filmov
tv
Understanding the Right Ref Type for TextInput in React Native with TypeScript
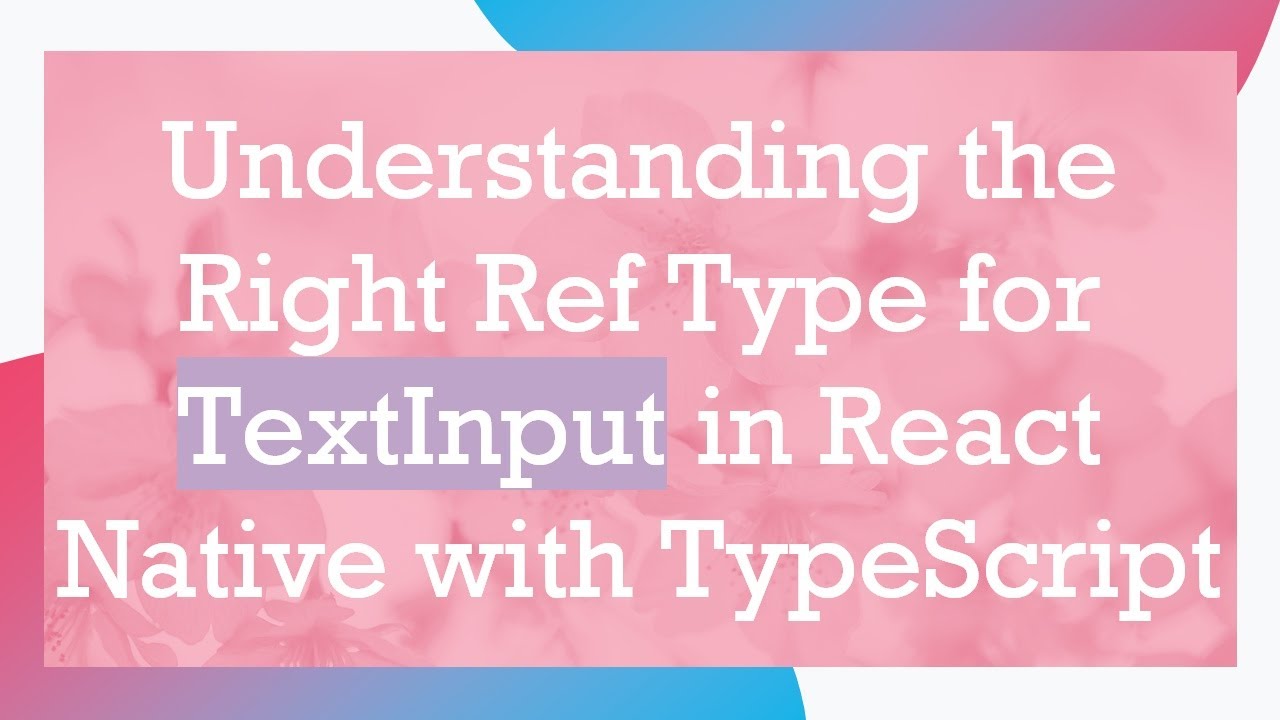
Показать описание
Learn how to correctly set up `TextInput` refs in React Native using TypeScript with this comprehensive guide.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: react native textinput ref typescript
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Solving Ref Type Issues in React Native TextInput with TypeScript
When developing applications with React Native, especially using TypeScript, you might run into issues regarding the ref types used in your components. One common question among developers is: What’s the right type to use when creating a ref for a TextInput? This question arises especially when you encounter errors similar to the following when trying to utilize a TextInput ref.
Common Error Scenario
You might find a use case similar to this:
[[See Video to Reveal this Text or Code Snippet]]
However, this results in an error message, indicating:
[[See Video to Reveal this Text or Code Snippet]]
This error message can be quite confusing, particularly if you're new to TypeScript and handling refs in React Native. So, let’s break down the solution.
Understanding the Problem
The root of the issue is that the type HTMLInputElement is specific to web development and does not apply to React Native. React Native's components, such as TextInput, need to reference the React Native types instead. Using the wrong type leads to type incompatibility, which is what you're experiencing.
The Solution: Correctly Defining Ref Types
To resolve the ref type error for TextInput in React Native when using TypeScript, you can use the following approach:
Step 1: Use the Correct Type for Ref
You should define the inputRef using typeof TextInput instead of HTMLInputElement. Here is the correct way to set it up:
[[See Video to Reveal this Text or Code Snippet]]
Full Example
Here’s the complete implementation with the correct ref type:
[[See Video to Reveal this Text or Code Snippet]]
Key Takeaway
When using refs in React Native with TypeScript, always ensure that you're referencing the correct component types provided by React Native. If you're dealing with React components, use typeof Component. This adjustment helps TypeScript understand the types properly and eliminates errors related to type mismatches.
Conclusion
By understanding the nuances of type management in TypeScript and how it integrates with React Native, you can effectively handle refs without running into unnecessary issues. This guide outlines the steps needed to resolve common ref type problems in React Native's TextInput. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: react native textinput ref typescript
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Solving Ref Type Issues in React Native TextInput with TypeScript
When developing applications with React Native, especially using TypeScript, you might run into issues regarding the ref types used in your components. One common question among developers is: What’s the right type to use when creating a ref for a TextInput? This question arises especially when you encounter errors similar to the following when trying to utilize a TextInput ref.
Common Error Scenario
You might find a use case similar to this:
[[See Video to Reveal this Text or Code Snippet]]
However, this results in an error message, indicating:
[[See Video to Reveal this Text or Code Snippet]]
This error message can be quite confusing, particularly if you're new to TypeScript and handling refs in React Native. So, let’s break down the solution.
Understanding the Problem
The root of the issue is that the type HTMLInputElement is specific to web development and does not apply to React Native. React Native's components, such as TextInput, need to reference the React Native types instead. Using the wrong type leads to type incompatibility, which is what you're experiencing.
The Solution: Correctly Defining Ref Types
To resolve the ref type error for TextInput in React Native when using TypeScript, you can use the following approach:
Step 1: Use the Correct Type for Ref
You should define the inputRef using typeof TextInput instead of HTMLInputElement. Here is the correct way to set it up:
[[See Video to Reveal this Text or Code Snippet]]
Full Example
Here’s the complete implementation with the correct ref type:
[[See Video to Reveal this Text or Code Snippet]]
Key Takeaway
When using refs in React Native with TypeScript, always ensure that you're referencing the correct component types provided by React Native. If you're dealing with React components, use typeof Component. This adjustment helps TypeScript understand the types properly and eliminates errors related to type mismatches.
Conclusion
By understanding the nuances of type management in TypeScript and how it integrates with React Native, you can effectively handle refs without running into unnecessary issues. This guide outlines the steps needed to resolve common ref type problems in React Native's TextInput. Happy coding!