filmov
tv
JavaScript Destructuring Simplified 🔥🔥 | JavaSrcipt Basics
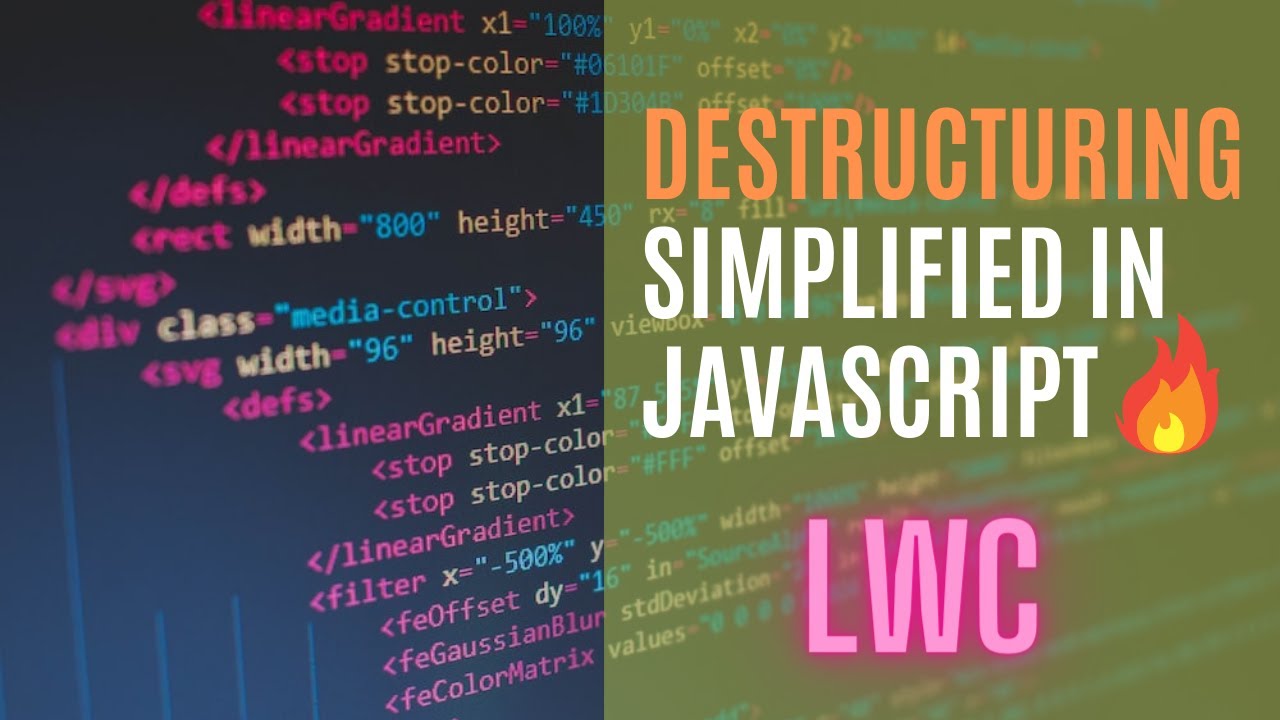
Показать описание
Destructuring is a feature in JavaScript that allows you to extract values from arrays or properties from objects and assign them to variables in a concise and convenient way.
With destructuring, you can easily unpack values from arrays or objects into separate variables, eliminating the need for repetitive indexing or property access.
Here's an example of array destructuring:
```javascript
const numbers = [1, 2, 3];
// Extract values from the array
const [a, b, c] = numbers;
```
In this example, the values 1, 2, and 3 are extracted from the `numbers` array and assigned to the variables `a`, `b`, and `c` respectively.
Object destructuring works similarly but extracts values based on property names:
```javascript
const person = { name: 'John', age: 25 };
// Extract properties from the object
const { name, age } = person;
```
In this case, the properties `name` and `age` of the `person` object are extracted and assigned to the variables `name` and `age`.
Destructuring can also be used in function parameters to extract values directly:
```javascript
// Function with array destructuring
function greet([firstName, lastName]) {
}
const fullName = ['John', 'Doe'];
greet(fullName); // Output: Hello, John Doe!
// Function with object destructuring
function displayInfo({ name, age }) {
}
const person = { name: 'Alice', age: 30 };
displayInfo(person); // Output: Name: Alice, Age: 30
```
Destructuring is a powerful feature that simplifies code and makes it more readable when working with arrays and objects in JavaScript.
With destructuring, you can easily unpack values from arrays or objects into separate variables, eliminating the need for repetitive indexing or property access.
Here's an example of array destructuring:
```javascript
const numbers = [1, 2, 3];
// Extract values from the array
const [a, b, c] = numbers;
```
In this example, the values 1, 2, and 3 are extracted from the `numbers` array and assigned to the variables `a`, `b`, and `c` respectively.
Object destructuring works similarly but extracts values based on property names:
```javascript
const person = { name: 'John', age: 25 };
// Extract properties from the object
const { name, age } = person;
```
In this case, the properties `name` and `age` of the `person` object are extracted and assigned to the variables `name` and `age`.
Destructuring can also be used in function parameters to extract values directly:
```javascript
// Function with array destructuring
function greet([firstName, lastName]) {
}
const fullName = ['John', 'Doe'];
greet(fullName); // Output: Hello, John Doe!
// Function with object destructuring
function displayInfo({ name, age }) {
}
const person = { name: 'Alice', age: 30 };
displayInfo(person); // Output: Name: Alice, Age: 30
```
Destructuring is a powerful feature that simplifies code and makes it more readable when working with arrays and objects in JavaScript.