filmov
tv
Efficiently Converting Lists to Maps Using Streams in Java
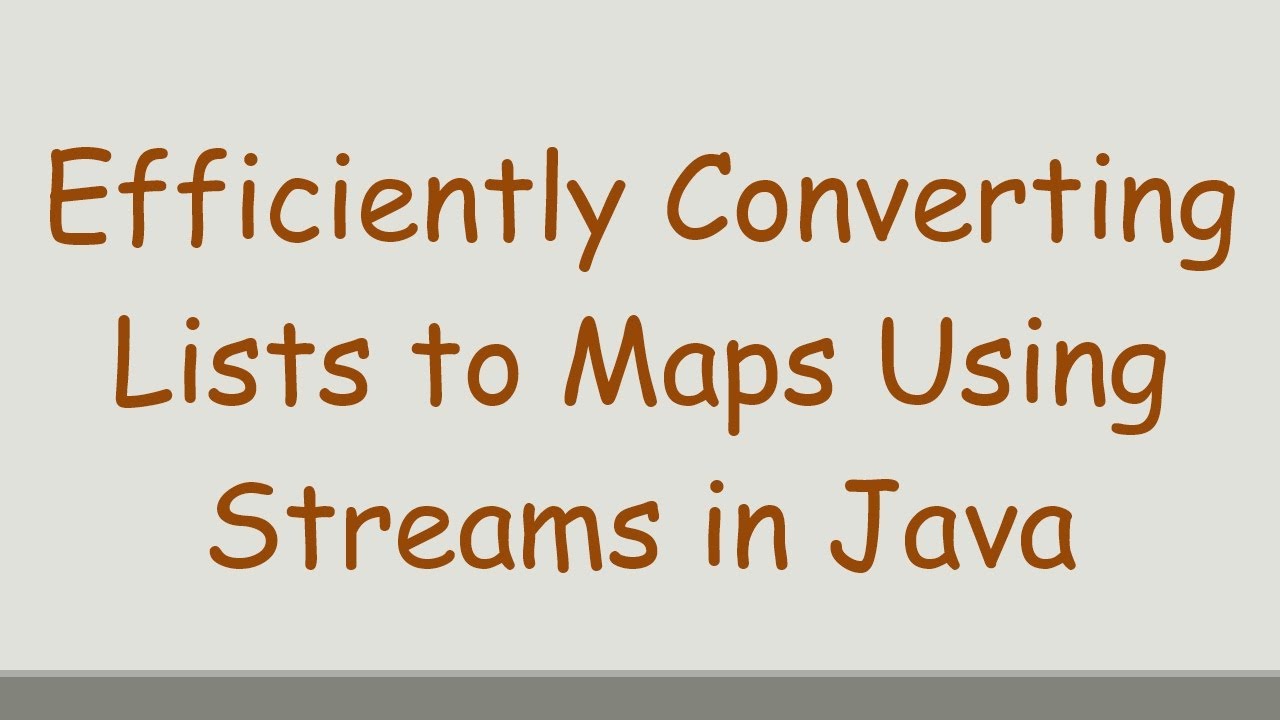
Показать описание
Explore step-by-step instructions on how to convert a list to a map using Java Streams. Enhance your Java development skills with comprehensive examples and explanations.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Efficiently Converting Lists to Maps Using Streams in Java
With Java 8's introduction of streams, developers have acquired powerful tools to handle collections with functional-style operations. One common task is converting a list to a map, which can be crucial for indexing elements or restructuring data for more efficient access. This guide will explore how to perform such conversions effectively using Java Streams.
Understanding the Basics
Before diving into the implementation, let’s briefly revisit Java Streams and the Map interface.
What are Streams?
Java Streams provide a high-level abstraction for processing sequences of elements. They allow you to perform operations like filtering, mapping, and reducing in a declarative manner.
What is a Map?
A Map is a collection of key-value pairs, where each key maps to a single value. The Map interface is part of Java's Collections Framework and is a popular data structure for efficiently indexed lookups.
Converting List to Map Using Streams
Step-by-Step Guide
Step 1: Define Your Data
Assume you have a list of objects, say, Person, where each person has an id and a name. Here’s a simple Person class:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Create a List of Persons
Create a list containing instances of the Person class:
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Import Necessary Packages
Ensure you have the following imports:
[[See Video to Reveal this Text or Code Snippet]]
Step 4: Using Streams to Convert List to Map
[[See Video to Reveal this Text or Code Snippet]]
In this example, Person::getId is used as the key, and Person::getName is used as the value.
Handling Duplicate Keys
If your list contains elements with duplicate keys, you need to handle such instances to avoid runtime exceptions. Here’s an improved version that resolves conflicts by keeping the last occurrence:
[[See Video to Reveal this Text or Code Snippet]]
Full Example
Here’s the complete code for converting a list of Person objects to a map:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By mastering this technique, you'll be better equipped to manage data transformations in your Java applications, paving the way to more efficient and maintainable codebases.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Efficiently Converting Lists to Maps Using Streams in Java
With Java 8's introduction of streams, developers have acquired powerful tools to handle collections with functional-style operations. One common task is converting a list to a map, which can be crucial for indexing elements or restructuring data for more efficient access. This guide will explore how to perform such conversions effectively using Java Streams.
Understanding the Basics
Before diving into the implementation, let’s briefly revisit Java Streams and the Map interface.
What are Streams?
Java Streams provide a high-level abstraction for processing sequences of elements. They allow you to perform operations like filtering, mapping, and reducing in a declarative manner.
What is a Map?
A Map is a collection of key-value pairs, where each key maps to a single value. The Map interface is part of Java's Collections Framework and is a popular data structure for efficiently indexed lookups.
Converting List to Map Using Streams
Step-by-Step Guide
Step 1: Define Your Data
Assume you have a list of objects, say, Person, where each person has an id and a name. Here’s a simple Person class:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Create a List of Persons
Create a list containing instances of the Person class:
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Import Necessary Packages
Ensure you have the following imports:
[[See Video to Reveal this Text or Code Snippet]]
Step 4: Using Streams to Convert List to Map
[[See Video to Reveal this Text or Code Snippet]]
In this example, Person::getId is used as the key, and Person::getName is used as the value.
Handling Duplicate Keys
If your list contains elements with duplicate keys, you need to handle such instances to avoid runtime exceptions. Here’s an improved version that resolves conflicts by keeping the last occurrence:
[[See Video to Reveal this Text or Code Snippet]]
Full Example
Here’s the complete code for converting a list of Person objects to a map:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By mastering this technique, you'll be better equipped to manage data transformations in your Java applications, paving the way to more efficient and maintainable codebases.