filmov
tv
Understanding Static Variables in PHP
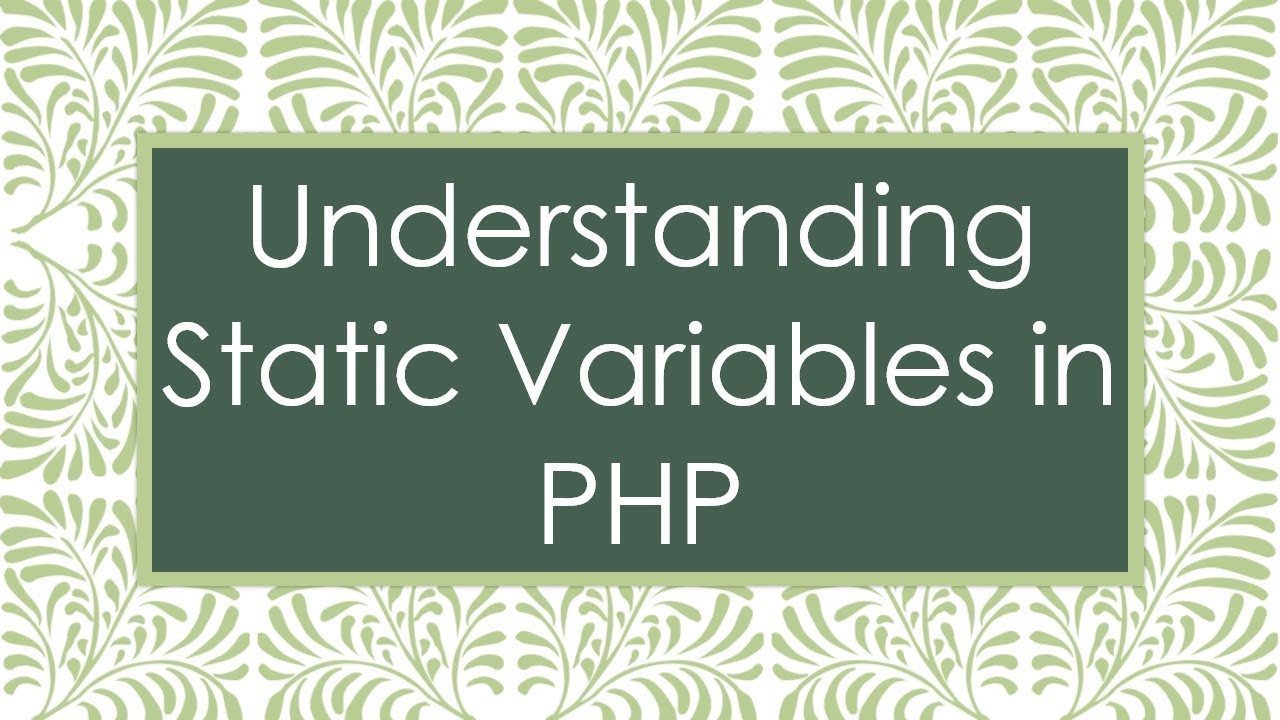
Показать описание
Learn about the function and benefits of using static variables in PHP, how they differ from other types of variables, and when to use them effectively in your code.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Understanding Static Variables in PHP: A Comprehensive Guide
In PHP, the keyword static holds particular significance when it comes to managing the lifecycle of variables within functions or methods. Static variables in PHP are a powerful feature that can significantly influence how data is managed and maintained across function calls. This guide aims to demystify what static variables are, how they work, and when to use them in your codebase.
What Are Static Variables?
A static variable in PHP is a variable that retains its value between multiple function or method calls. Unlike a regular local variable, a static variable does not lose its value when the function exits. Instead, it preserves its state and resumes with the same value when the function is called again.
Syntax
Here is a simple example to illustrate the concept of a static variable:
[[See Video to Reveal this Text or Code Snippet]]
In this example, the $counter variable is declared as static within the incrementCounter function. Each time the function is invoked, $counter is incremented by one, and it retains this state across calls.
