filmov
tv
Exploring the Best Practices for Key Checking in Nested Dictionaries in Python 3
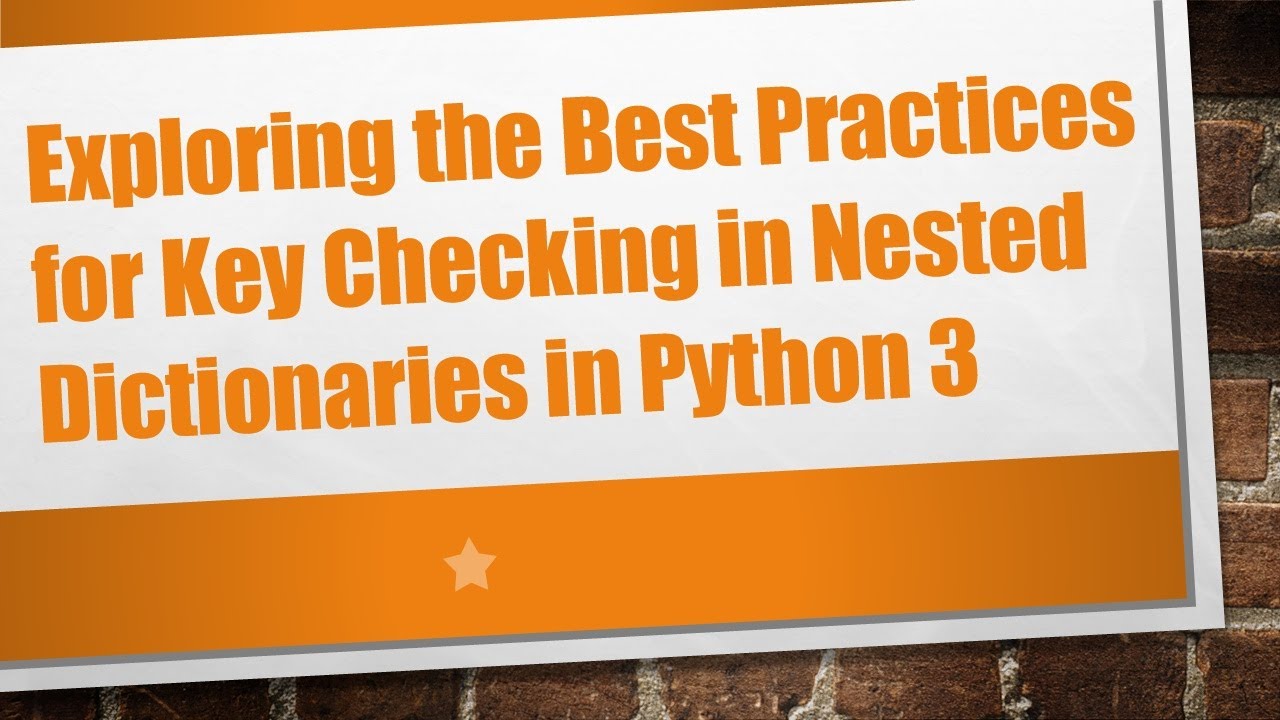
Показать описание
Discover whether using a try-except block is poor practice for checking keys in nested dictionaries in Python 3, and learn more efficient alternatives.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Python 3: Testing presence of each (sub)key in nested dict vs just using a try block? Poor practise or OK?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Navigating Nested Dictionaries in Python: A Guide to Efficient Key Checking
When working with nested dictionaries in Python, particularly when transitioning from other programming languages like Perl, one common challenge arises: how to efficiently check for the presence of keys before trying to access their values. In this post, we'll explore this topic in-depth and evaluate two prevalent methods: using conditional checks versus try-except blocks.
The Problem: Checking for Keys in Nested Dictionaries
Consider a scenario in Python where you have a nested dictionary and need to access certain values safely. For instance, let's say you have a dictionary a that may or may not contain the keys 'x' and 'y'. Attempting to directly access a['x']['y'] can lead to KeyError exceptions if those keys do not exist. Here’s a typical example:
[[See Video to Reveal this Text or Code Snippet]]
In the code above, we first check if the keys exist, and only then do we access them safely. While this approach works well, another method is to use a try-except block:
[[See Video to Reveal this Text or Code Snippet]]
However, this raises the question: Is the try-except block a poor practice?
Analyzing the Approaches
Method 1: Using Conditional Checks
The first method, where we check for the existence of keys, is clear and explicit. It enhances code readability and makes it straightforward for other developers (or yourself in the future) to understand the logic at play. Some advantages include:
Readability: It’s easy to see what conditions must be met to proceed.
