filmov
tv
Happy Number - Leetcode 202 - Python
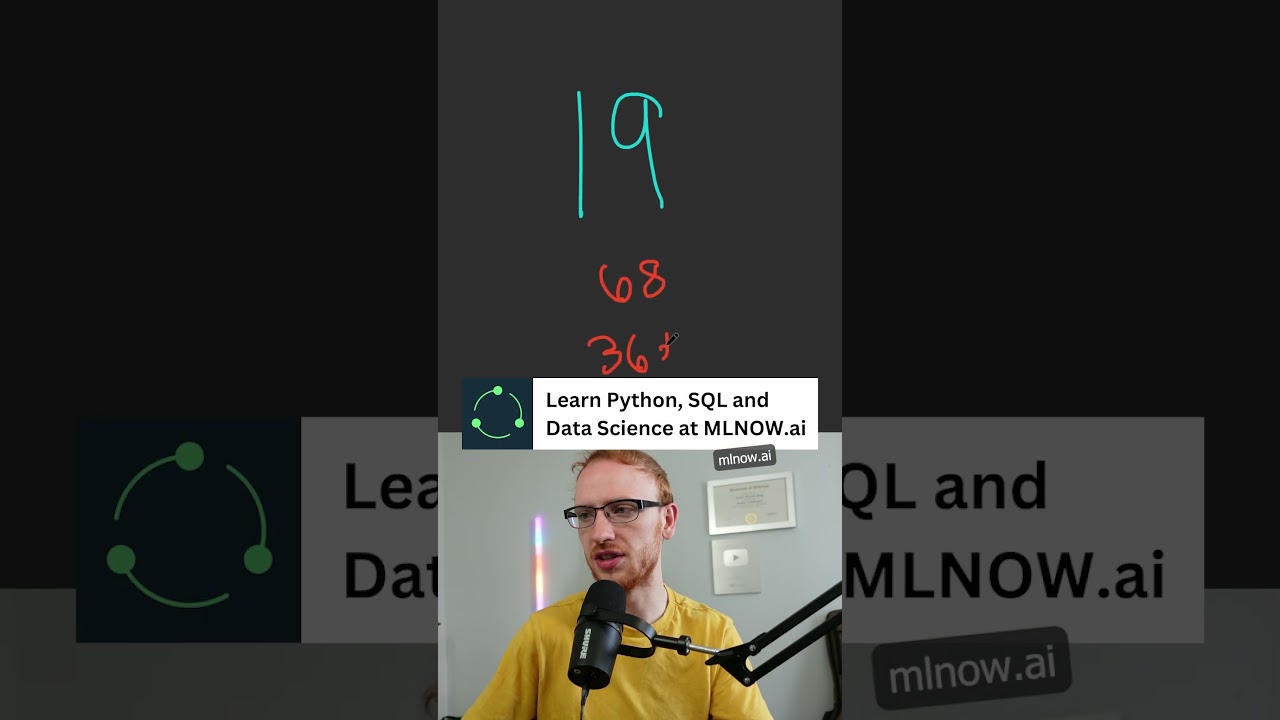
Показать описание
FAANG Coding Interviews / Data Structures and Algorithms / Leetcode
Happy Number - Leetcode 202 - Python
Happy Number - Leetcode 202
Google LOVES This Coding Interview Question! | Happy Number - Leetcode 202
Happy Number (LeetCode 202) | Full solution with with easy explanation | Study Algorithms
Happy Number - Leetcode 202 - Python
Happy Number | LeetCode 202 | Java Solution
LeetCode 202 - Happy Number | Java Solution
Разбор задачи 202 leetcode.com Happy Number. Решение на C++
Счастливое или не очень число. Leetcode 202. Happy Number
Leetcode 202. Happy Number
leetcode 202 Happy Number
Happy Number [LeetCode 202] | Math | Approach and Intuition
LeetCode#202 Happy Number - Python
Happy Number - Leetcode 202
LEETCODE 202 (JAVASCRIPT) | HAPPY NUMBER
Happy Number LeetCode 202 C#
Software Engineer/Developer Interview Question: 202. Happy Number
Happy Number - LeetCode 202 - Python #happynumber #leetcode
202 Happy Number LeetCode (Google Interview Question) JavaScript
LeetCode #202: Happy Number | Facebook Interview Question | C++
Happy Number Javascript 202 Leetcode | Google Interview question
Happy Number | LeetCode 202 | Python Solution
How to Solve '202 Happy Number' on LeetCode? - Javascript
LEETCODE 202 HAPPY NUMBER PYTHON
Комментарии