filmov
tv
Mastering LINQ: Select to New Object and Object List
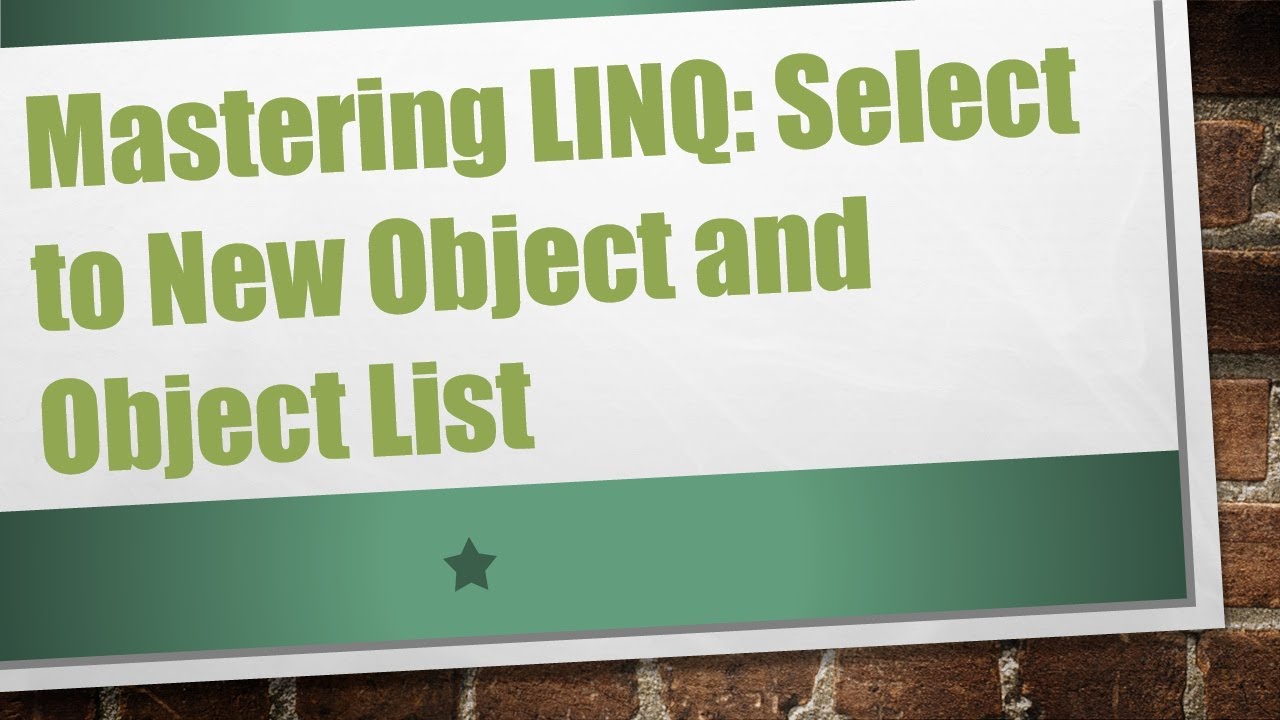
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Learn how to use LINQ to create new objects and object lists, making your data manipulation more efficient and readable. Dive into practical examples and improve your skills.
---
Mastering LINQ: Select to New Object and Object List
LINQ (Language-Integrated Query) has become a fundamental aspect of data manipulation in .NET. It provides a more streamlined way to query, sort, filter, and project data. One of the powerful features of LINQ is its ability to create new objects and object lists in a succinct and readable manner. This guide will explore how to use LINQ to create new objects and object lists, with practical examples to help you harness its capabilities effectively.
Using LINQ to Select a New Object
In many scenarios, working with data means transforming it into different forms. LINQ's Select method allows you to project each element of a collection into a new form, such as a new object. Here's an example to illustrate this.
Example
Suppose you have a list of Person objects and you want to create a new list containing only the names and ages:
[[See Video to Reveal this Text or Code Snippet]]
In this example, people.Select(p => new { p.Name, p.Age }).ToList(); creates an anonymous type containing only the Name and Age properties. The result is a list of these new objects.
Explanation
Select Method: Projects each element of the people collection into a new form (anonymous type).
Anonymous Type: A type created on the fly that contains only the specified properties.
ToList Method: Converts the result of Select into a list.
Using LINQ to Select to a New Object List
In some cases, you might want to convert your data into a known type instead of an anonymous type. This can be achieved by defining a custom class and selecting into this class.
Example
Let's extend the previous example by creating a dedicated PersonInfo class:
[[See Video to Reveal this Text or Code Snippet]]
Explanation
Custom Class: PersonInfo is a class defined to hold the desired properties.
Select with Custom Class: Projects each element of people into a new PersonInfo object.
ToList Method: Converts the result into a list of PersonInfo objects.
Conclusion
Understanding how to use LINQ to create new objects and object lists is a valuable skill for any .NET developer. It allows you to transform and project data into meaningful forms, enhancing the readability and maintainability of your code. By mastering these techniques, you too can make your data manipulation tasks more efficient and elegant.
Happy coding!
---
Summary: Learn how to use LINQ to create new objects and object lists, making your data manipulation more efficient and readable. Dive into practical examples and improve your skills.
---
Mastering LINQ: Select to New Object and Object List
LINQ (Language-Integrated Query) has become a fundamental aspect of data manipulation in .NET. It provides a more streamlined way to query, sort, filter, and project data. One of the powerful features of LINQ is its ability to create new objects and object lists in a succinct and readable manner. This guide will explore how to use LINQ to create new objects and object lists, with practical examples to help you harness its capabilities effectively.
Using LINQ to Select a New Object
In many scenarios, working with data means transforming it into different forms. LINQ's Select method allows you to project each element of a collection into a new form, such as a new object. Here's an example to illustrate this.
Example
Suppose you have a list of Person objects and you want to create a new list containing only the names and ages:
[[See Video to Reveal this Text or Code Snippet]]
In this example, people.Select(p => new { p.Name, p.Age }).ToList(); creates an anonymous type containing only the Name and Age properties. The result is a list of these new objects.
Explanation
Select Method: Projects each element of the people collection into a new form (anonymous type).
Anonymous Type: A type created on the fly that contains only the specified properties.
ToList Method: Converts the result of Select into a list.
Using LINQ to Select to a New Object List
In some cases, you might want to convert your data into a known type instead of an anonymous type. This can be achieved by defining a custom class and selecting into this class.
Example
Let's extend the previous example by creating a dedicated PersonInfo class:
[[See Video to Reveal this Text or Code Snippet]]
Explanation
Custom Class: PersonInfo is a class defined to hold the desired properties.
Select with Custom Class: Projects each element of people into a new PersonInfo object.
ToList Method: Converts the result into a list of PersonInfo objects.
Conclusion
Understanding how to use LINQ to create new objects and object lists is a valuable skill for any .NET developer. It allows you to transform and project data into meaningful forms, enhancing the readability and maintainability of your code. By mastering these techniques, you too can make your data manipulation tasks more efficient and elegant.
Happy coding!