filmov
tv
Mastering LINQ: Converting Integers and Lists to Strings in C#
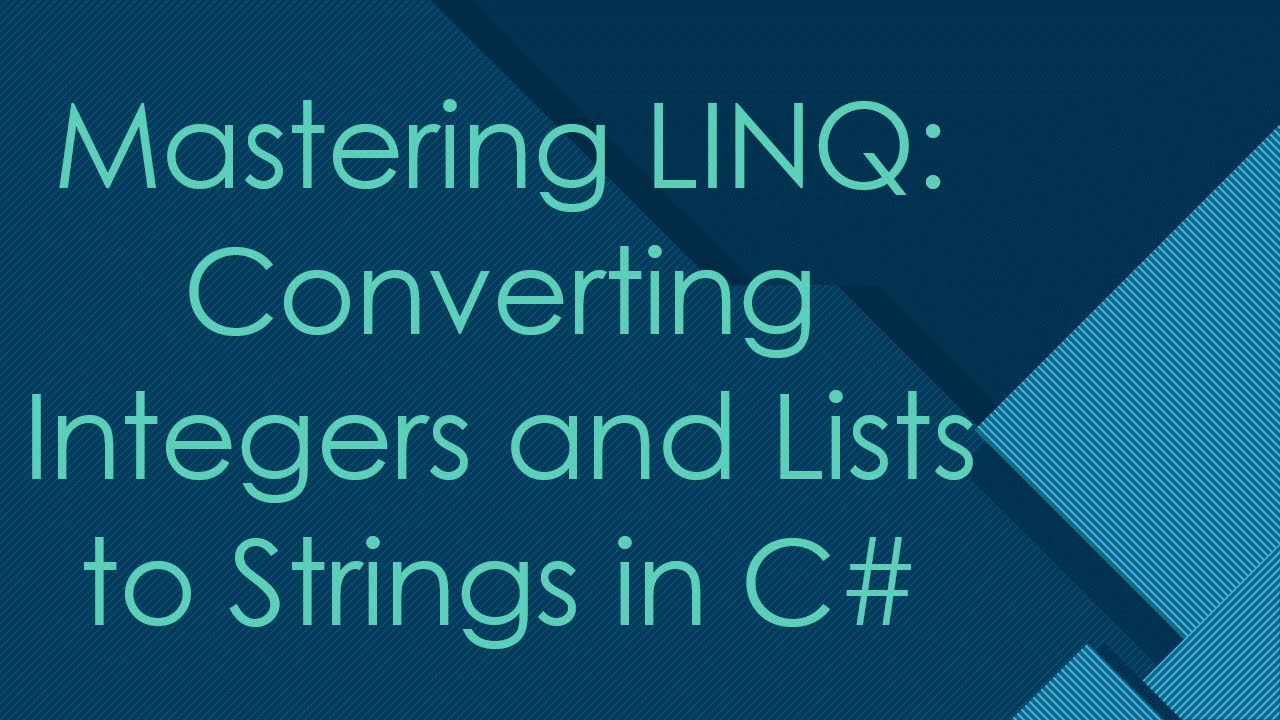
Показать описание
Summary: Learn how to efficiently convert integers and lists of integers to strings using LINQ in C#. This guide covers practical examples, including converting lists to comma-separated strings.
---
Mastering LINQ: Converting Integers and Lists to Strings in C
Introduction
LINQ (Language Integrated Query) is a powerful feature in C that enables you to query and manipulate data in a readable and concise way. One common task developers face is converting integers or lists of integers into strings. This guide will guide you through different approaches to achieve this using LINQ.
Converting an Integer to a String
While converting a single integer to a string can be easily done using the ToString() method, LINQ provides a more elegant solution, especially when dealing with multiple conversions. For a single integer:
[[See Video to Reveal this Text or Code Snippet]]
Converting a List of Integers to a String
When you need to convert a list of integers to a single string, LINQ can simplify the operation. Here's how you can convert a list of integers to a space-separated string:
[[See Video to Reveal this Text or Code Snippet]]
In this example, the Select method is used to convert each integer in the list to a string, and the string.Join method concatenates them with a space in between.
Converting a List of Integers to a Comma-Separated String
Another common requirement is to convert a list of integers into a comma-separated string. LINQ makes this task straightforward:
[[See Video to Reveal this Text or Code Snippet]]
Just like the previous example, the Select method converts each integer to a string, and string.Join creates the comma-separated result.
Advanced Use Case: Conditional Formatting
Suppose you want to format the numbers conditionally. For instance, you might want to surround negative numbers with parentheses:
[[See Video to Reveal this Text or Code Snippet]]
This example uses a ternary operator within the Select method to apply conditional formatting based on the value of each integer.
Conclusion
Using LINQ for converting integers and lists of integers to strings in C allows for clean, readable code. Whether you're working with single integers, lists, or applying conditional formatting, LINQ offers a versatile and elegant approach to these common tasks.
LINQ's rich feature set ensures that you can handle a wide range of data manipulation tasks efficiently, making it an essential tool in the C programmer's toolkit.
---
Mastering LINQ: Converting Integers and Lists to Strings in C
Introduction
LINQ (Language Integrated Query) is a powerful feature in C that enables you to query and manipulate data in a readable and concise way. One common task developers face is converting integers or lists of integers into strings. This guide will guide you through different approaches to achieve this using LINQ.
Converting an Integer to a String
While converting a single integer to a string can be easily done using the ToString() method, LINQ provides a more elegant solution, especially when dealing with multiple conversions. For a single integer:
[[See Video to Reveal this Text or Code Snippet]]
Converting a List of Integers to a String
When you need to convert a list of integers to a single string, LINQ can simplify the operation. Here's how you can convert a list of integers to a space-separated string:
[[See Video to Reveal this Text or Code Snippet]]
In this example, the Select method is used to convert each integer in the list to a string, and the string.Join method concatenates them with a space in between.
Converting a List of Integers to a Comma-Separated String
Another common requirement is to convert a list of integers into a comma-separated string. LINQ makes this task straightforward:
[[See Video to Reveal this Text or Code Snippet]]
Just like the previous example, the Select method converts each integer to a string, and string.Join creates the comma-separated result.
Advanced Use Case: Conditional Formatting
Suppose you want to format the numbers conditionally. For instance, you might want to surround negative numbers with parentheses:
[[See Video to Reveal this Text or Code Snippet]]
This example uses a ternary operator within the Select method to apply conditional formatting based on the value of each integer.
Conclusion
Using LINQ for converting integers and lists of integers to strings in C allows for clean, readable code. Whether you're working with single integers, lists, or applying conditional formatting, LINQ offers a versatile and elegant approach to these common tasks.
LINQ's rich feature set ensures that you can handle a wide range of data manipulation tasks efficiently, making it an essential tool in the C programmer's toolkit.