filmov
tv
The Box Smart Pointer in Rust
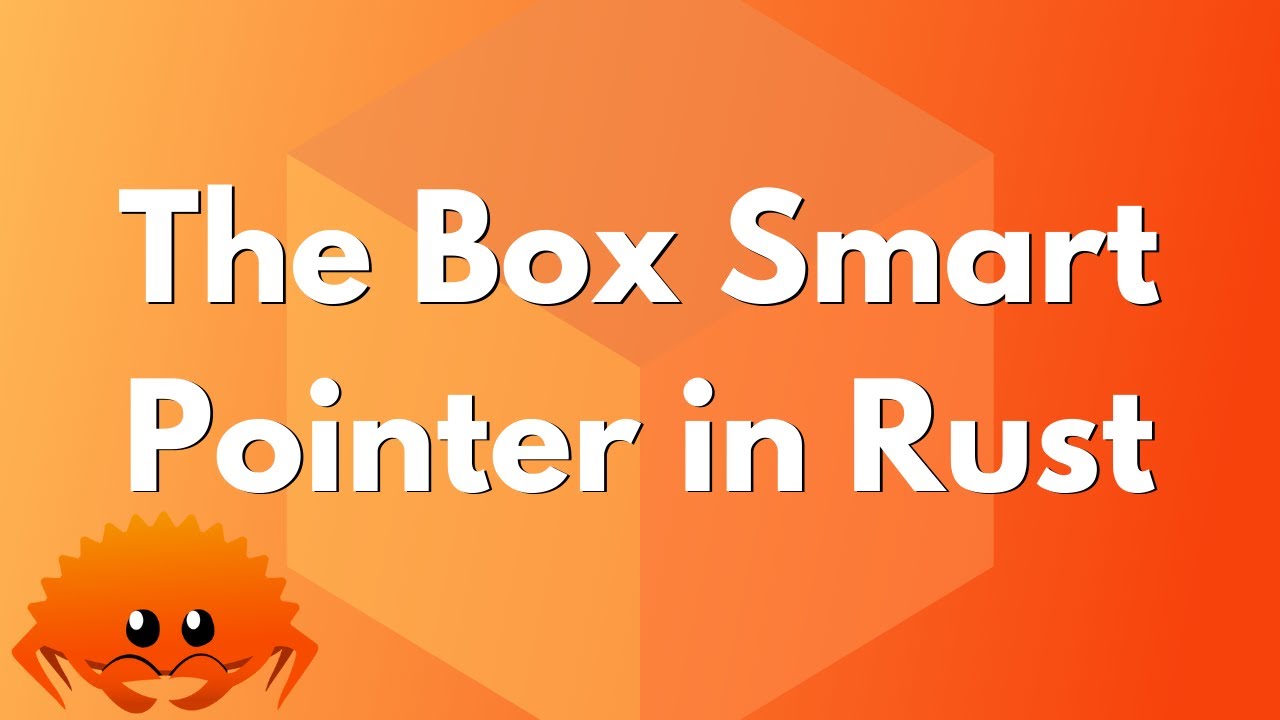
Показать описание
The ultimate Rust lang tutorial. Follow along as we go through the Rust lang book chapter by chapter.
Chapters:
0:00 Intro
0:29 Pointers, References, and Smart Pointers
2:38 Using a Box to Store Data
4:43 Enabling Recursive Types with Boxes
11:53 Outro
#letsgetrusty #rustlang #tutorial
Chapters:
0:00 Intro
0:29 Pointers, References, and Smart Pointers
2:38 Using a Box to Store Data
4:43 Enabling Recursive Types with Boxes
11:53 Outro
#letsgetrusty #rustlang #tutorial
The Box Smart Pointer in Rust
Rust's Alien Data Types 👽 Box, Rc, Arc
Smart Pointers & Box - Rust
Rust: Smart Pointers (Box)
Rust For Beginners Tutorial - Box Smart Pointers
Box / Rc / Arc / Mutex - Smart Pointers Simplified - Rust
Crust of Rust: Smart Pointers and Interior Mutability
Using The Box Smart pointer to Point to Data on the Heap - Full Crash Rust Tutorial for Beginners
Rust: Store Data on the Heap with Box
8. smart pointers Box in rust
Smart Pointers in Rust - The Deref Trait
Learn Rust Together Part 20: Chapter 15 (Smart Pointers)
Smart Pointers - data structures that act like a pointer - Full Crash Rust Tutorial for Beginners
Smart Pointers in Rust - Reference Counting
Generics & Smart Pointers in Rust - CS 128 Honors Lecture 10
Back to Basics: C++ Smart Pointers - David Olsen - CppCon 2022
Rust Smart Pointers - Box, Rc, Ref & RefMut (15)
Generics and Smart Pointers in Rust - CS 128 Honors Lecture 10
Ep15 Rust Language for Beginners Smart Pointers
Rust Lang Book Ch 15::01 — Smart Pointers: Boxes
10. Smart Pointers - From Python to Rust
Smart Pointers in Rust - Interior Mutability
LG Magic Remote: How to Turn Pointer ON & OFF
Rust Boxes: Efficient Memory Management and Ownership Transfer
Комментарии