filmov
tv
[Unity] Creating a 2D Platformer (E10. variable jump height)
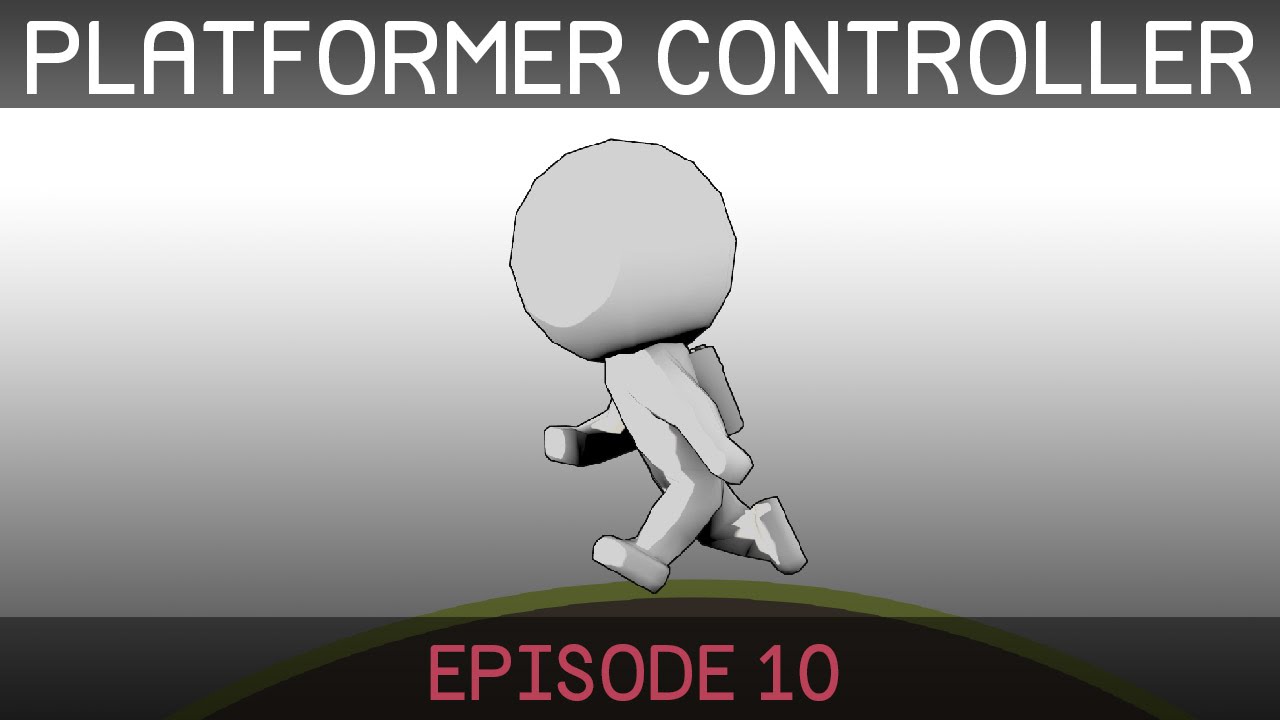
Показать описание
Learn how to create a 2D platformer controller in Unity that can reliably handle slopes and moving platforms.
Or a once-off donation through PayPal:
Or a once-off donation through PayPal:
Complete Unity 2D Platformer Tutorial – Easy for Beginners 2024
How to make a 2D platformer - Unity Tutorial Crash Course
Ultimate 2D Platformer Controller in Unity (source code provided)
How I made an Excellent Platformer
Learn How to Make a 2D Platformer in Unity 2022 - FULL GAMEDEV COURSE!
Make a Unity 2D Camera Follow Player in 15 seconds
How To Make A 2D Platformer In Unity (THE FASTEST WAY!)
How To Fail At Making A Platformer
Started Making my First Game | Unity Game DevLog
How To Make 2D Platformers (Unity Fundamentals Tutorial)
Improve your Platformer with Acceleration | Examples in Unity
The Unity Tutorial For Complete Beginners
How to make a good platforming character (Developing 6)
ULTIMATE 2D Platformer Controller for Unity
2D JUMP IN UNITY IN UNDER 1 MINUTE
Full Course on How to make a 2d game in UNITY with C# | Beginners& Intermediate
How I Create BEAUTIFUL LEVELS for my #indiegame #metroidvania #shorts
Easy Tilemaps and Dynamic Auto Tiling - Unity 2D
[Unity] Creating a 2D Platformer (E01. set-up)
Improve your Platformer’s Jump (and Wall Jump) | Unity
I Wish I Had Known This Before I Started Unity Game Development...
Tilemaps | Unity 2D Platformer Tutorial #3
AUTOMATIC TILEMAP COLLIDER IN UNDER 1 MINUTE UNITY 2D
How To Make A 2.5D Game - Unity
Комментарии