filmov
tv
JavaScript DESTRUCTURING in 8 minutes! 💥
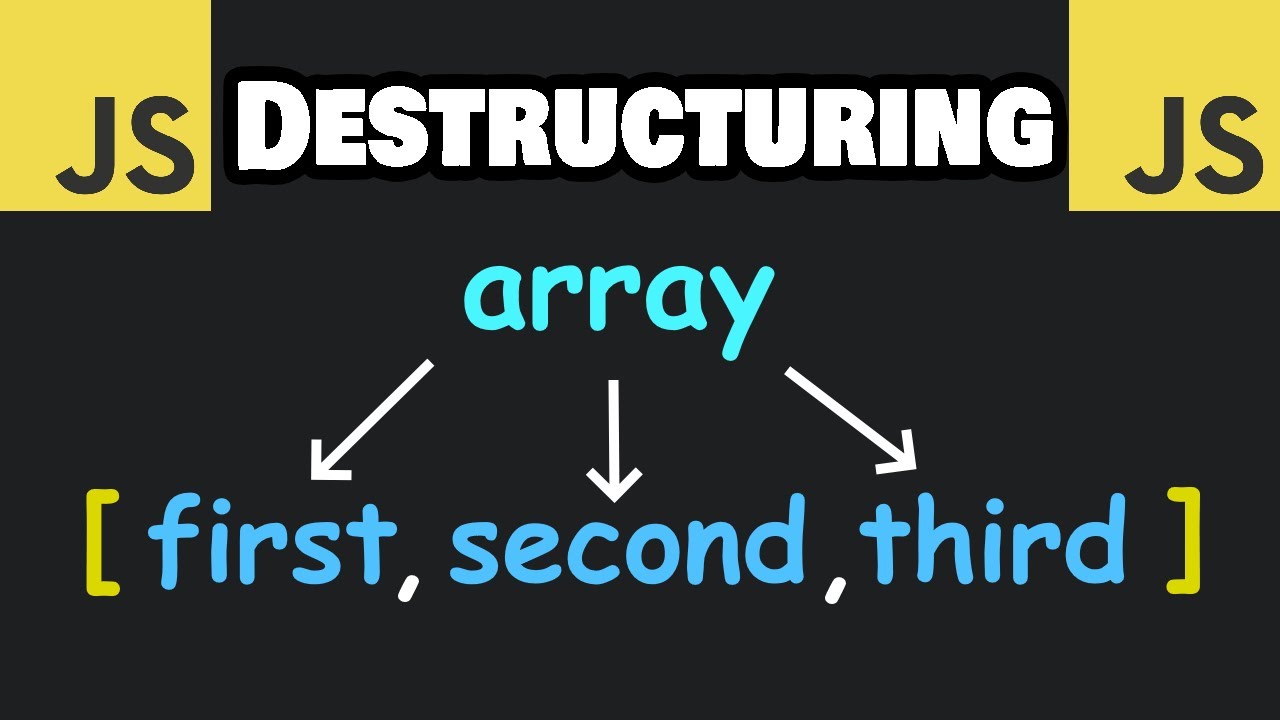
Показать описание
00:00:00 intro
00:00:21 swap the values of two variables
00:01:11 swap two elements in an array
00:02:34 assign array elements to variables
00:03:47 extract values from objects
00:06:11 destructuring in function parameters
// destructuring = extract values from arrays and objects,
// then assign them to variables in a convenient way
// [] = to perform array destructuring
// {} = to perform object destructuring
// ---------- EXAMPLE 1 ----------
// SWAP THE VALUE OF TWO VARIABLES
let a = 1;
let b = 2;
[a, b] = [b, a];
// ---------- EXAMPLE 2 ----------
// SWAP 2 ELEMENTS IN AN ARRAY
const colors = ['red', 'green', 'blue', 'black', 'white'];
[colors[0], colors[4]] = [colors[4], colors[0]]
// ---------- EXAMPLE 3 ----------
// ASSIGN ARRAY ELEMENTS TO VARIABLES
const [firstColor, secondColor, thirdColor, ...extraColors] = colors;
// ---------- EXAMPLE 4 ----------
// EXTRACT VALUES FROM OBJECTS
const person1 = {
firstName: 'Spongebob',
lastName: 'Squarepants',
age: 30,
job: "Fry cook",
};
const person2 = {
firstName: 'Patrick',
lastName: 'Star',
age: 34
};
const {firstName, lastName, age, job="Unemployed"} = person2;
// ---------- EXAMPLE 5 ----------
// DESTRUCTURING IN FUNCTION PARAMETERS
function displayPerson({ firstName, lastName, age, job="Unemployed" }) {
}
displayPerson(person1);
displayPerson(person2);
00:00:21 swap the values of two variables
00:01:11 swap two elements in an array
00:02:34 assign array elements to variables
00:03:47 extract values from objects
00:06:11 destructuring in function parameters
// destructuring = extract values from arrays and objects,
// then assign them to variables in a convenient way
// [] = to perform array destructuring
// {} = to perform object destructuring
// ---------- EXAMPLE 1 ----------
// SWAP THE VALUE OF TWO VARIABLES
let a = 1;
let b = 2;
[a, b] = [b, a];
// ---------- EXAMPLE 2 ----------
// SWAP 2 ELEMENTS IN AN ARRAY
const colors = ['red', 'green', 'blue', 'black', 'white'];
[colors[0], colors[4]] = [colors[4], colors[0]]
// ---------- EXAMPLE 3 ----------
// ASSIGN ARRAY ELEMENTS TO VARIABLES
const [firstColor, secondColor, thirdColor, ...extraColors] = colors;
// ---------- EXAMPLE 4 ----------
// EXTRACT VALUES FROM OBJECTS
const person1 = {
firstName: 'Spongebob',
lastName: 'Squarepants',
age: 30,
job: "Fry cook",
};
const person2 = {
firstName: 'Patrick',
lastName: 'Star',
age: 34
};
const {firstName, lastName, age, job="Unemployed"} = person2;
// ---------- EXAMPLE 5 ----------
// DESTRUCTURING IN FUNCTION PARAMETERS
function displayPerson({ firstName, lastName, age, job="Unemployed" }) {
}
displayPerson(person1);
displayPerson(person2);