filmov
tv
Counting Production Cycles in Pandas
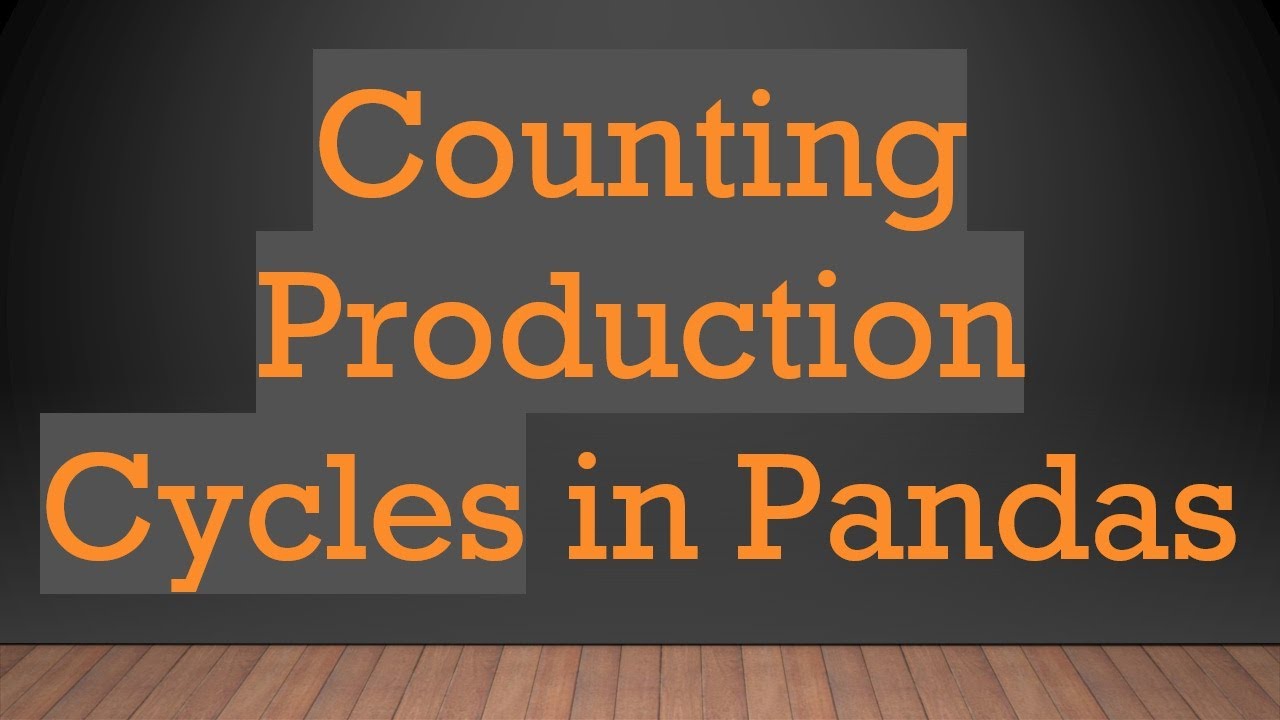
Показать описание
Learn how to iteratively count instances of a category in a Pandas DataFrame and reset counts when encountering a different category.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: pandas how to iteratively count instances of a category by row and reset them when the other category appears?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Counting Production Cycles in Pandas: A Step-by-Step Guide
As data analysts, we often deal with time series data that involves different states. One common scenario arises when we need to track how long a machine operates under specific conditions. In this guide, we'll tackle the problem of counting production cycles for a machine that switches between two states: Production and Cleaning.
The Problem at Hand
Imagine you have a DataFrame that tracks the behavior of a machine. This machine operates in two distinct states, indicated by a dummy variable called Production:
1 indicates that the machine is producing.
0 signifies that the machine is in the cleaning mode.
