filmov
tv
Converting a Java List to a Reactor Flux for Efficient Batch Mapping in Spring Boot
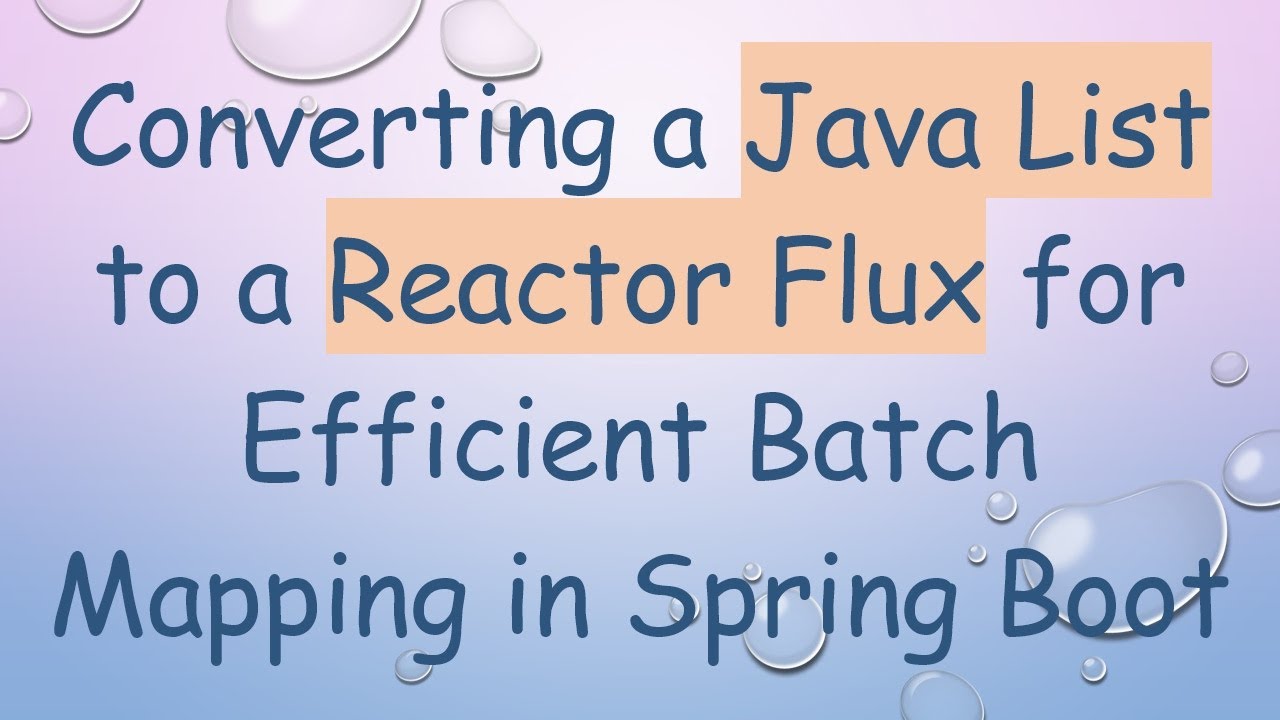
Показать описание
Learn how to effectively convert a Java List to a Reactor Flux, and create an identity mapping between Books and Authors using Spring Boot's BatchMapping.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Java List to Reactor Flux to Identity Map
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Converting a Java List to Reactor Flux: Efficient Mapping in Spring Boot GraphQL
When working with frameworks like Spring Boot and GraphQL, you may encounter various scenarios that challenge your understanding of reactive programming. One such common challenge is transforming a list of Book objects into a map of their respective Author objects using Reactor's Flux and Mono.
The Problem: Mapping Books to Authors
In a typical Spring Boot application, you might find yourself needing to map a list of books, each associated with an author, to a result that makes this relationship clear. The goal here is to create a Mono<Map<Book, Author>>, where each Book serves as a key and the corresponding Author as its value.
To articulate the problem clearly:
Each Book has an authorId.
Multiple Books can share the same Author.
The requirement is to retrieve a map where every book is matched to its respective author.
Here's a small code snippet illustrating your scenario:
[[See Video to Reveal this Text or Code Snippet]]
In this case, the goal is to effectively handle the mapping so that every Author is correctly associated with their Books.
The Solution: Utilizing Flux and Mono
To achieve this mapping outcome, you can employ the collectList() operator from Project Reactor. This transforms the retrieved authors from the repository into a list. Here’s how you can implement it:
Step 1: Collect Authors into a List
First, gather the authors based on their IDs:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Create an Author Map
Next, you can utilize map() to convert the List<Author> into a Map<String, Author>—where the keys are the author IDs, making it easier to look up authors by their IDs:
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Build the Final Map
Once you have the authorIdMap, you can then map each Book to its corresponding Author using the authorIdMap:
[[See Video to Reveal this Text or Code Snippet]]
Key Takeaways
Use collectList() to transform and work with lists instead of streams directly.
Utilize collectMap() for easier access to Author objects using their IDs.
Use flatMap() when needing a reactive stream as the result.
Conclusion
By leveraging Reactor's Flux and Mono, along with the various collection methods, you can efficiently manage and manipulate data mappings in a Spring Boot GraphQL application. This approach not only simplifies the mapping process but also aligns with reactive programming principles, making your code cleaner and more maintainable.
With these guidelines, you should be well-equipped to tackle similar challenges in your projects!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Java List to Reactor Flux to Identity Map
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Converting a Java List to Reactor Flux: Efficient Mapping in Spring Boot GraphQL
When working with frameworks like Spring Boot and GraphQL, you may encounter various scenarios that challenge your understanding of reactive programming. One such common challenge is transforming a list of Book objects into a map of their respective Author objects using Reactor's Flux and Mono.
The Problem: Mapping Books to Authors
In a typical Spring Boot application, you might find yourself needing to map a list of books, each associated with an author, to a result that makes this relationship clear. The goal here is to create a Mono<Map<Book, Author>>, where each Book serves as a key and the corresponding Author as its value.
To articulate the problem clearly:
Each Book has an authorId.
Multiple Books can share the same Author.
The requirement is to retrieve a map where every book is matched to its respective author.
Here's a small code snippet illustrating your scenario:
[[See Video to Reveal this Text or Code Snippet]]
In this case, the goal is to effectively handle the mapping so that every Author is correctly associated with their Books.
The Solution: Utilizing Flux and Mono
To achieve this mapping outcome, you can employ the collectList() operator from Project Reactor. This transforms the retrieved authors from the repository into a list. Here’s how you can implement it:
Step 1: Collect Authors into a List
First, gather the authors based on their IDs:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Create an Author Map
Next, you can utilize map() to convert the List<Author> into a Map<String, Author>—where the keys are the author IDs, making it easier to look up authors by their IDs:
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Build the Final Map
Once you have the authorIdMap, you can then map each Book to its corresponding Author using the authorIdMap:
[[See Video to Reveal this Text or Code Snippet]]
Key Takeaways
Use collectList() to transform and work with lists instead of streams directly.
Utilize collectMap() for easier access to Author objects using their IDs.
Use flatMap() when needing a reactive stream as the result.
Conclusion
By leveraging Reactor's Flux and Mono, along with the various collection methods, you can efficiently manage and manipulate data mappings in a Spring Boot GraphQL application. This approach not only simplifies the mapping process but also aligns with reactive programming principles, making your code cleaner and more maintainable.
With these guidelines, you should be well-equipped to tackle similar challenges in your projects!