filmov
tv
Dividing Array Elements in Python: A Conditional Approach Using NumPy
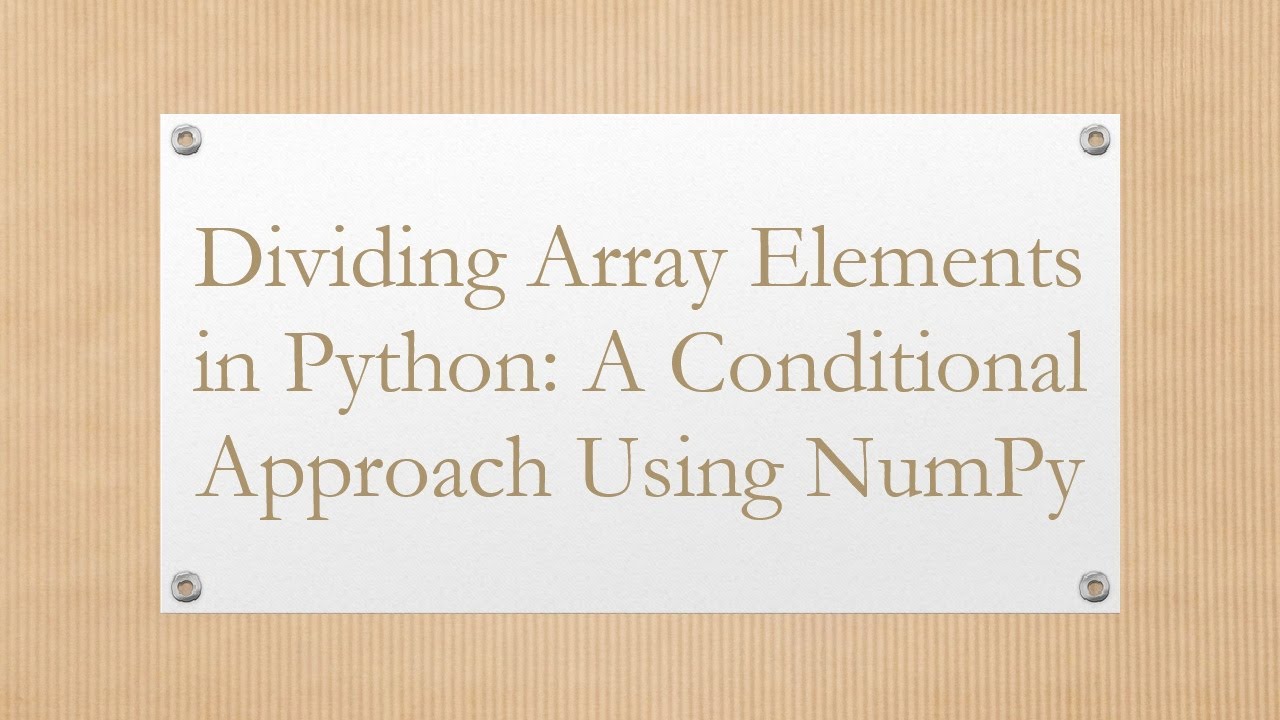
Показать описание
Learn how to divide elements of two arrays in Python based on specific conditions using NumPy. Get insights into using logical indexing and efficient array operations.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Python: how how to divide elements of arrays based on condition?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Dividing Array Elements in Python: A Conditional Approach Using NumPy
When working with arrays in Python, especially with NumPy, you might encounter situations where you need to perform operations based on certain conditions. A common problem is dividing elements of one array by another, but only under specific circumstances. In this guide, we will explore how to handle such scenarios effectively using NumPy.
The Problem at Hand
Let’s consider a specific example. You have two arrays, F and P, of the same dimensions, and you want to divide each element of the F array by the corresponding element in the P array only when the values in F are less than 100. Here's the setup:
[[See Video to Reveal this Text or Code Snippet]]
In this example, we want to handle arrays F and P, distinguishing between the elements in F that are under the threshold of 100 and performing the division accordingly.
The Solution
Step 1: Understanding Condition Checks
To start, we need to determine which elements of the array F are less than 100. This can be achieved using NumPy’s conditional checks which return a boolean array. For instance:
[[See Video to Reveal this Text or Code Snippet]]
This logical condition will help us filter the elements for our operation.
Step 2: Dividing with Conditions
Using the logical array from Step 1, we can set up our division operation. The formula can be expressed as:
[[See Video to Reveal this Text or Code Snippet]]
Alternatively, you can express it as:
[[See Video to Reveal this Text or Code Snippet]]
This formula efficiently consists of three main parts:
First Part: (F < 100) * (F / P) computes the division where the condition is satisfied.
Second Part: (F >= 100) * F ensures that elements greater than or equal to 100 are unchanged.
Combining those parts: The final result combines both conditions.
Step 3: Final Output
When you run this code with the arrays provided, you will receive the following output:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
In this guide, we outlined how to divide elements of two arrays based on specific conditions in Python, using NumPy. By leveraging NumPy’s powerful functionality for logical operations and manipulating arrays, we can perform such selective computations efficiently.
If you encounter similar problems working with arrays in your projects, remember this approach, and it will surely simplify your tasks. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Python: how how to divide elements of arrays based on condition?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Dividing Array Elements in Python: A Conditional Approach Using NumPy
When working with arrays in Python, especially with NumPy, you might encounter situations where you need to perform operations based on certain conditions. A common problem is dividing elements of one array by another, but only under specific circumstances. In this guide, we will explore how to handle such scenarios effectively using NumPy.
The Problem at Hand
Let’s consider a specific example. You have two arrays, F and P, of the same dimensions, and you want to divide each element of the F array by the corresponding element in the P array only when the values in F are less than 100. Here's the setup:
[[See Video to Reveal this Text or Code Snippet]]
In this example, we want to handle arrays F and P, distinguishing between the elements in F that are under the threshold of 100 and performing the division accordingly.
The Solution
Step 1: Understanding Condition Checks
To start, we need to determine which elements of the array F are less than 100. This can be achieved using NumPy’s conditional checks which return a boolean array. For instance:
[[See Video to Reveal this Text or Code Snippet]]
This logical condition will help us filter the elements for our operation.
Step 2: Dividing with Conditions
Using the logical array from Step 1, we can set up our division operation. The formula can be expressed as:
[[See Video to Reveal this Text or Code Snippet]]
Alternatively, you can express it as:
[[See Video to Reveal this Text or Code Snippet]]
This formula efficiently consists of three main parts:
First Part: (F < 100) * (F / P) computes the division where the condition is satisfied.
Second Part: (F >= 100) * F ensures that elements greater than or equal to 100 are unchanged.
Combining those parts: The final result combines both conditions.
Step 3: Final Output
When you run this code with the arrays provided, you will receive the following output:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
In this guide, we outlined how to divide elements of two arrays based on specific conditions in Python, using NumPy. By leveraging NumPy’s powerful functionality for logical operations and manipulating arrays, we can perform such selective computations efficiently.
If you encounter similar problems working with arrays in your projects, remember this approach, and it will surely simplify your tasks. Happy coding!