filmov
tv
Understanding PHP Static Variables: Usage Inside Functions and Classes
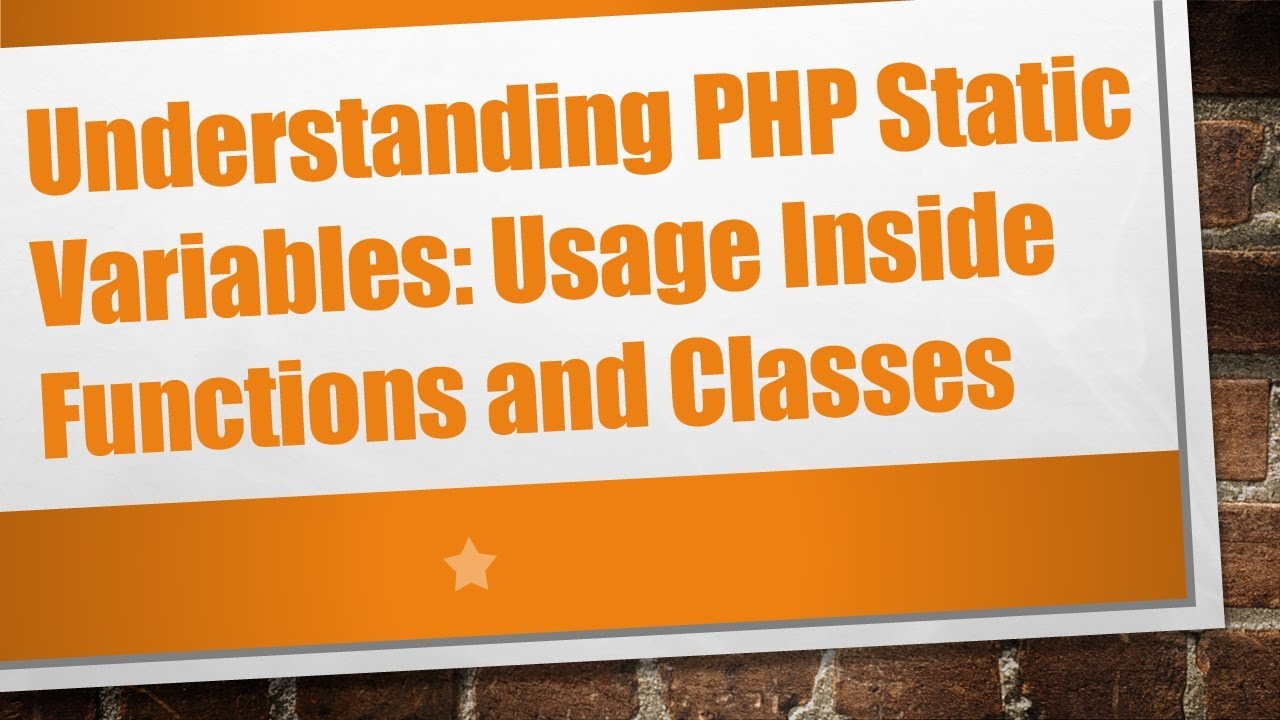
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Learn about `PHP static variables`, their use inside functions and classes, and get an example to illustrate their functionality.
---
Understanding PHP Static Variables: Usage Inside Functions and Classes
In PHP, static variables are an important concept that allows for the preservation of a variable's state between function or method calls. This guide delves into PHP static variables, their application in functions and classes, and provides an illustrative example to enhance understanding.
What are PHP Static Variables?
A static variable in PHP is a variable that retains its value even after the execution of the block of code in which it is defined. This means that the variable is initialized only once and is not re-initialized each time the code block runs.
PHP Static Variables in Functions
Static variables can be particularly useful in functions where you need to keep track of state information across multiple calls to the function. Here is a quick example:
[[See Video to Reveal this Text or Code Snippet]]
In this example, the static variable $count starts at 0 and increments by 1 with each function call. Because it's declared as static, it retains its value between calls to exampleFunction.
PHP Static Variables Inside Classes
Static variables can also be applied to class properties. These variables are shared among all instances of the class, meaning if one instance modifies a static variable, the change will be reflected across all other instances.
Static Methods and Properties
To declare a static class property, use the static keyword. Similarly, static methods are accessed using the self keyword within the class.
[[See Video to Reveal this Text or Code Snippet]]
In the code above, self::$staticVariable is a static property, and incrementStaticVariable is a static method. When the static method is called, it increments the static property, demonstrating how static variables persist across different instances and calls.
Practical Applications
Static variables are useful in scenarios where you need to maintain a persistent state:
Counting Function Calls: As shown earlier, static variables can be used to keep track of the number of times a function has been called.
Singletons: In object-oriented programming, static properties can be used to enforce the Singleton pattern, ensuring that a class has only one instance.
Caching: Static variables can be used for simple caching mechanisms within functions.
Conclusion
Understanding how to use PHP static variables effectively can significantly enhance the efficiency and reliability of your code. Whether they are employed in functions to retain state between calls or within classes to share data among instances, static variables offer powerful capabilities for developers.
By grasping the basics and diving into practical examples, you can leverage static variables to create more sophisticated and maintainable PHP applications. We hope this post has provided you with the knowledge to use PHP static variables effectively in your projects.
---
Summary: Learn about `PHP static variables`, their use inside functions and classes, and get an example to illustrate their functionality.
---
Understanding PHP Static Variables: Usage Inside Functions and Classes
In PHP, static variables are an important concept that allows for the preservation of a variable's state between function or method calls. This guide delves into PHP static variables, their application in functions and classes, and provides an illustrative example to enhance understanding.
What are PHP Static Variables?
A static variable in PHP is a variable that retains its value even after the execution of the block of code in which it is defined. This means that the variable is initialized only once and is not re-initialized each time the code block runs.
PHP Static Variables in Functions
Static variables can be particularly useful in functions where you need to keep track of state information across multiple calls to the function. Here is a quick example:
[[See Video to Reveal this Text or Code Snippet]]
In this example, the static variable $count starts at 0 and increments by 1 with each function call. Because it's declared as static, it retains its value between calls to exampleFunction.
PHP Static Variables Inside Classes
Static variables can also be applied to class properties. These variables are shared among all instances of the class, meaning if one instance modifies a static variable, the change will be reflected across all other instances.
Static Methods and Properties
To declare a static class property, use the static keyword. Similarly, static methods are accessed using the self keyword within the class.
[[See Video to Reveal this Text or Code Snippet]]
In the code above, self::$staticVariable is a static property, and incrementStaticVariable is a static method. When the static method is called, it increments the static property, demonstrating how static variables persist across different instances and calls.
Practical Applications
Static variables are useful in scenarios where you need to maintain a persistent state:
Counting Function Calls: As shown earlier, static variables can be used to keep track of the number of times a function has been called.
Singletons: In object-oriented programming, static properties can be used to enforce the Singleton pattern, ensuring that a class has only one instance.
Caching: Static variables can be used for simple caching mechanisms within functions.
Conclusion
Understanding how to use PHP static variables effectively can significantly enhance the efficiency and reliability of your code. Whether they are employed in functions to retain state between calls or within classes to share data among instances, static variables offer powerful capabilities for developers.
By grasping the basics and diving into practical examples, you can leverage static variables to create more sophisticated and maintainable PHP applications. We hope this post has provided you with the knowledge to use PHP static variables effectively in your projects.