filmov
tv
Swift 3 Fun Algorithms: Abstract Syntax Tree (Warning: Somewhat Difficult Recursion)
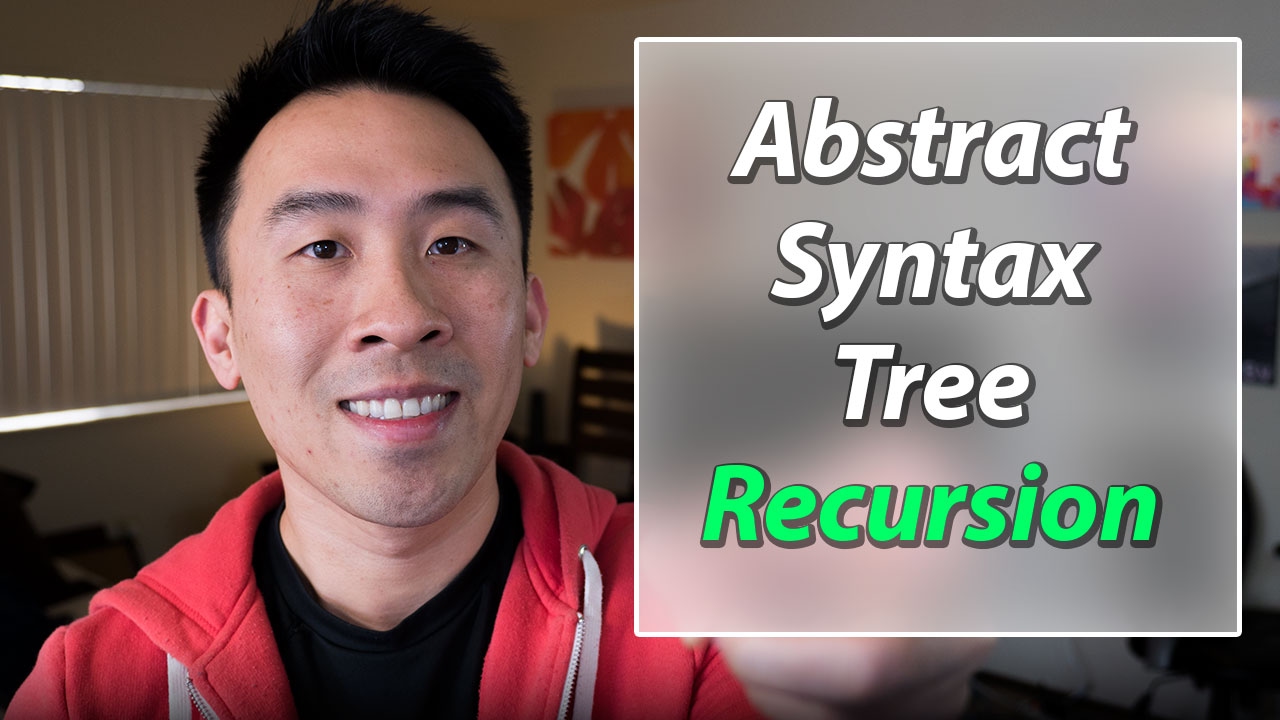
Показать описание
Let's continue with our theme from last week and implement an even harder recursion algorithm this time. Today, I'll introduce to you something called an Abstract Syntax Tree which is typically used by compilers to figure out what kind of code you are writing.
Because this is a somewhat difficult challenge, it's useful to walk through each use case one step at a time. This way you can see clearly how the recursion should be performed.
Facebook Group
iOS Basic Training Course
Completed Source Code
Because this is a somewhat difficult challenge, it's useful to walk through each use case one step at a time. This way you can see clearly how the recursion should be performed.
Facebook Group
iOS Basic Training Course
Completed Source Code
Swift 3 Fun Algorithms: Abstract Syntax Tree (Warning: Somewhat Difficult Recursion)
Swift 3 Fun Algorithms: Wrapping an Array
Swift 3 Fun Algorithms: Recursive Search through Binary Tree
Swift 3 Fun Algorithms: Filter, Map, Reduce Higher Order Functions
Swift Fun Algorithms: How to Create a Retain Cycle?
Swift Fun Algorithms #3: Factorials & Recursion
Swift Fun Algorithms #1: FizzBuzz
I gave 127 interviews. Top 5 Algorithms they asked me.
Abstract relationships in Swift by Andrei Raifura
Top 6 Coding Interview Concepts (Data Structures & Algorithms)
Parsing Algorithms. Lecture [5/22] Abstract Syntax Trees
Power of Recursion in Swift (Nth Fibonacci Number)
Harnessing The Power of Abstract Syntax Trees by Jamund Ferguson
8 Design Patterns EVERY Developer Should Know
10 Design Patterns Explained in 10 Minutes
Why You Shouldn't Nest Your Code
Interview Algorithm Challenge: Reverse Linked List - Swift
Tech burner gf @techburner
try! Swift Tokyo 2018 - Using Swift to Visualize Algorithms
How I would learn to code
Maximum Depth Of Binary Tree #algorithm #leetcode #swift
5 Design Patterns That Are ACTUALLY Used By Developers
Nesting 'If Statements' Is Bad. Do This Instead.
James Titcumb - Climbing the Abstract Syntax Tree
Комментарии