filmov
tv
Cryptography: Caesar Cipher Python 3
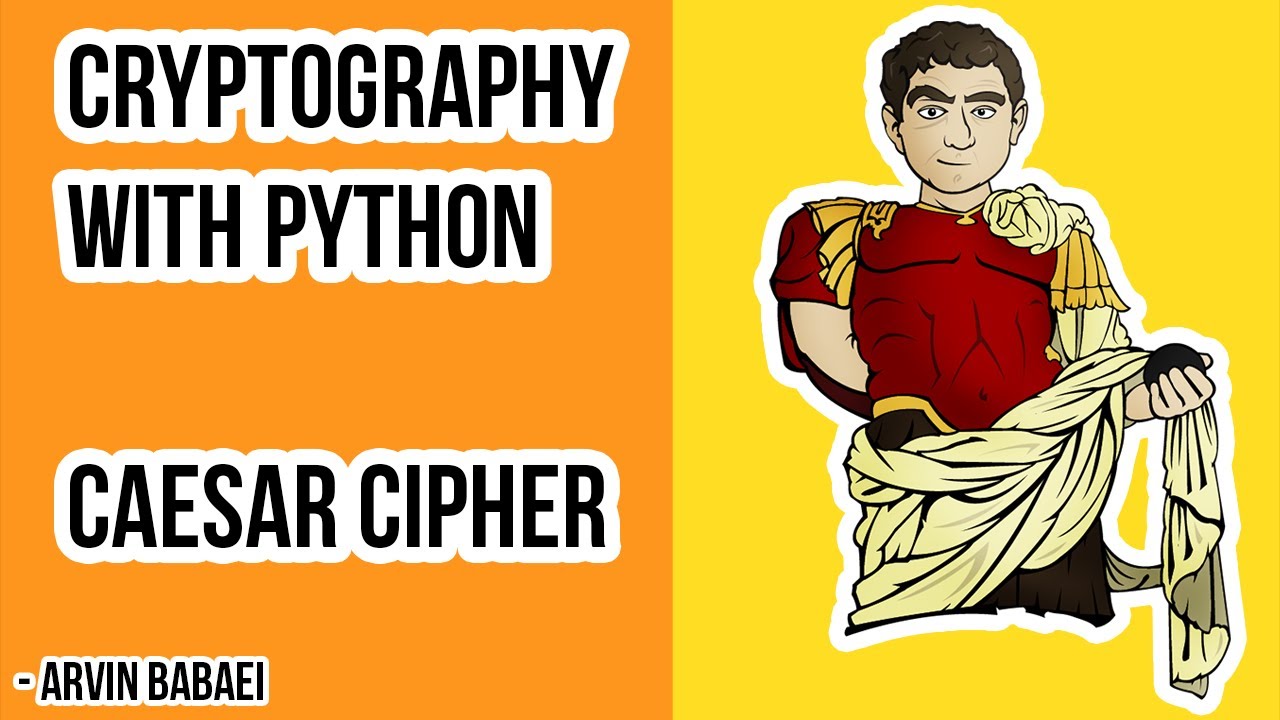
Показать описание
Give a thumbs up 👍🏻 if my code was useful to you.
Showing compilation and execution of my code in Python 3, which is capable of Encrypting, Decrypting, Brute force Attacking using Caesar Cipher cryptography.
#python #sublime #caesarcipher #cryptography
Develop a single program using any programming language (e.g. Java, Python, C++, etc) that:
a. Encrypts and decrypts a message using Cesar’s Cipher. The user encrypting the message is prompted to enter a message and then a key that indicates the displacement. The user decrypting the message is prompted to enter the encrypted message and the key.
b. Decrypts and displays all (26) possible solutions.
# Writen by Arvin Babaei
#Caesar Cipher cryptography code in Python 3
def encrypt(message, key):
encrypt_str = ''
for ch in message:
encrypt_str += chr(ord('A') + ((ord(ch) - ord('A') + key + 26) % 26))
encrypt_str += chr(ord('a') + ((ord(ch) - ord('a') + key + 26) % 26))
else:
encrypt_str += ch
return encrypt_str
def decrypt (message, key):
decrypt_str = ''
for ch in message:
decrypt_str += chr(ord('A') + ((ord(ch) - ord('A') - key + 26) % 26))
decrypt_str += chr(ord('a') + ((ord(ch) - ord('a') - key + 26) % 26))
else:
decrypt_str += ch
return decrypt_str
def brute (message, key):
brute_str = ''
for ch in message:
brute_str += chr(ord('A') + ((ord(ch) - ord('A') - key + 26) % 26))
brute_str += chr(ord('a') + ((ord(ch) - ord('a') - key + 26) % 26))
else:
brute_str += ch
return brute_str
if __name__ == '__main__':
while True:
input_message = input("Enter message: ")
option = input("Encrypt(E) or Decrypt(D) or Brute froce (B)?: ")
shift_amount = int(input("Enter key: "))
print(encrypt(input_message, shift_amount))
shift_amount = int(input("Enter key: "))
print(decrypt(input_message, shift_amount))
for shift_amount in range(26):
print ("using key: " + str(shift_amount))
print(brute(input_message, shift_amount))
else:
print("please enter valid input")
go_again = input("Go again?(Y/N):")
break;
Showing compilation and execution of my code in Python 3, which is capable of Encrypting, Decrypting, Brute force Attacking using Caesar Cipher cryptography.
#python #sublime #caesarcipher #cryptography
Develop a single program using any programming language (e.g. Java, Python, C++, etc) that:
a. Encrypts and decrypts a message using Cesar’s Cipher. The user encrypting the message is prompted to enter a message and then a key that indicates the displacement. The user decrypting the message is prompted to enter the encrypted message and the key.
b. Decrypts and displays all (26) possible solutions.
# Writen by Arvin Babaei
#Caesar Cipher cryptography code in Python 3
def encrypt(message, key):
encrypt_str = ''
for ch in message:
encrypt_str += chr(ord('A') + ((ord(ch) - ord('A') + key + 26) % 26))
encrypt_str += chr(ord('a') + ((ord(ch) - ord('a') + key + 26) % 26))
else:
encrypt_str += ch
return encrypt_str
def decrypt (message, key):
decrypt_str = ''
for ch in message:
decrypt_str += chr(ord('A') + ((ord(ch) - ord('A') - key + 26) % 26))
decrypt_str += chr(ord('a') + ((ord(ch) - ord('a') - key + 26) % 26))
else:
decrypt_str += ch
return decrypt_str
def brute (message, key):
brute_str = ''
for ch in message:
brute_str += chr(ord('A') + ((ord(ch) - ord('A') - key + 26) % 26))
brute_str += chr(ord('a') + ((ord(ch) - ord('a') - key + 26) % 26))
else:
brute_str += ch
return brute_str
if __name__ == '__main__':
while True:
input_message = input("Enter message: ")
option = input("Encrypt(E) or Decrypt(D) or Brute froce (B)?: ")
shift_amount = int(input("Enter key: "))
print(encrypt(input_message, shift_amount))
shift_amount = int(input("Enter key: "))
print(decrypt(input_message, shift_amount))
for shift_amount in range(26):
print ("using key: " + str(shift_amount))
print(brute(input_message, shift_amount))
else:
print("please enter valid input")
go_again = input("Go again?(Y/N):")
break;