filmov
tv
Solving jQuery Click Event Issues for Dynamically Created Buttons in Loops
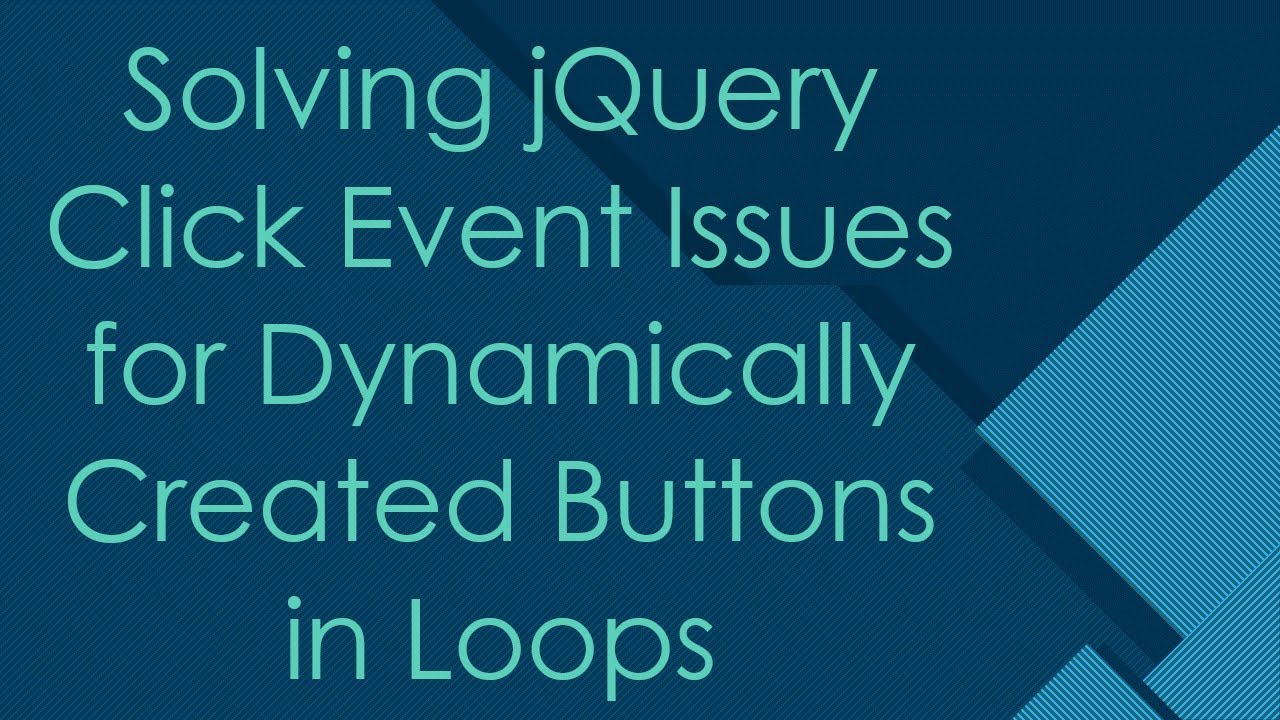
Показать описание
Learn how to effectively manage click events for buttons generated within a loop using jQuery. Discover best practices to simplify your code and enhance performance.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: jQuery - Call click event on dinamiclly created button in loop
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Solving jQuery Click Event Issues for Dynamically Created Buttons in Loops
When working with dynamic content generation in JavaScript, especially when combined with jQuery, developers often encounter challenges related to event handling. A common scenario arises when attempting to attach click events to buttons that are created within a loop after an AJAX request. This guide will address the specific issue of triggering click events on dynamically generated buttons and provide a structured solution to streamline your code.
The Problem
Imagine a scenario where you're pulling data from a server and populating an HTML table with product information. Each row of this table has a button to delete the corresponding product. However, when you attempt to attach a click event to these buttons within the loop, you may notice that the click event does not fire as expected. This can be frustrating and might leave you wondering what went wrong.