When to Use Static Variables
Static variables are particularly useful in scenarios where you need to maintain a state between multiple function calls without resorting to global variables or other forms of state management. Below are some common use cases:
Counting Function Calls: As shown in the example above, static variables can be used to count the number of times a function is called.
Caching: If you need to store some computed values for subsequent calls without re-calculating, static variables can be useful.
Singleton Pattern: In Object-Oriented Programming, static variables are often used to implement the Singleton design pattern.
Practical Example: Singleton Pattern
In OOP, the Singleton pattern ensures a class has only one instance and provides a global point of access to it. Here's a simple implementation:
[[See Video to Reveal this Text or Code Snippet]]
In this example, the static variable $instance holds the Singleton instance. The getInstance method checks whether an instance already exists and creates one if it does not. This ensures that Singleton has only one instance throughout.
Benefits and Limitations
Using static variables responsibly can bring several benefits:
Performance: Static variables can improve performance by avoiding unnecessary computations.
State Retention: They preserve state information across multiple calls, which can be very useful for caching and maintaining counters.
However, static variables also have some limitations:
Limited Scope: Static variables are visible only within the function or method they are declared in.
Potential Misuse: Overusing static variables may lead to code that is harder to understand and maintain.
Conclusion
Static variables in PHP provide a straightforward and efficient way to retain state within a function or method scope. They are ideal for scenarios where state needs to persist across function calls, offering a neat solution without cluttering the global scope. However, like any feature, they should be used judiciously to avoid potential pitfalls.
By understanding how and when to utilize static variables, PHP developers can write more efficient and maintainable code.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Understanding Static Variables in PHP: A Comprehensive Guide
In PHP, the keyword static holds particular significance when it comes to managing the lifecycle of variables within functions or methods. Static variables in PHP are a powerful feature that can significantly influence how data is managed and maintained across function calls. This guide aims to demystify what static variables are, how they work, and when to use them in your codebase.
What Are Static Variables?
A static variable in PHP is a variable that retains its value between multiple function or method calls. Unlike a regular local variable, a static variable does not lose its value when the function exits. Instead, it preserves its state and resumes with the same value when the function is called again.
Syntax
Here is a simple example to illustrate the concept of a static variable:
[[See Video to Reveal this Text or Code Snippet]]
In this example, the $counter variable is declared as static within the incrementCounter function. Each time the function is invoked, $counter is incremented by one, and it retains this state across calls.
When to Use Static Variables
Static variables are particularly useful in scenarios where you need to maintain a state between multiple function calls without resorting to global variables or other forms of state management. Below are some common use cases:
Counting Function Calls: As shown in the example above, static variables can be used to count the number of times a function is called.
Caching: If you need to store some computed values for subsequent calls without re-calculating, static variables can be useful.
Singleton Pattern: In Object-Oriented Programming, static variables are often used to implement the Singleton design pattern.
Practical Example: Singleton Pattern
In OOP, the Singleton pattern ensures a class has only one instance and provides a global point of access to it. Here's a simple implementation:
[[See Video to Reveal this Text or Code Snippet]]
In this example, the static variable $instance holds the Singleton instance. The getInstance method checks whether an instance already exists and creates one if it does not. This ensures that Singleton has only one instance throughout.
Benefits and Limitations
Using static variables responsibly can bring several benefits:
Performance: Static variables can improve performance by avoiding unnecessary computations.
State Retention: They preserve state information across multiple calls, which can be very useful for caching and maintaining counters.
However, static variables also have some limitations:
Limited Scope: Static variables are visible only within the function or method they are declared in.
Potential Misuse: Overusing static variables may lead to code that is harder to understand and maintain.
Conclusion
Static variables in PHP provide a straightforward and efficient way to retain state within a function or method scope. They are ideal for scenarios where state needs to persist across function calls, offering a neat solution without cluttering the global scope. However, like any feature, they should be used judiciously to avoid potential pitfalls.
By understanding how and when to utilize static variables, PHP developers can write more efficient and maintainable code.