Error Handling: By checking the keys, we avoid potentially hiding bugs with a broad exception catch.
Method 2: Using Try-Except Blocks
On the other hand, using a try-except block as shown in the second approach can be seen as less explicit. While it can sometimes lead to quicker code (especially when you're not sure about the structure of your data), it also has notable drawbacks:
Hides Errors: If some unexpected error occurs in the try block (not specifically a KeyError), it will be caught and ignored, possibly leading to more significant issues later.
Poor Clarity: It can be less obvious what part of the code could fail without explicitly checking conditions.
The Recommended Approach: Using get()
Python provides a more succinct and readable method to safely access nested dictionary values using the .get() method. This enables you to specify a default value if the key doesn’t exist, while also allowing for chained access to subkeys in a single line. Here’s how you can implement it:
[[See Video to Reveal this Text or Code Snippet]]
Reasons to Prefer .get()
Conciseness: This approach allows for cleaner, easier to read code without multiple checks.
Safety: It avoids raising an exception if the key isn’t found and simply returns None or an empty object instead.
Functional: Makes use of Python's capabilities, adhering to the language's philosophy of simplicity and elegance.
Conclusion: Finding the Right Balance
When working with nested dictionaries in Python, always weigh the options between clarity and brevity. While both the conditional checks and try-except blocks have their places, the .get() method often strikes the right balance. It allows accessing nested values gracefully and safely.
In summary, if you're transitioning to Python from another language, embrace Pythonic ways of handling data, and utilize methods like .get() to keep your code clean and free from unnecessary complexity.
Remember, clear and maintainable code should always be a priority!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Python 3: Testing presence of each (sub)key in nested dict vs just using a try block? Poor practise or OK?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Navigating Nested Dictionaries in Python: A Guide to Efficient Key Checking
When working with nested dictionaries in Python, particularly when transitioning from other programming languages like Perl, one common challenge arises: how to efficiently check for the presence of keys before trying to access their values. In this post, we'll explore this topic in-depth and evaluate two prevalent methods: using conditional checks versus try-except blocks.
The Problem: Checking for Keys in Nested Dictionaries
Consider a scenario in Python where you have a nested dictionary and need to access certain values safely. For instance, let's say you have a dictionary a that may or may not contain the keys 'x' and 'y'. Attempting to directly access a['x']['y'] can lead to KeyError exceptions if those keys do not exist. Here’s a typical example:
[[See Video to Reveal this Text or Code Snippet]]
In the code above, we first check if the keys exist, and only then do we access them safely. While this approach works well, another method is to use a try-except block:
[[See Video to Reveal this Text or Code Snippet]]
However, this raises the question: Is the try-except block a poor practice?
Analyzing the Approaches
Method 1: Using Conditional Checks
The first method, where we check for the existence of keys, is clear and explicit. It enhances code readability and makes it straightforward for other developers (or yourself in the future) to understand the logic at play. Some advantages include:
Readability: It’s easy to see what conditions must be met to proceed.
Error Handling: By checking the keys, we avoid potentially hiding bugs with a broad exception catch.
Method 2: Using Try-Except Blocks
On the other hand, using a try-except block as shown in the second approach can be seen as less explicit. While it can sometimes lead to quicker code (especially when you're not sure about the structure of your data), it also has notable drawbacks:
Hides Errors: If some unexpected error occurs in the try block (not specifically a KeyError), it will be caught and ignored, possibly leading to more significant issues later.
Poor Clarity: It can be less obvious what part of the code could fail without explicitly checking conditions.
The Recommended Approach: Using get()
Python provides a more succinct and readable method to safely access nested dictionary values using the .get() method. This enables you to specify a default value if the key doesn’t exist, while also allowing for chained access to subkeys in a single line. Here’s how you can implement it:
[[See Video to Reveal this Text or Code Snippet]]
Reasons to Prefer .get()
Conciseness: This approach allows for cleaner, easier to read code without multiple checks.
Safety: It avoids raising an exception if the key isn’t found and simply returns None or an empty object instead.
Functional: Makes use of Python's capabilities, adhering to the language's philosophy of simplicity and elegance.
Conclusion: Finding the Right Balance
When working with nested dictionaries in Python, always weigh the options between clarity and brevity. While both the conditional checks and try-except blocks have their places, the .get() method often strikes the right balance. It allows accessing nested values gracefully and safely.
In summary, if you're transitioning to Python from another language, embrace Pythonic ways of handling data, and utilize methods like .get() to keep your code clean and free from unnecessary complexity.
Remember, clear and maintainable code should always be a priority!