filmov
tv
Extracting Data from Nested JSON in Rails
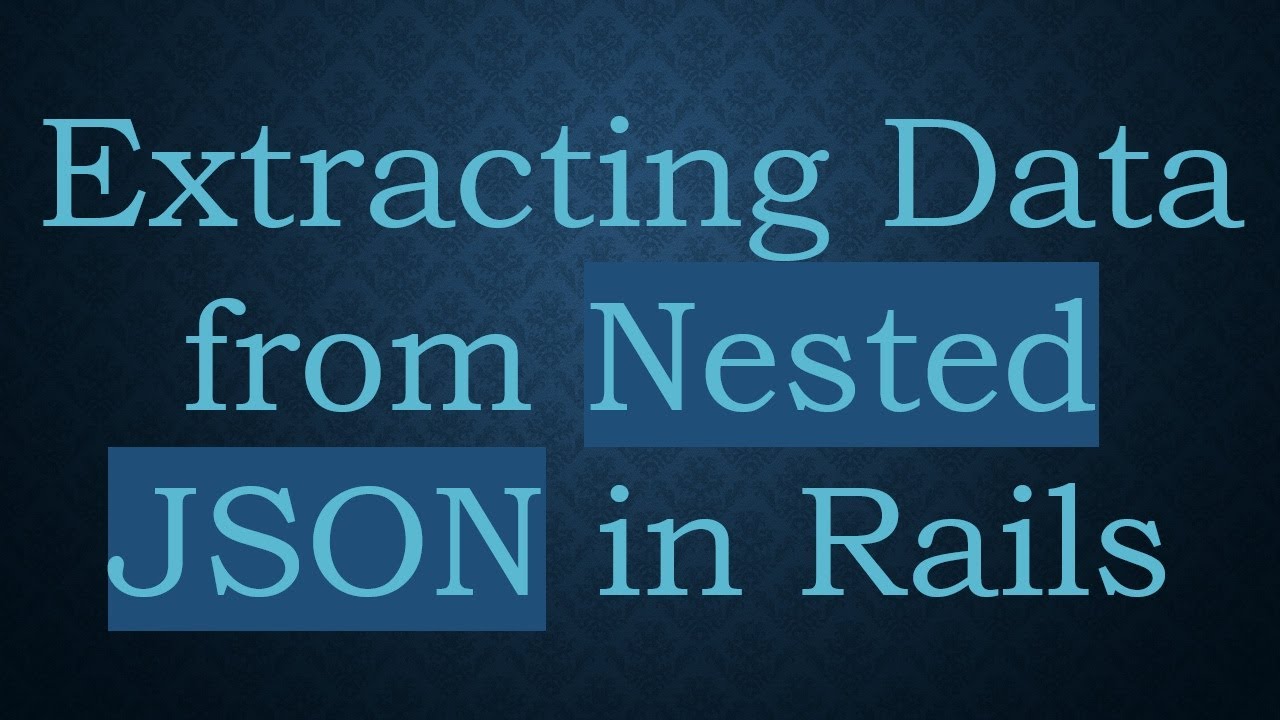
Показать описание
Learn how to effectively extract values from nested JSON objects in Rails. Dive into the details of accessing elements such as `responders`, `service_id`, and `codes`.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Rails how to extract data from nested JSON data
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Extracting Data from Nested JSON in Rails: A Step-by-Step Guide
When working with APIs or data files, you may encounter complex, nested JSON structures. These structures can sometimes be challenging to parse, especially when you want specific information from them. This guide will address a common problem: how to extract values from nested JSON in Rails.
The Problem
You’re dealing with a JSON object that contains multiple layers of information. For instance, consider the JSON data below, which includes a quotas array and nested objects within it:
[[See Video to Reveal this Text or Code Snippet]]
In this example, you might need to extract the following:
The number of responders from each quota.
The service_id and codes from each qualified object.
You attempted to get the data using the following Ruby code, but it did not work as expected:
[[See Video to Reveal this Text or Code Snippet]]
You received an error message: []': no implicit conversion of String into Integer, indicating a problem in how you were attempting to iterate over the data.
The Solution
To successfully extract values from nested JSON data, you'll need to carefully navigate through each level of the JSON object. Here's a clear, step-by-step guide to helping you achieve this.
Step 1: Parse the JSON Data
First, ensure that the JSON string is converted into a Ruby hash. You can do this using the following code:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Access the Quotas
Now that the data is converted, you can focus on the quotas. You'll want to loop through each quota in the quotas array:
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Access Nested Qualified Data
For each quota, you can then iterate over the qualified array. This allows you to grab the service_id and codes from each nested object:
[[See Video to Reveal this Text or Code Snippet]]
Complete Code Example
Here’s the complete solution brought together into a single code snippet:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Parsing nested JSON data in Rails may seem daunting at first, but by following these structured steps, you can efficiently extract the data you need. Whether it’s responders, service_id, or codes, you now have the tools to navigate and work with complex JSON structures.
Next time you encounter a similar situation, remember this guide. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Rails how to extract data from nested JSON data
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Extracting Data from Nested JSON in Rails: A Step-by-Step Guide
When working with APIs or data files, you may encounter complex, nested JSON structures. These structures can sometimes be challenging to parse, especially when you want specific information from them. This guide will address a common problem: how to extract values from nested JSON in Rails.
The Problem
You’re dealing with a JSON object that contains multiple layers of information. For instance, consider the JSON data below, which includes a quotas array and nested objects within it:
[[See Video to Reveal this Text or Code Snippet]]
In this example, you might need to extract the following:
The number of responders from each quota.
The service_id and codes from each qualified object.
You attempted to get the data using the following Ruby code, but it did not work as expected:
[[See Video to Reveal this Text or Code Snippet]]
You received an error message: []': no implicit conversion of String into Integer, indicating a problem in how you were attempting to iterate over the data.
The Solution
To successfully extract values from nested JSON data, you'll need to carefully navigate through each level of the JSON object. Here's a clear, step-by-step guide to helping you achieve this.
Step 1: Parse the JSON Data
First, ensure that the JSON string is converted into a Ruby hash. You can do this using the following code:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Access the Quotas
Now that the data is converted, you can focus on the quotas. You'll want to loop through each quota in the quotas array:
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Access Nested Qualified Data
For each quota, you can then iterate over the qualified array. This allows you to grab the service_id and codes from each nested object:
[[See Video to Reveal this Text or Code Snippet]]
Complete Code Example
Here’s the complete solution brought together into a single code snippet:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Parsing nested JSON data in Rails may seem daunting at first, but by following these structured steps, you can efficiently extract the data you need. Whether it’s responders, service_id, or codes, you now have the tools to navigate and work with complex JSON structures.
Next time you encounter a similar situation, remember this guide. Happy coding!