filmov
tv
Unity Parallax Tutorial - How to infinite scrolling background
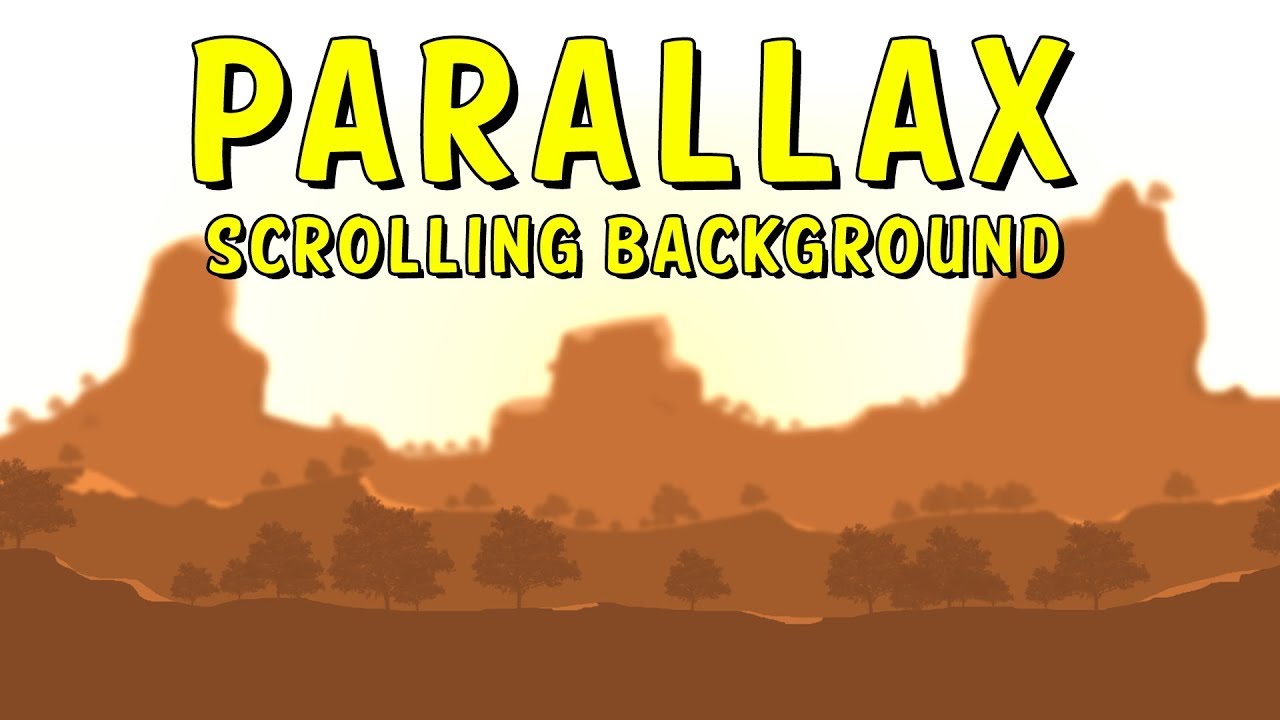
Показать описание
A quick tutorial on how to achieve a cool parallax effect with infinite scrolling. This method uses a simple script, which means you don't need to mess with the z-axis. Personally, I think this is much better for a 2D game, and couldn't find any easy tutorials on it, so figured I would make my own. Go to my discord to download the tutorial resources!
//about
Hey! If you're new here, welcome! I'm an indie game developer, currently working on a physics based game using Unity. This is my devlog / devblog where I show off the progress I make on it. I'll try uploading every week, but I'm pretty busy with university and part time job, so I won't have time every week. But I'll do my best!
#UnityTutorial #unity #gamedev #indiegame #gamedevelopment #unity3d #madewithunity #indiedev #unity2d
//about
Hey! If you're new here, welcome! I'm an indie game developer, currently working on a physics based game using Unity. This is my devlog / devblog where I show off the progress I make on it. I'll try uploading every week, but I'm pretty busy with university and part time job, so I won't have time every week. But I'll do my best!
#UnityTutorial #unity #gamedev #indiegame #gamedevelopment #unity3d #madewithunity #indiedev #unity2d
Unity Parallax Tutorial - How to infinite scrolling background
Unity 2D Parallax Background Effect in 100 Seconds
Unity 2D PARALLAX EFFECT Tutorial | Endless Scrolling Background
Background Types (and why Parallax is important)
The Perfect Pixel Art Parallax Tutorial [and Unity script!]
Infinite Parallax Scrolling Background - Unity 2D Complete Tutorial
Scrolling & Parallax ( Unity 2D ) - Game Mechanics - Unity 3D
Parallax Effect in Unity 2021
Unity 2D Parallax Tutorial
PARALLAX & INFINITE BACKGROUND IN UNITY 🎮 | Create A Endless Background In Unity | Unity Tutoria...
Parallax Infinite Scrolling Background in Unity
Unity parallax tutorial - fast and easy space scene
Unity 3D | Tutorial | Infinite vertical parallax scrolling background
Unity Beginners - How to create Parallax Scrolling
Simple Parallax in Unity (with Tilemaps)
The Secret of Parallax | Unity 2D Platformer Tutorial for Beginners
How to make Parallax Backgrounds (Pixel Art Tutorial)
How to create simple Parallax Scrolling Background effect in 2D Unity game. Easy tutorial.
Scrolling Background in 90 seconds - Unity Tutorial
How to make a parallax background effect in Unity 2D platformer game | Very simple Unity 2D tutorial
Unity Parallax Background - 07 Vertical Movement & Randomized Start
Create Parallax Fog and Dust Particles | Unity Tutorial
Parallax Effect in Unity Tutorial - Infinite Scrolling Background
Unity Parallax Background - 01 Intro & Project Setup
Комментарии