filmov
tv
Binary Heaps (Min/Max Heaps) in JavaScript For Beginners An Implementation of a Priority Queue
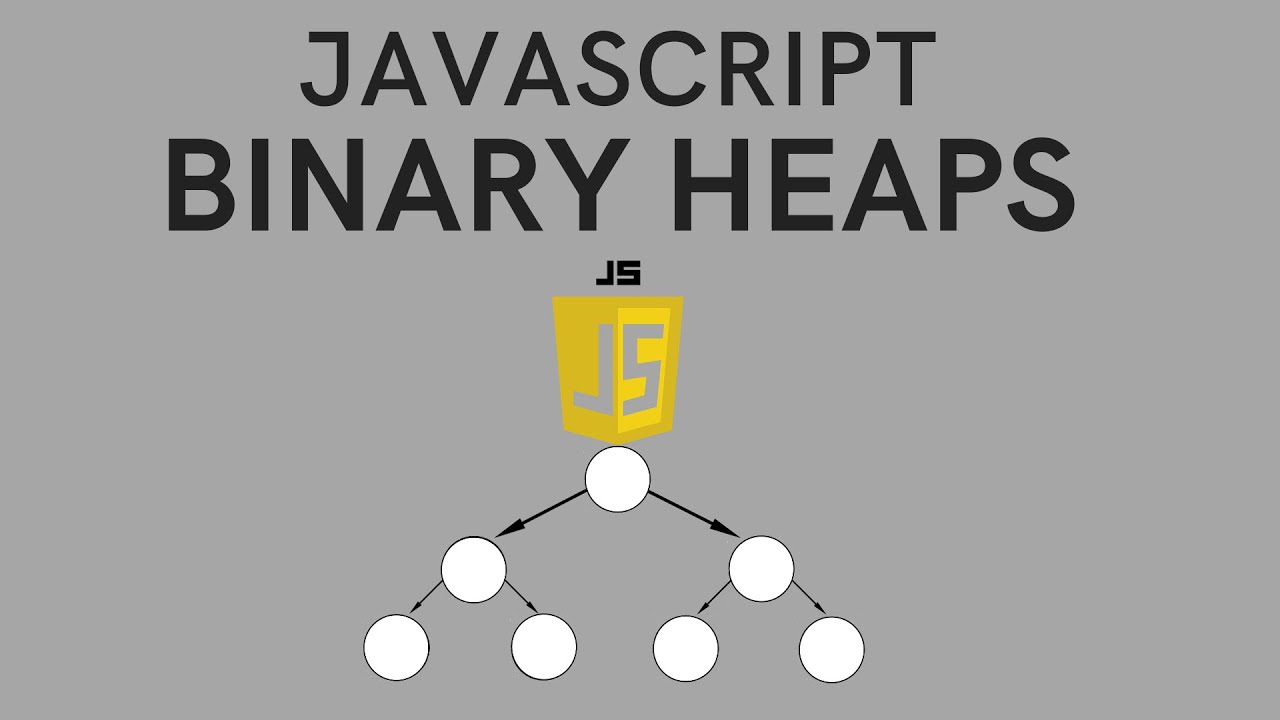
Показать описание
In this tutorial, we will be going over a binary heap, using JavaScript. Within this video, we'll be implementing a binary heap using an array. We'll be going over what a Binary Heap is, as well as going over the Iterative Implementation as well as a Recursive Implementation. A Binary Heap comes in 2 forms, we have a Min-Heap and a Max-Heap. Within this video we'll be going over a Min-Heap. Once you understand how to implement a Min-Heap, you should be able to implement a Max-Heap on your own. A Binary Heap is also an Implementation of the Abstract Data Structure of a Priority Queue.
Source Code:
0:00 : Intro
0:36 : Binary Heap Properties
2:10 : Insertion Overview
4:29 : Deletion Overview
6:00 : Representing Heap using Array Overview
7:57 Min-Heap Constructor Code
8:23 Helper Methods Code
11:07 insert method code (iterative)
11:40 heapifyUp method code (iterative)
12:18 Insertion Code Example Iterative
14:30 insert method code (recursive)
14:53 heapifyUp method code (recursive)
15:37 Insertion Code Example (recursive)
19:03 removeMin method code (iterative)
19:50 heapifyDown method code (iterative)
20:58 Deletion Code Example (iterative)
24:55 removeMin method code (recursive)
25:17 heapifyDown method code (recursive)
26:43 Deletion Code Example (recursive)
Source Code:
0:00 : Intro
0:36 : Binary Heap Properties
2:10 : Insertion Overview
4:29 : Deletion Overview
6:00 : Representing Heap using Array Overview
7:57 Min-Heap Constructor Code
8:23 Helper Methods Code
11:07 insert method code (iterative)
11:40 heapifyUp method code (iterative)
12:18 Insertion Code Example Iterative
14:30 insert method code (recursive)
14:53 heapifyUp method code (recursive)
15:37 Insertion Code Example (recursive)
19:03 removeMin method code (iterative)
19:50 heapifyDown method code (iterative)
20:58 Deletion Code Example (iterative)
24:55 removeMin method code (recursive)
25:17 heapifyDown method code (recursive)
26:43 Deletion Code Example (recursive)
Комментарии