filmov
tv
OpenCV error for reading a video file with python 2 7
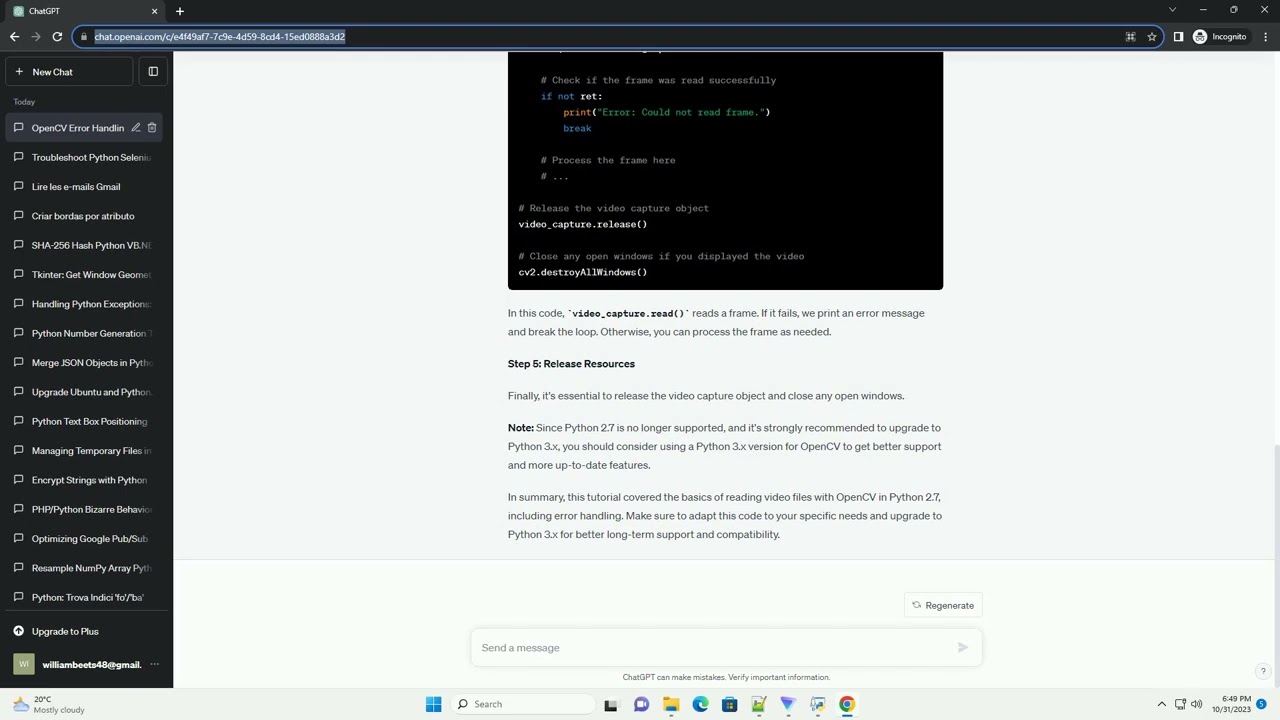
Показать описание
I'd be happy to help you with an informative tutorial on handling OpenCV errors when reading a video file in Python 2.7. However, please note that Python 2.7 is no longer supported as of January 1, 2020, and it's highly recommended to upgrade to a more recent version of Python, such as Python 3.x. OpenCV is also better supported in Python 3.x. Nevertheless, I'll provide a tutorial for Python 2.7 as you requested.
In this tutorial, you'll learn how to use the OpenCV library in Python 2.7 to read a video file and handle common errors that may occur during the process. We'll also provide code examples to illustrate the concepts.
Step 1: Install OpenCV for Python 2.7
If you haven't already, you need to install OpenCV for Python 2.7. You can use pip to install it:
Step 2: Import OpenCV and Python 2.7 Modules
Step 3: Read a Video File
Now, let's write code to read a video file. We'll use cv2.VideoCapture() for this purpose:
Here, we check if the video file was opened successfully using isOpened(). If it fails, we print an error message and exit the program.
Step 4: Read Frames
To read frames from the video, you can use a loop. The loop will continue until there are no more frames to read. In this step, we handle potential errors related to reading frames.
Step 5: Release Resources
Finally, it's essential to release the video capture object and close any open windows.
Note: Since Python 2.7 is no longer supported, and it's strongly recommended to upgrade to Python 3.x, you should consider using a Python 3.x version for OpenCV to get better support and more up-to-date features.
In summary, this tutorial covered the basics of reading video files with OpenCV in Python 2.7, including error handling. Make sure to adapt this code to your specific needs and upgrade to Python 3.x for better long-term support and compatibility.
ChatGPT
In this tutorial, you'll learn how to use the OpenCV library in Python 2.7 to read a video file and handle common errors that may occur during the process. We'll also provide code examples to illustrate the concepts.
Step 1: Install OpenCV for Python 2.7
If you haven't already, you need to install OpenCV for Python 2.7. You can use pip to install it:
Step 2: Import OpenCV and Python 2.7 Modules
Step 3: Read a Video File
Now, let's write code to read a video file. We'll use cv2.VideoCapture() for this purpose:
Here, we check if the video file was opened successfully using isOpened(). If it fails, we print an error message and exit the program.
Step 4: Read Frames
To read frames from the video, you can use a loop. The loop will continue until there are no more frames to read. In this step, we handle potential errors related to reading frames.
Step 5: Release Resources
Finally, it's essential to release the video capture object and close any open windows.
Note: Since Python 2.7 is no longer supported, and it's strongly recommended to upgrade to Python 3.x, you should consider using a Python 3.x version for OpenCV to get better support and more up-to-date features.
In summary, this tutorial covered the basics of reading video files with OpenCV in Python 2.7, including error handling. Make sure to adapt this code to your specific needs and upgrade to Python 3.x for better long-term support and compatibility.
ChatGPT