filmov
tv
Java Programming
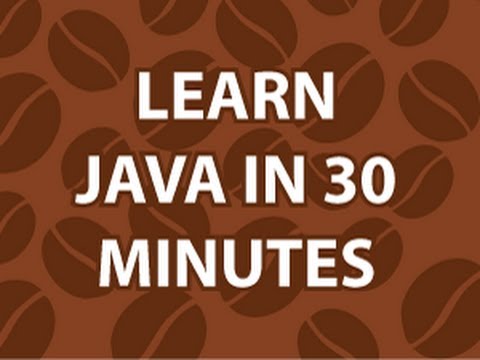
Показать описание
MY UDEMY COURSES ARE 87.5% OFF TIL December 19th ($9.99) ONE IS FREE
In this Java programming Tutorial I'll teach you all of the core knowledge needed to write Java code in 30 minutes. This is the most popular request from everyone.
I specifically cover the following topics: primitive data types, comments, class, import, Scanner, final, Strings, static, private, protected, public, constructors, math, hasNextLine, nextLine, getters, setters, method overloading, Random, casting, toString, conversion from Strings to primitives, converting from primitives to Strings, if, else, else if, print, println, printf, logical operators, comparison operators, ternary operator, switch, for, while, break, continue, do while, polymorphism, arrays, for each, multidimensional arrays and more.
*Watch More Learn in One Videos*
Like the channel? Consider becoming a Patreon! Check it out here:
In this Java programming Tutorial I'll teach you all of the core knowledge needed to write Java code in 30 minutes. This is the most popular request from everyone.
I specifically cover the following topics: primitive data types, comments, class, import, Scanner, final, Strings, static, private, protected, public, constructors, math, hasNextLine, nextLine, getters, setters, method overloading, Random, casting, toString, conversion from Strings to primitives, converting from primitives to Strings, if, else, else if, print, println, printf, logical operators, comparison operators, ternary operator, switch, for, while, break, continue, do while, polymorphism, arrays, for each, multidimensional arrays and more.
*Watch More Learn in One Videos*
Like the channel? Consider becoming a Patreon! Check it out here:
Learn Java in 14 Minutes (seriously)
Java Tutorial for Beginners
Java Programming for Beginners – Full Course
Java in 100 Seconds
What Is Java? | Java In 5 Minutes | Java Programming | Java Tutorial For Beginners | Simplilearn
Learn Java in One Video - 15-minute Crash Course
Java Full Course for free ☕
Intro to Java Programming - Course for Absolute Beginners
CODE || Programming In Java Week 5 || NPTEL ANSWERS | My Swayam | IIT Kharagpur #nptel2024 #myswayam
Java is mounting a huge comeback
Java Full Course [NEW]
Fastest Way to Learn ANY Programming Language: 80-20 rule
Introduction to Java Programming
❤❤❤ Make a full heart java code ❤❤💻💻💻📒📒💻😘🤗❤❤
Object-Oriented Programming Java Tutorial (Java OOP) #71
Java Programming Tutorial - 01 - Introduction To Methods
Does Java SUCK!? 👩💻 #programming #technology #software #tech #code #career #java
Java Tutorial for Beginners 2023
Big Update in Java for Learners and Trainers
Java for the Haters in 100 Seconds
Anatomy of Java Program
#01 Introduction to Java Programming Tutorial Series | For Beginners in Tamil | Error Makes Clever
What is PUBLIC STATIC VOID MAIN ( STRING[] Args ) in JAVA | Most Asked interview Question
Java tutorial for complete beginners with interesting examples - Easy-to-follow Java programming
Комментарии