filmov
tv
Creating Multiple Excel Sheets Using Python Pandas
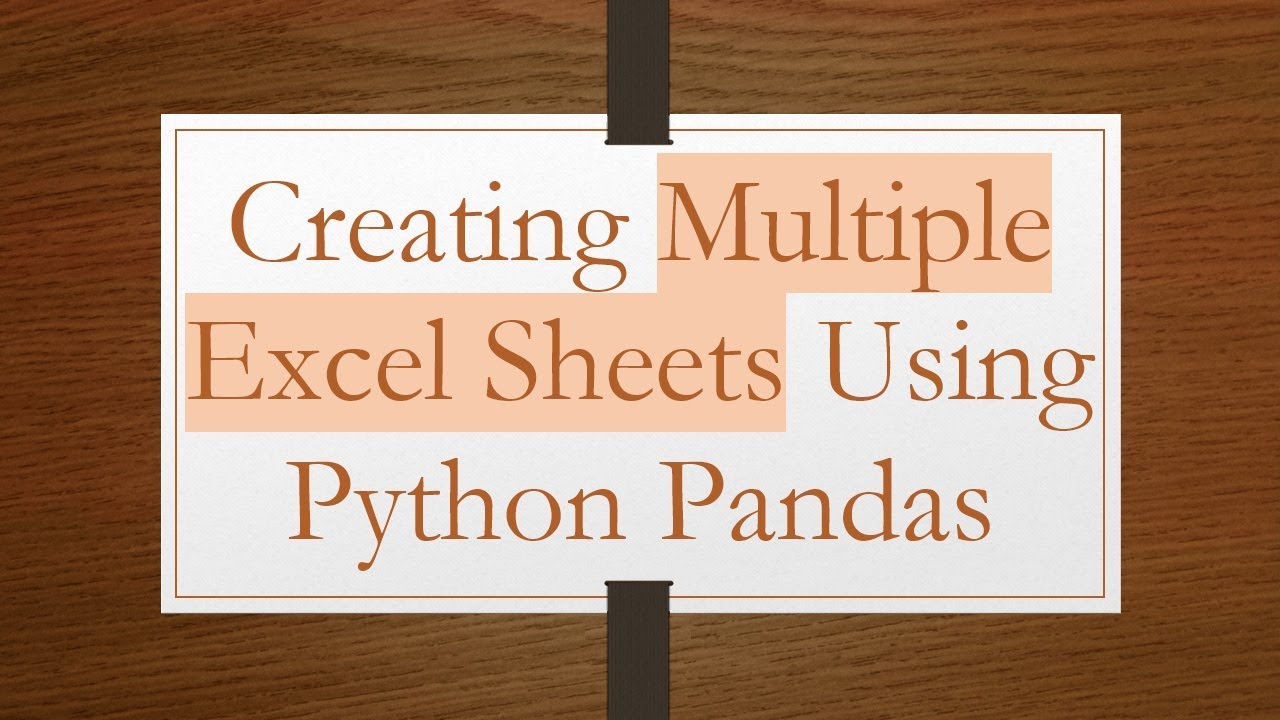
Показать описание
Learn how to save `multiple sheets` in an Excel file using Python Pandas without losing existing data.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Creating Multiple Excel sheets using data frames Python pandas
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Creating Multiple Excel Sheets Using Python Pandas
When working with data frames in Python, specifically with the Pandas library, you might encounter a common issue—saving multiple sheets in a single Excel file. It's a frustrating situation when you think you've successfully stored your data, only to find that the new sheet has replaced the previous one! This guide aims to provide a clear solution to this problem by guiding you through the steps of creating multiple Excel sheets efficiently.
The Problem Explained
In your initial attempt to create multiple sheets in an Excel file, you might have used the to_excel() method like this:
[[See Video to Reveal this Text or Code Snippet]]
The Solution: Using ExcelWriter
To successfully save multiple sheets in an Excel file without overwriting existing data, you need to utilize Pandas' ExcelWriter method. This allows you to create an Excel file and write multiple data frames to separate sheets without any data loss. Let's walk through how to implement this.
Step-by-Step Instructions
Import Required Libraries
Make sure to import the necessary libraries:
[[See Video to Reveal this Text or Code Snippet]]
Read Your Data Files
Load your CSV data into data frames. For example:
[[See Video to Reveal this Text or Code Snippet]]
Create Multiple Sheets
You can create multiple sheets by using the ExcelWriter context manager. Here's how:
[[See Video to Reveal this Text or Code Snippet]]
OR if you prefer to specify the engine explicitly:
[[See Video to Reveal this Text or Code Snippet]]
Benefits of Using ExcelWriter
Avoid Data Loss: By opening an Excel writer context, you avoid overwriting previous sheets.
Organization: Each data frame can be neatly organized into its respective sheet within the same Excel file.
Compatibility: ExcelWriter is compatible with various file formats and provides the flexibility of different engines.
Conclusion
By following this guide, you can efficiently create multiple sheets in an Excel workbook using Python Pandas. Avoiding the initial pitfall of overwriting is essential for maintaining data integrity, and using methods like ExcelWriter allows you to manage your datasets correctly.
Whether you're analyzing data from multiple sources or handling large datasets, knowing how to operate with Excel sheets can significantly enhance your productivity.
So, go ahead and try building your Excel sheets with confidence! If you have any further questions or issues, feel free to leave a comment below.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Creating Multiple Excel sheets using data frames Python pandas
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Creating Multiple Excel Sheets Using Python Pandas
When working with data frames in Python, specifically with the Pandas library, you might encounter a common issue—saving multiple sheets in a single Excel file. It's a frustrating situation when you think you've successfully stored your data, only to find that the new sheet has replaced the previous one! This guide aims to provide a clear solution to this problem by guiding you through the steps of creating multiple Excel sheets efficiently.
The Problem Explained
In your initial attempt to create multiple sheets in an Excel file, you might have used the to_excel() method like this:
[[See Video to Reveal this Text or Code Snippet]]
The Solution: Using ExcelWriter
To successfully save multiple sheets in an Excel file without overwriting existing data, you need to utilize Pandas' ExcelWriter method. This allows you to create an Excel file and write multiple data frames to separate sheets without any data loss. Let's walk through how to implement this.
Step-by-Step Instructions
Import Required Libraries
Make sure to import the necessary libraries:
[[See Video to Reveal this Text or Code Snippet]]
Read Your Data Files
Load your CSV data into data frames. For example:
[[See Video to Reveal this Text or Code Snippet]]
Create Multiple Sheets
You can create multiple sheets by using the ExcelWriter context manager. Here's how:
[[See Video to Reveal this Text or Code Snippet]]
OR if you prefer to specify the engine explicitly:
[[See Video to Reveal this Text or Code Snippet]]
Benefits of Using ExcelWriter
Avoid Data Loss: By opening an Excel writer context, you avoid overwriting previous sheets.
Organization: Each data frame can be neatly organized into its respective sheet within the same Excel file.
Compatibility: ExcelWriter is compatible with various file formats and provides the flexibility of different engines.
Conclusion
By following this guide, you can efficiently create multiple sheets in an Excel workbook using Python Pandas. Avoiding the initial pitfall of overwriting is essential for maintaining data integrity, and using methods like ExcelWriter allows you to manage your datasets correctly.
Whether you're analyzing data from multiple sources or handling large datasets, knowing how to operate with Excel sheets can significantly enhance your productivity.
So, go ahead and try building your Excel sheets with confidence! If you have any further questions or issues, feel free to leave a comment below.