filmov
tv
Swift Fun Algorithms #1: FizzBuzz
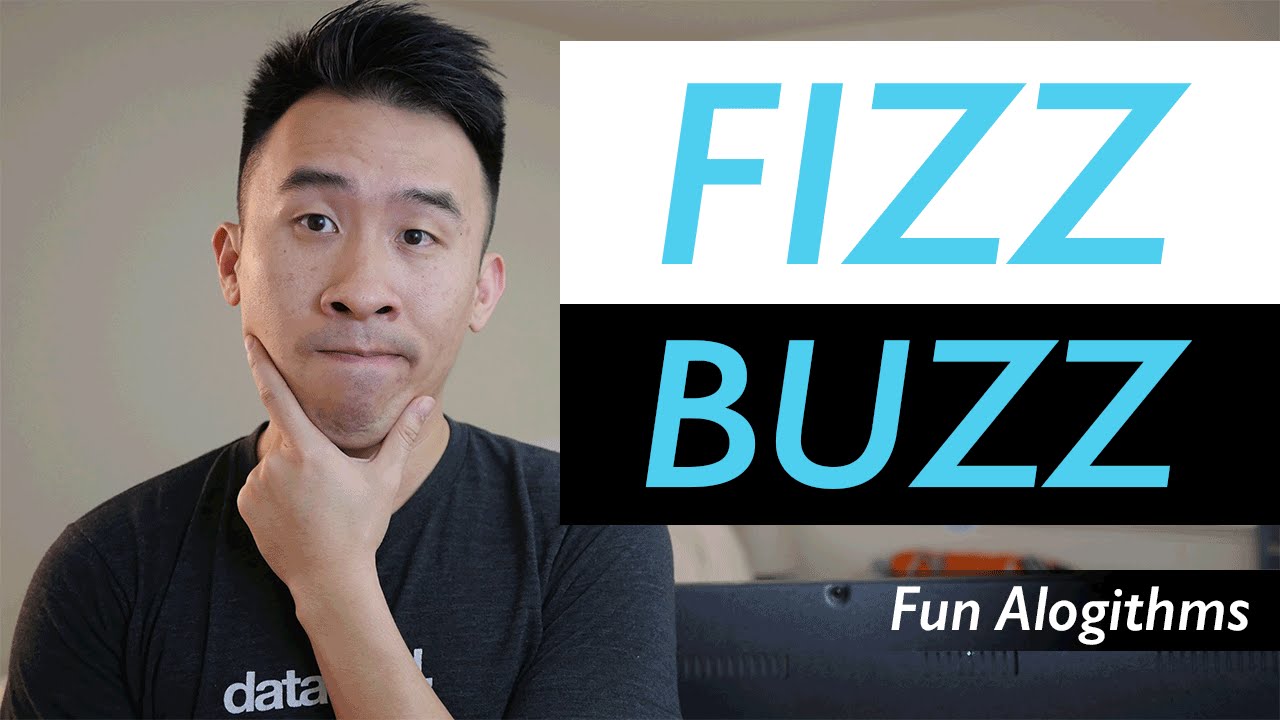
Показать описание
We're going to take a quick break from building apps today to go over a very simple and straightforward algorithm called FizzBuzz. The general idea behind this algorithm is to run through a list of numbers and print Fizz when divisible by 3, Buzz when divisible by 5, and FizzBuzz when divisible by both 3 and 5.
Code available here:
Code available here:
Swift Fun Algorithms #1: FizzBuzz
FizzBuzz with Swift - Six Ways!
FizzBuzz Algorithm in Swift | Algorithms with Swift
Algorithms In Swift: Fizz Buzz (DAY 14)
Swift Fun Algorithms #4: Most Common Name in Array
Swift Programming - Fizz Buzz Challenge
FizzBuzz: One Simple Interview Question
Swift interview question - FIZZBUZZ
Test-Driven Development in Swift – Fizz Buzz
Swift: Fun Algorithms - Counting Palindromes
Swift Fun Algorithms: How to Draw a Circle using Math
Swift 3 Fun Algorithms: Wrapping an Array
Micro-objects / Swift Code Katas #3: FizzBuzz
Swift Fun Algorithms: Closure Reference Cycles
FIZZBUZZ PROGRAMMING EXERCISE
Fizz Buzz Implementation
FizzBuzz - Python | Coding Interview Question
Write Better Code with Swift Algorithms
Swift 5 Brand New isMultiple(of: x) FizzBuzz and Clean Switch Statements
First Duplicate !!Swift Coding Challenge!!
Swift Fun Algorithms: Retain Cycle Correction!
Fizzbuzz in ONE LINE of Code #Shorts
Swift 3 Fun Algorithms: Filter, Map, Reduce Higher Order Functions
Swift 3 Fun Algorithms: Recursive Search through Binary Tree
Комментарии