filmov
tv
Solving the Cannot access variable inside event issue in jQuery
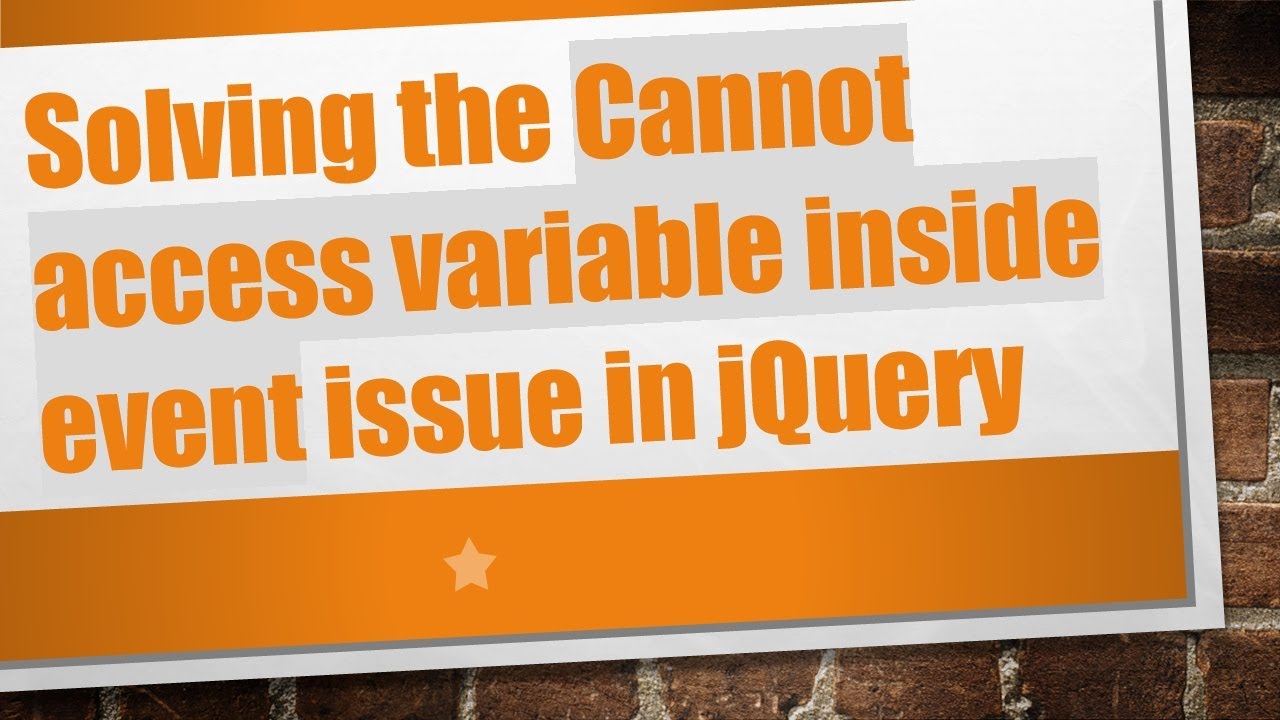
Показать описание
Discover how to resolve the `Cannot access variable inside event` problem in jQuery with simple steps. Enhance your JavaScript skills!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Cannot access variable inside event
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Fixing the "Cannot Access Variable Inside Event" Issue in jQuery
If you're working with jQuery to handle form submissions or button clicks, you may have encountered an annoying problem: trying to access a variable inside an event function, only to find it returning an empty string. Let's explore why this happens and how to fix it effectively.
Understanding the Problem
Imagine you've set up a form with fields for a username, email, and password. When you try to retrieve the value of the username variable in a click event, you get an empty response. You might be puzzled, especially when you see that the variable is set correctly outside the event function. This confusion is common among developers working with jQuery or JavaScript.
Example Code
Here’s the code snippet that illustrates the problem:
[[See Video to Reveal this Text or Code Snippet]]
Why Does This Happen?
The root of the issue lies in when the variable username is being set versus when it's being accessed. The line var username = $('# username').val(); runs once when the script is loaded, not each time the button is clicked. Consequently, if the user changes the input after the script runs, the variable username won't reflect the latest user input.
In a click event function, if you reuse the variable name username without redeclaring it, it might refer to the context of the event instead of the variable you intended to access.
The Solution
Step-by-Step Fix
To ensure that you correctly retrieve the username value every time the button is clicked, you should redefine the variable inside the click event function. Here's how to do it:
Move the variable declarations inside the event handler. This ensures that every time the button is clicked, you are fetching the latest input from the fields.
Remove any potential naming conflicts by only using meaningful names when declaring your variables.
Here’s the modified code that fixes the issue:
[[See Video to Reveal this Text or Code Snippet]]
Key Points
Variable Scope: Understand where and when your variables are defined. Variables defined outside of an event handler will not update automatically based on user input.
Event Handler Context: Each time an event is triggered, execute any code needed to capture fresh data.
Testing: Always test your code by interacting with the form to check that values are correctly captured within event handlers.
Conclusion
By placing your variable declarations inside the event function, you can access the most recent values from your form inputs. This simple change could save you a lot of debugging headache in the future.
Make sure to apply this pattern in your jQuery projects, and handle user inputs correctly every time!
If you have any further questions or run into other jQuery issues along the way, don’t hesitate to ask for help.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Cannot access variable inside event
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Fixing the "Cannot Access Variable Inside Event" Issue in jQuery
If you're working with jQuery to handle form submissions or button clicks, you may have encountered an annoying problem: trying to access a variable inside an event function, only to find it returning an empty string. Let's explore why this happens and how to fix it effectively.
Understanding the Problem
Imagine you've set up a form with fields for a username, email, and password. When you try to retrieve the value of the username variable in a click event, you get an empty response. You might be puzzled, especially when you see that the variable is set correctly outside the event function. This confusion is common among developers working with jQuery or JavaScript.
Example Code
Here’s the code snippet that illustrates the problem:
[[See Video to Reveal this Text or Code Snippet]]
Why Does This Happen?
The root of the issue lies in when the variable username is being set versus when it's being accessed. The line var username = $('# username').val(); runs once when the script is loaded, not each time the button is clicked. Consequently, if the user changes the input after the script runs, the variable username won't reflect the latest user input.
In a click event function, if you reuse the variable name username without redeclaring it, it might refer to the context of the event instead of the variable you intended to access.
The Solution
Step-by-Step Fix
To ensure that you correctly retrieve the username value every time the button is clicked, you should redefine the variable inside the click event function. Here's how to do it:
Move the variable declarations inside the event handler. This ensures that every time the button is clicked, you are fetching the latest input from the fields.
Remove any potential naming conflicts by only using meaningful names when declaring your variables.
Here’s the modified code that fixes the issue:
[[See Video to Reveal this Text or Code Snippet]]
Key Points
Variable Scope: Understand where and when your variables are defined. Variables defined outside of an event handler will not update automatically based on user input.
Event Handler Context: Each time an event is triggered, execute any code needed to capture fresh data.
Testing: Always test your code by interacting with the form to check that values are correctly captured within event handlers.
Conclusion
By placing your variable declarations inside the event function, you can access the most recent values from your form inputs. This simple change could save you a lot of debugging headache in the future.
Make sure to apply this pattern in your jQuery projects, and handle user inputs correctly every time!
If you have any further questions or run into other jQuery issues along the way, don’t hesitate to ask for help.