filmov
tv
Binary Search Tree Insertion (Iterative method)
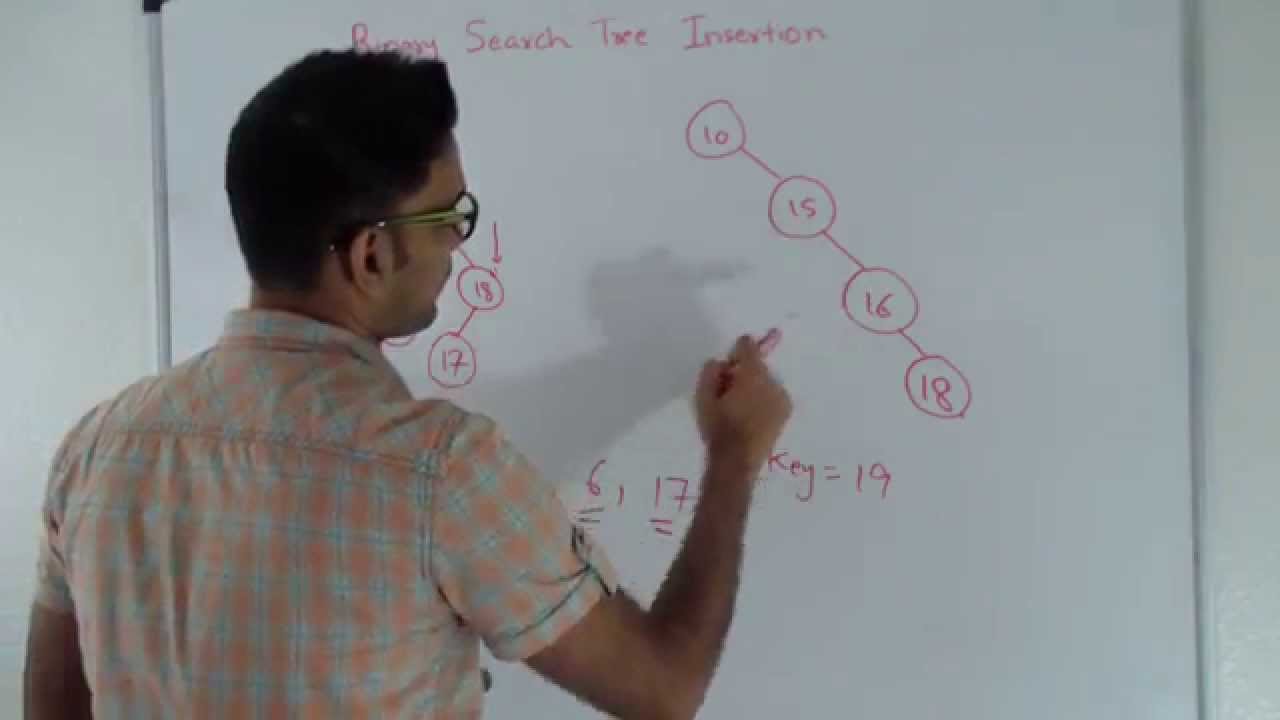
Показать описание
Insert into binary search tree.
Binary Search Tree Insertion (Iterative method)
Binary Search Tree Insertion
Insertion on a Binary Search Tree...Iterative Approach
Binary search tree insertion with visualizer | Iterative | JavaScript
Binary Search Tree : Insertion | Iterative | HackerRank | Tree | Python
Insertion in Binary Search Tree | Insertion in BST | Iteratively & Recursively | DSA-One Course ...
Insert into a Binary Search Tree - Leetcode 701 - Python
Binary Search Tree - implementing an insert method - iterative
Leetcode - Insert into a Binary Search Tree (Python)
Binary Search Trees - Iterative Search Function
Iterative Search in a Binary Search Tree
Insert a node in a binary search tree - an iterative implementation
L50. Binary Search Tree Iterator | BST | O(H) Space
Binary Search Tree in Python
Lec-53: Binary Search Tree in Data Structure | Insertion and Traversal in BST
L43. Insert a given Node in Binary Search Tree | BST | C++ | Java
Insertion in a Binary Search Tree
Insert into a Binary Search Tree | Leetcode 701 | Recursive Iterative | Google Amazon | BST
Insert a Node in BST💥: An Iterative Solution Explained | C++✌#geeksforgeeks #binarysearchtree
Binary Search Tree - Insertion Pseudo Code
128 - Binary Search Tree: Insertion | Trees | Hackerrank Solution | Python
HackerRank - Binary Search Tree : Insertion
Coding Interview Tutorial 23: Validate Binary Search Tree (Iterative) [LeetCode]
Binary Tree Insertion Algorithm (in Rust)
Комментарии