiently optimize nested loops in Python to handle large datasets without running into memory errors.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Optimizing 3x nested loop to avoid MemoryError working with big datasets
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Introduction
Working with large datasets can be a daunting task for many data enthusiasts and developers. One common issue encountered is the infamous MemoryError, particularly when dealing with structures like nested loops. This can leave you scratching your head, trying to figure out how to optimize your code efficiently.
In this guide, we will explore a specific case involving two dataframes containing configurations of laptops and PCs. We will identify the problem of inefficient looping and provide practical solutions using Python, particularly with the assistance of libraries like Pandas.
The Problem
Imagine you have two dataframes, df_original and df_compare, each containing the specifications of laptops. The goal is to compare the configurations of each item from df_original against all items in df_compare. By doing so, we want to calculate a "weight" that quantifies how similar the configurations are based on certain criteria.
Your current approach involves a 3x nested loop, which is not only slowing down the execution but also causing memory overflow due to the size of the datasets—each containing around 200,000 rows.
Example DataFrames
Here's what our dataframes look like:
DataFrame One (df_original)
processorNameGraphicsCardnameProcessorBrand5950xRtx 3060 tii73600Rtx 3090i71165g7Rtx 3050i5DataFrame Two (df_compare)
processorNameGraphicsCardnameProcessorBrand5950xRtx 3090i71165g7Rtx 3060 tii71165g7Rtx 3050i5The challenge is to compare each row of df_original with those in df_compare, and calculate a weight based on how many columns' values differ. A key detail is that the processorName has a weight of 2, and we have similar weights for other columns.
The Solution
Avoiding Nested Loops
Instead of using nested loops, we can utilize list comprehensions alongside Pandas' vectorized operations to enhance performance and reduce memory consumption. Below are two sample code snippets demonstrating different approaches based on your needs.
Basic Weight Calculation
Assuming each column has a weight of 2, you can use the following code:
[[See Video to Reveal this Text or Code Snippet]]
Here’s how it works:
val != df_compare: This creates a boolean dataframe showing where values differ.
* 2: This multiplies the boolean values by 2, giving a weight measure.
sum(axis=1): This computes the total weight for each row comparison.
Example Output:
[[See Video to Reveal this Text or Code Snippet]]
Custom Weights Calculation
For situations where each column might have different weights, you can create a custom dictionary for weights and use it for the calculations:
[[See Video to Reveal this Text or Code Snippet]]
In this case:
.replace(weights): Replaces boolean values with the corresponding weights specified in the dictionary.
Example Output:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By refactoring your code to utilize list comprehensions and vectorized operations provided by Pandas, you can solve the MemoryError issue while significantly speeding up the computations needed for your large datasets. This not only makes your code more efficient but also improves its readability and maintainability.
If you ever find yourself facing the limits of Python's loops with large data, remember that there are often cleaner and faster solutions waiting in the data analysis libraries. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Optimizing 3x nested loop to avoid MemoryError working with big datasets
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Introduction
Working with large datasets can be a daunting task for many data enthusiasts and developers. One common issue encountered is the infamous MemoryError, particularly when dealing with structures like nested loops. This can leave you scratching your head, trying to figure out how to optimize your code efficiently.
In this guide, we will explore a specific case involving two dataframes containing configurations of laptops and PCs. We will identify the problem of inefficient looping and provide practical solutions using Python, particularly with the assistance of libraries like Pandas.
The Problem
Imagine you have two dataframes, df_original and df_compare, each containing the specifications of laptops. The goal is to compare the configurations of each item from df_original against all items in df_compare. By doing so, we want to calculate a "weight" that quantifies how similar the configurations are based on certain criteria.
Your current approach involves a 3x nested loop, which is not only slowing down the execution but also causing memory overflow due to the size of the datasets—each containing around 200,000 rows.
Example DataFrames
Here's what our dataframes look like:
DataFrame One (df_original)
processorNameGraphicsCardnameProcessorBrand5950xRtx 3060 tii73600Rtx 3090i71165g7Rtx 3050i5DataFrame Two (df_compare)
processorNameGraphicsCardnameProcessorBrand5950xRtx 3090i71165g7Rtx 3060 tii71165g7Rtx 3050i5The challenge is to compare each row of df_original with those in df_compare, and calculate a weight based on how many columns' values differ. A key detail is that the processorName has a weight of 2, and we have similar weights for other columns.
The Solution
Avoiding Nested Loops
Instead of using nested loops, we can utilize list comprehensions alongside Pandas' vectorized operations to enhance performance and reduce memory consumption. Below are two sample code snippets demonstrating different approaches based on your needs.
Basic Weight Calculation
Assuming each column has a weight of 2, you can use the following code:
[[See Video to Reveal this Text or Code Snippet]]
Here’s how it works:
val != df_compare: This creates a boolean dataframe showing where values differ.
* 2: This multiplies the boolean values by 2, giving a weight measure.
sum(axis=1): This computes the total weight for each row comparison.
Example Output:
[[See Video to Reveal this Text or Code Snippet]]
Custom Weights Calculation
For situations where each column might have different weights, you can create a custom dictionary for weights and use it for the calculations:
[[See Video to Reveal this Text or Code Snippet]]
In this case:
.replace(weights): Replaces boolean values with the corresponding weights specified in the dictionary.
Example Output:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By refactoring your code to utilize list comprehensions and vectorized operations provided by Pandas, you can solve the MemoryError issue while significantly speeding up the computations needed for your large datasets. This not only makes your code more efficient but also improves its readability and maintainability.
If you ever find yourself facing the limits of Python's loops with large data, remember that there are often cleaner and faster solutions waiting in the data analysis libraries. Happy coding!