filmov
tv
Kth Largest Element in an Array - Quick Select - Leetcode 215 - Python
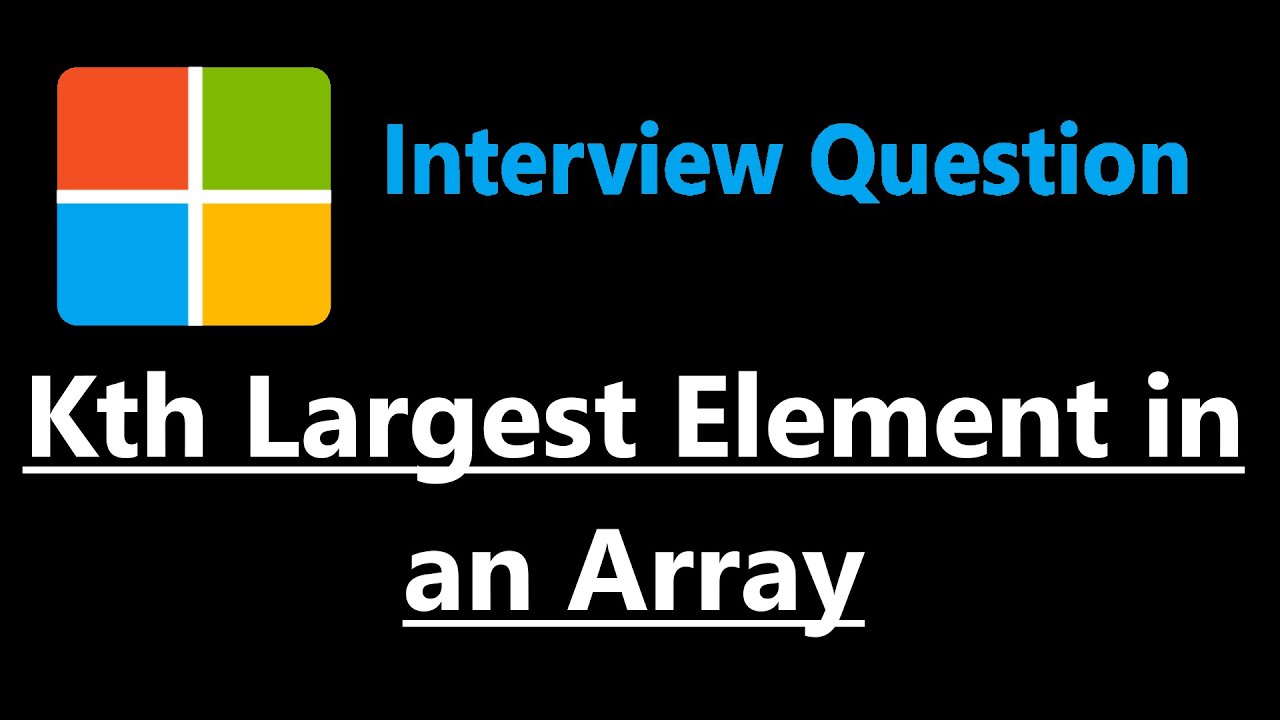
Показать описание
0:00 - Read the problem
3:39 - Drawing Explanation
11:40 - Time Complexity
13:25 - Coding Explanation
leetcode 215
#quick #select #python
Disclosure: Some of the links above may be affiliate links, from which I may earn a small commission.
Kth Largest Element in an Array - Quick Select - Leetcode 215 - Python
Kth Largest Element in a Stream - Leetcode 703 - Python
Kth Largest Element in an Array - Leetcode 215 - Heaps (Python)
How to find the kth largest element in an array? (LeetCode .215) - Inside code
Kth largest element in an array | Kth smallest element in an array
Leetcode - Kth Largest Element in an Array (Python)
Kth LARGEST ELEMENT IN AN ARRAY - SOLUTION EXPLAINED [PYTHON]
Kth Largest Element in an Array | Live Coding with Explanation | Leetcode - 215
Find the Kth Largest Element in an Array.
Find the k'th Largest or Smallest Element of an Array: From Sorting To Heaps To Partitioning
215. Kth Largest Element in an Array | Day 014 | 3 Ways | Quick Select | Min Heap | Sorting
Kth Largest Element in an Array
Kth Largest Element in an Array | Heap | Sorting | 2 Approaches | Time Complexity | Leetcode-215
3 Return K largest Elements in array
How to Find kth Smallest & Largest Element in Array
Find Kth Largest/Smallest Element in an Array | PriorityQueue in Java & C++ | DSA-One Course #33
FACEBOOK ASKED THIS CODING QUESTION 127 TIMES!!! | Kth Largest Element in an Array - Leetcode 215
Leetcode Kth Largest Element in an Array | Solving AlgoPrep 151 | Nishant Chahar
Kth Largest Element in an Array | Quick Select | Time Complexity | MICROSOFT | Leetcode-215
BST - 18: Get Kth Largest element in given Binary Search Tree (BST)
Kth Largest Element in a Stream | Heap | AMAZON | Leetcode-703 | Explanation ➕ Live Coding 🧑🏻💻...
Leetcode - Kth Largest Element in a Stream (Python)
L45. K-th Smallest/Largest Element in BST
703. Kth Largest Element in a Stream | Leetcode Daily Challenge | DSA | Hindi
Комментарии