The key issue arises because the buttons are generated dynamically at runtime, and using id attributes for these elements adds complexity without real benefit. Let's dive deeper into how to solve this problem.
Solution Overview: Use Delegated Event Handling
Instead of trying to attach click events directly inside the loop using ids, a more effective approach is to use a common class for the buttons. Furthermore, leveraging jQuery's delegated event handling allows you to manage events on elements that may not exist at the time of binding. Here’s how to implement this solution step by step.
Step 1: Generate HTML with Common Class
First, modify your AJAX success callback to apply a common class (e.g., btn-delete) to your buttons instead of using unique ids. You can use data- attributes to store specific information, such as the product ID.
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Implement Delegated Event Handler
Next, set up a delegated event listener on the table body. This allows you to capture clicks on any .btn-delete button, regardless of when it was created.
[[See Video to Reveal this Text or Code Snippet]]
Considerations for Performance
While the solution above solves the click event issue, it's also important to consider performance implications. Generating HTML in this way can be optimized further. For better performance:
Use a <template> element: This keeps your HTML clean and allows you to clone a template for each row, reducing DOM manipulation overhead.
Build a single HTML string: Instead of creating multiple jQuery objects within the loop, build your HTML structure as a string and append it to the DOM once, which is usually faster.
Conclusion
By applying delegated event handling and using common classes rather than relying on unique IDs, you can effectively manage click events for dynamically generated buttons. This not only helps to resolve the specific problem you might be facing but also promotes cleaner and more maintainable code. Consider further optimizations, such as using templates and building a single HTML string for the best performance. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: jQuery - Call click event on dinamiclly created button in loop
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Solving jQuery Click Event Issues for Dynamically Created Buttons in Loops
When working with dynamic content generation in JavaScript, especially when combined with jQuery, developers often encounter challenges related to event handling. A common scenario arises when attempting to attach click events to buttons that are created within a loop after an AJAX request. This guide will address the specific issue of triggering click events on dynamically generated buttons and provide a structured solution to streamline your code.
The Problem
Imagine a scenario where you're pulling data from a server and populating an HTML table with product information. Each row of this table has a button to delete the corresponding product. However, when you attempt to attach a click event to these buttons within the loop, you may notice that the click event does not fire as expected. This can be frustrating and might leave you wondering what went wrong.
The key issue arises because the buttons are generated dynamically at runtime, and using id attributes for these elements adds complexity without real benefit. Let's dive deeper into how to solve this problem.
Solution Overview: Use Delegated Event Handling
Instead of trying to attach click events directly inside the loop using ids, a more effective approach is to use a common class for the buttons. Furthermore, leveraging jQuery's delegated event handling allows you to manage events on elements that may not exist at the time of binding. Here’s how to implement this solution step by step.
Step 1: Generate HTML with Common Class
First, modify your AJAX success callback to apply a common class (e.g., btn-delete) to your buttons instead of using unique ids. You can use data- attributes to store specific information, such as the product ID.
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Implement Delegated Event Handler
Next, set up a delegated event listener on the table body. This allows you to capture clicks on any .btn-delete button, regardless of when it was created.
[[See Video to Reveal this Text or Code Snippet]]
Considerations for Performance
While the solution above solves the click event issue, it's also important to consider performance implications. Generating HTML in this way can be optimized further. For better performance:
Use a <template> element: This keeps your HTML clean and allows you to clone a template for each row, reducing DOM manipulation overhead.
Build a single HTML string: Instead of creating multiple jQuery objects within the loop, build your HTML structure as a string and append it to the DOM once, which is usually faster.
Conclusion
By applying delegated event handling and using common classes rather than relying on unique IDs, you can effectively manage click events for dynamically generated buttons. This not only helps to resolve the specific problem you might be facing but also promotes cleaner and more maintainable code. Consider further optimizations, such as using templates and building a single HTML string for the best performance. Happy coding!