You want to create a new column that counts how many hours the machine remains in each state, resetting the count whenever the state changes. Here’s how your output should look, for instance:
[[See Video to Reveal this Text or Code Snippet]]
The Solution Explained
To achieve this in Pandas, we will follow a few straightforward steps that utilize a combination of functions to detect the state changes and group the data accordingly.
Step 1: Detect State Changes
First, we will identify where the state changes occur. This can be done using the diff() function, which computes the difference between current and previous rows. The places where this difference is not equal to zero indicate state changes.
Step 2: Cumulative Sum for Grouping
Next, we perform a cumulative sum (cumsum()) of the changes detected. This helps in grouping the rows based on consecutive states.
Step 3: Group by State and Count Rows
Finally, we can group the DataFrame by the newly created group and use transform("count") to count the number of consecutive occurrences for each state.
Implementation Example
Here’s how to implement these steps in code:
[[See Video to Reveal this Text or Code Snippet]]
The Result
When you run the code above, you will receive an output DataFrame that looks like this:
[[See Video to Reveal this Text or Code Snippet]]
Verification of Grouping
To confirm that the grouping is correct, you can print the grouper, which should display the group assignments for each row:
[[See Video to Reveal this Text or Code Snippet]]
The output will help verify how your DataFrame was categorized at each step:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Counting instances of a category in Pandas while resetting those counts upon state changes may seem complex, but by following systematic steps, you can effortlessly manage and analyze such data. Using techniques like diff(), cumsum(), and groupby(), you can gain valuable insights into your machine's production and cleaning cycles.
Now that you have a clear understanding of how to perform this operation in Pandas, you can apply these techniques to your own datasets and unlock deeper insights into machine behaviors and operations.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: pandas how to iteratively count instances of a category by row and reset them when the other category appears?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Counting Production Cycles in Pandas: A Step-by-Step Guide
As data analysts, we often deal with time series data that involves different states. One common scenario arises when we need to track how long a machine operates under specific conditions. In this guide, we'll tackle the problem of counting production cycles for a machine that switches between two states: Production and Cleaning.
The Problem at Hand
Imagine you have a DataFrame that tracks the behavior of a machine. This machine operates in two distinct states, indicated by a dummy variable called Production:
1 indicates that the machine is producing.
0 signifies that the machine is in the cleaning mode.
You want to create a new column that counts how many hours the machine remains in each state, resetting the count whenever the state changes. Here’s how your output should look, for instance:
[[See Video to Reveal this Text or Code Snippet]]
The Solution Explained
To achieve this in Pandas, we will follow a few straightforward steps that utilize a combination of functions to detect the state changes and group the data accordingly.
Step 1: Detect State Changes
First, we will identify where the state changes occur. This can be done using the diff() function, which computes the difference between current and previous rows. The places where this difference is not equal to zero indicate state changes.
Step 2: Cumulative Sum for Grouping
Next, we perform a cumulative sum (cumsum()) of the changes detected. This helps in grouping the rows based on consecutive states.
Step 3: Group by State and Count Rows
Finally, we can group the DataFrame by the newly created group and use transform("count") to count the number of consecutive occurrences for each state.
Implementation Example
Here’s how to implement these steps in code:
[[See Video to Reveal this Text or Code Snippet]]
The Result
When you run the code above, you will receive an output DataFrame that looks like this:
[[See Video to Reveal this Text or Code Snippet]]
Verification of Grouping
To confirm that the grouping is correct, you can print the grouper, which should display the group assignments for each row:
[[See Video to Reveal this Text or Code Snippet]]
The output will help verify how your DataFrame was categorized at each step:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Counting instances of a category in Pandas while resetting those counts upon state changes may seem complex, but by following systematic steps, you can effortlessly manage and analyze such data. Using techniques like diff(), cumsum(), and groupby(), you can gain valuable insights into your machine's production and cleaning cycles.
Now that you have a clear understanding of how to perform this operation in Pandas, you can apply these techniques to your own datasets and unlock deeper insights into machine behaviors and operations.