filmov
tv
The Most Basic Pathfinding Algorithm, Explained
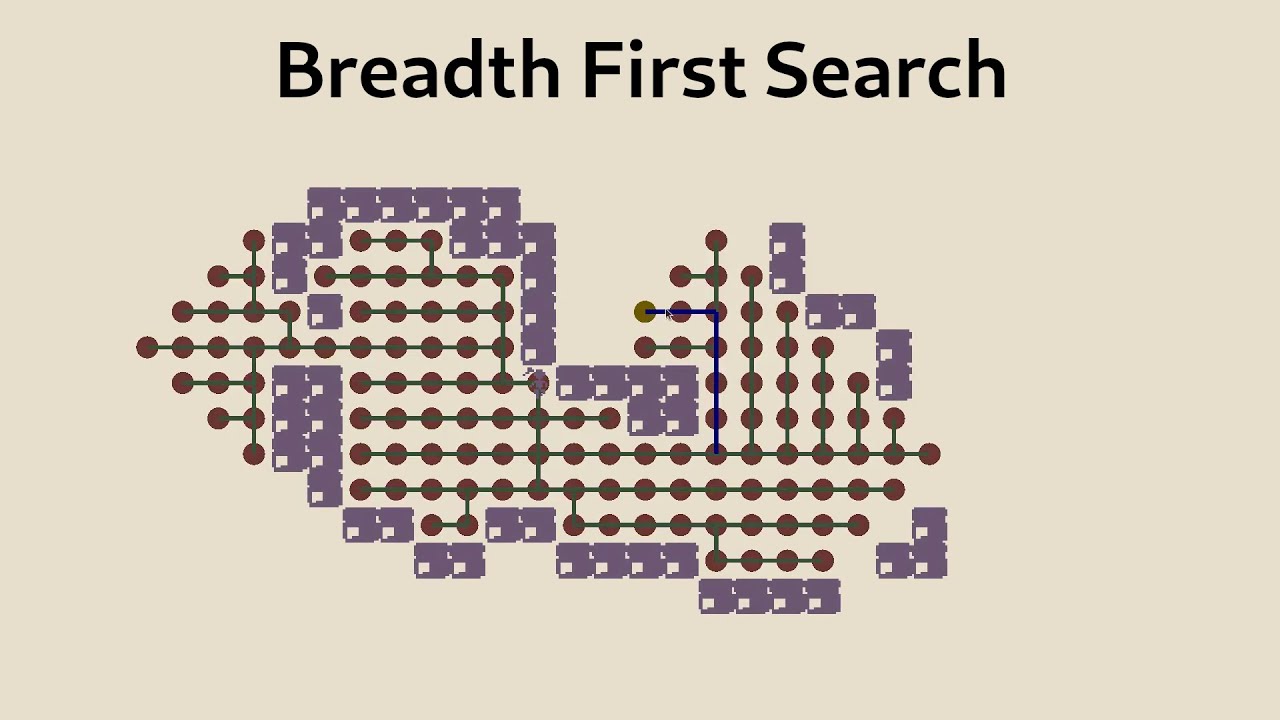
Показать описание
- Support Me -
- Other Stuff -
The Most Basic Pathfinding Algorithm, Explained
A* Pathfinding (E01: algorithm explanation)
Visualizing Pathfinding Algorithms
How To Find Shortest Paths #computerscience #algorithms
A Comparison of Pathfinding Algorithms
A* Shortest Path Algorithm Visually Explained
Path Finding Algorithm Basics: The A* Algorithm in 10 Min
Pathfinding Algorithm | Machine Learning | Artificial Intelligence #ai
ANATOLIAN CROSSROADS: Dijkstra and A* Finding Paths Through Ankara's Modern Capital | 4K UHD
A* (A Star) Search Algorithm - Computerphile
Best-First Search: AI's Smart Pathfinding Algorithm Explained! 🌍
Sigma Maze Solved with Dijkstra's Algorithm
What is an Algorithm ? | Most Asked Interview Q&A
Randomized Kruskal's Maze Solved with A* Algorithm (Euclidean Distance)
Factorio New Pathfinding System
Visualizing Maze Solving with Depth-First Search (DFS) Algorithm
How to Do PATHFINDING: A* Algorithm (The Thing That Most Games Actually Use)
Randomized Kruskal's Maze Solved with A* Algorithm (Manhattan Distance)
The secret behind pathfinding in video games | A* Algorithm | Dijkstra’s algorithm #shorts
Solving mazes using a Pathfinding Algorithm -- visualized
Ants = Pathfinding Algorithm
Breadth First Search (BFS) Algorithm Explained + Python Implementation
Mastering Pathfinding Algorithms: From Basics to Implementation
How dijkstra algorithms work 🚀🚀 || dijkstra algorithm in daa || #shorts #algorithm #dsa #programming...
Комментарии