filmov
tv
Data transfer using Socket in C
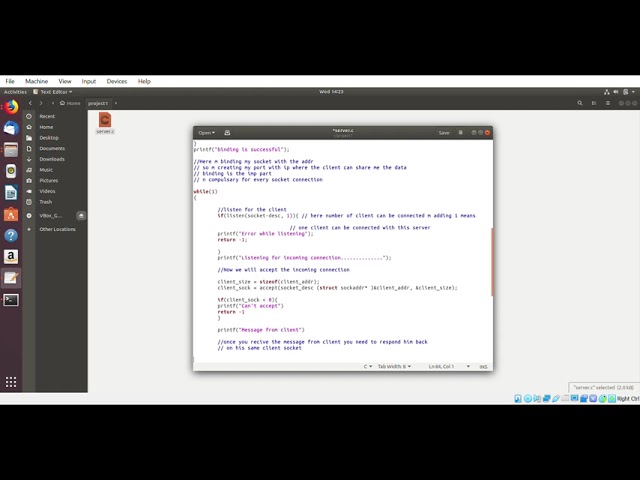
Показать описание
Hello guys
In this tutorial, you will learn how to perform a file (data) transfer over a TCP socket in the C programming language. You will see how many clients send the data to the server which are connected to the server, here the code describes we can connect as many clients with small modifications in the code.
sockets are used and manipulated to create a connection between software. Sockets are used to connect software either between different computers or within the same computer so the programs can share data
What is TCP
TCP refers to the Transmission Control Protocol, which is a highly efficient and reliable protocol designed for end-to-end data transmission over an unreliable network.
What is UDP?
The UDP or User Datagram Protocol, a communication protocol used for transferring data across the network. It is an unreliable and connectionless communication protocol as it does not establish a proper connection between the client and the server. It is used for time-sensitive applications like gaming, playing videos, or Domain Name System (DNS) lookups. UDP is a faster communication protocol as compared to the TCP.
//NOTE use angle brackets which are removed from the code
I wrote use smaller than use small angled brackets
I wrote use greater than use greater angled brackets
Given Code with steps:
#include stdio.h
#include string.h
#include sys/socket.h
#include arpa/inet.h
int main(void)
{
int socket_desc, client_sock, client_size;
struct sockaddr_in server_addr, client_addr;//we created struct to bind the data
char server_message[2000], client_message[2000];//this is where we going to pass n recive the data
//client buffer need to be empty every time
memset(server_message, '\0', sizeof(server_message));
memset(client_message, '\0', sizeof(client_message));
//now the data is NILL
//Create socket
socket_desc = socket(AF_INET, SOCK_STREAM, 0);
//0 is for tcp in that you can add 1 for udp
// SOCK_STREAM indicate type of socket TCP or UDP
// AF_inet is the addr family of socket
if(socket_desc use smaller then 0){
printf("Error");
return -1;
}
printf("socket is created ");
//socket is created or not
//now nedd to set port and IP
// once you create por and IP
// Need to bind with the socket
if(bind(socket_desc,(struct sockaddr*)&server_addr, sizeof(server_addr)) use greater then 0){
printf("Couldn't bind");
return -1;
}
printf("binding is successful\n");
//Here m binding my socket with the addr
// so m creating my port with ip where the client can share me the data
// binding is the imp part
// n compulsary for every socket connection
while(1)
{
//listen for the client
if(listen(socket_desc, 1)){ // here number of client can be connected m adding 1 means
// one client can be connected with this server
printf("Error while listening");
return -1;
}
printf("Listening for incoming connection..............\n");
//Now we will accept the incoming connection
client_size = sizeof(client_addr);
client_sock = accept(socket_desc, (struct sockaddr* )&client_addr, &client_size);
if(client_sock use smaller then 0){
printf("Can't accept");
return -1;
}
// before this we need to see client connection
if(recv(client_sock, client_message, sizeof(client_message), 0) use smaller then 0){
printf("Couldn't receive");
return -1;
}
printf("Message from client: %s\n", client_message);
//once you recive the message from client you need to respond him back
// on his same client socket
//now for responding
strcpy(server_message, "This is the server message: ");
if(send(client_sock, server_message, strlen(server_message), 0) use smaller then 0){
printf("Can't send");
return -1;
}
}
//close(client_sock);
//close(socket_desc);
return 0;
}
In this tutorial, you will learn how to perform a file (data) transfer over a TCP socket in the C programming language. You will see how many clients send the data to the server which are connected to the server, here the code describes we can connect as many clients with small modifications in the code.
sockets are used and manipulated to create a connection between software. Sockets are used to connect software either between different computers or within the same computer so the programs can share data
What is TCP
TCP refers to the Transmission Control Protocol, which is a highly efficient and reliable protocol designed for end-to-end data transmission over an unreliable network.
What is UDP?
The UDP or User Datagram Protocol, a communication protocol used for transferring data across the network. It is an unreliable and connectionless communication protocol as it does not establish a proper connection between the client and the server. It is used for time-sensitive applications like gaming, playing videos, or Domain Name System (DNS) lookups. UDP is a faster communication protocol as compared to the TCP.
//NOTE use angle brackets which are removed from the code
I wrote use smaller than use small angled brackets
I wrote use greater than use greater angled brackets
Given Code with steps:
#include stdio.h
#include string.h
#include sys/socket.h
#include arpa/inet.h
int main(void)
{
int socket_desc, client_sock, client_size;
struct sockaddr_in server_addr, client_addr;//we created struct to bind the data
char server_message[2000], client_message[2000];//this is where we going to pass n recive the data
//client buffer need to be empty every time
memset(server_message, '\0', sizeof(server_message));
memset(client_message, '\0', sizeof(client_message));
//now the data is NILL
//Create socket
socket_desc = socket(AF_INET, SOCK_STREAM, 0);
//0 is for tcp in that you can add 1 for udp
// SOCK_STREAM indicate type of socket TCP or UDP
// AF_inet is the addr family of socket
if(socket_desc use smaller then 0){
printf("Error");
return -1;
}
printf("socket is created ");
//socket is created or not
//now nedd to set port and IP
// once you create por and IP
// Need to bind with the socket
if(bind(socket_desc,(struct sockaddr*)&server_addr, sizeof(server_addr)) use greater then 0){
printf("Couldn't bind");
return -1;
}
printf("binding is successful\n");
//Here m binding my socket with the addr
// so m creating my port with ip where the client can share me the data
// binding is the imp part
// n compulsary for every socket connection
while(1)
{
//listen for the client
if(listen(socket_desc, 1)){ // here number of client can be connected m adding 1 means
// one client can be connected with this server
printf("Error while listening");
return -1;
}
printf("Listening for incoming connection..............\n");
//Now we will accept the incoming connection
client_size = sizeof(client_addr);
client_sock = accept(socket_desc, (struct sockaddr* )&client_addr, &client_size);
if(client_sock use smaller then 0){
printf("Can't accept");
return -1;
}
// before this we need to see client connection
if(recv(client_sock, client_message, sizeof(client_message), 0) use smaller then 0){
printf("Couldn't receive");
return -1;
}
printf("Message from client: %s\n", client_message);
//once you recive the message from client you need to respond him back
// on his same client socket
//now for responding
strcpy(server_message, "This is the server message: ");
if(send(client_sock, server_message, strlen(server_message), 0) use smaller then 0){
printf("Can't send");
return -1;
}
}
//close(client_sock);
//close(socket_desc);
return 0;
}