filmov
tv
Converting Roman Numerals to Integer in Java
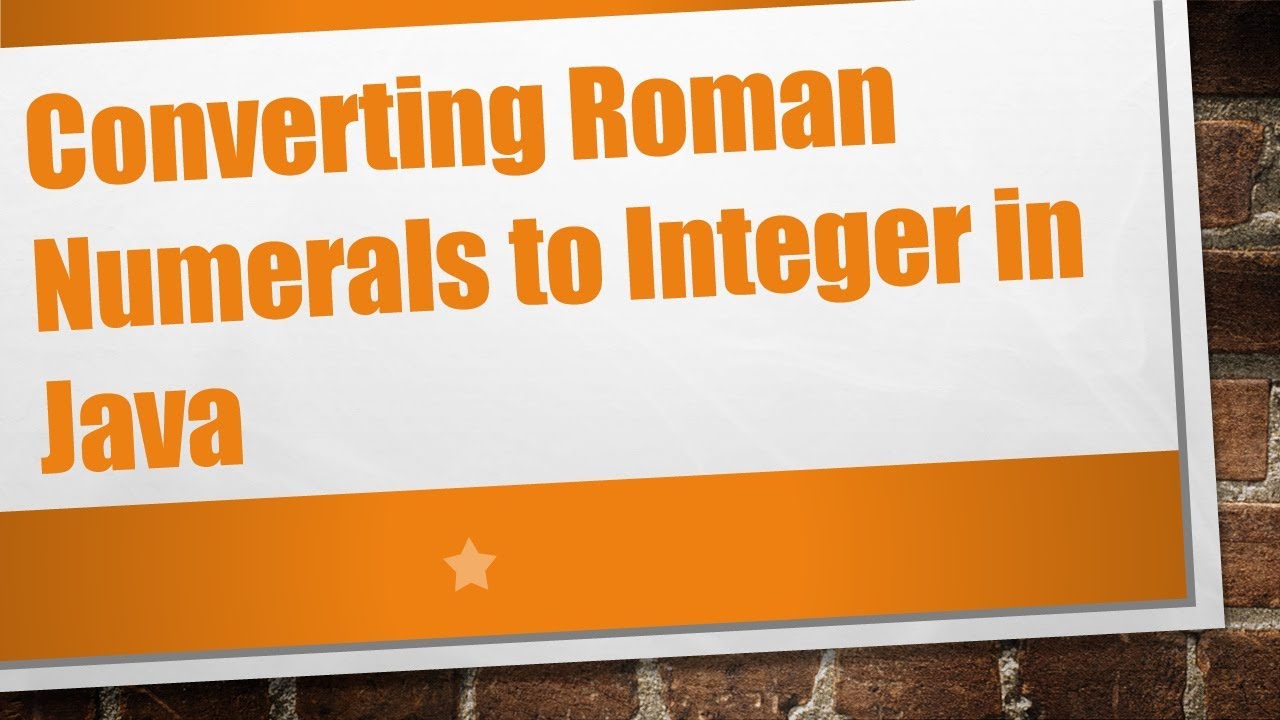
Показать описание
Learn how to convert Roman numerals to integers in Java with step-by-step instructions. This guide covers the process of writing a Java program to convert Roman numerals to their corresponding integer values, providing clarity and understanding for developers.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Roman numerals have a rich historical significance and are still occasionally used today, especially in certain contexts like numbering movie sequels or representing chapters in books. However, when it comes to programming, dealing with Roman numerals might require conversion to more conventional numerical representations. In Java, you can accomplish this conversion through a systematic approach. Let's delve into the process step by step:
Step 1: Define the Roman Numeral to Integer Mapping
To convert Roman numerals to integers, you first need to define a mapping between the individual Roman numeral characters and their corresponding integer values. Here's a typical mapping:
'I' -> 1
'V' -> 5
'X' -> 10
'L' -> 50
'C' -> 100
'D' -> 500
'M' -> 1000
Step 2: Iterate through the Roman Numeral String
Next, you'll iterate through the Roman numeral string character by character. You'll compare each character with its successor to determine if it represents a subtraction or addition.
Step 3: Calculate the Integer Value
During the iteration, you'll compare each character with its successor. If the value of the current character is less than the value of the next character, it indicates subtraction. Otherwise, it indicates addition.
Step 4: Handle Subtraction Cases
When subtraction occurs, you'll subtract the value of the current character from the value of the next character and add this result to the total integer value.
Step 5: Handle Addition Cases
For addition, you'll simply add the value of the current character to the total integer value.
Step 6: Return the Final Integer Value
Once you've iterated through the entire Roman numeral string, you'll have calculated the corresponding integer value. Return this value as the result.
Implementation Example
Here's a sample Java code snippet implementing the above steps:
[[See Video to Reveal this Text or Code Snippet]]
This Java program defines a RomanToIntegerConverter class with a romanToInt method to convert Roman numerals to integers. The main method demonstrates how to use this class to convert a Roman numeral string to its corresponding integer value.
By following these steps and utilizing the provided code example, you can efficiently convert Roman numerals to integers in Java, enabling seamless integration of historical number representations into modern programming contexts.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Roman numerals have a rich historical significance and are still occasionally used today, especially in certain contexts like numbering movie sequels or representing chapters in books. However, when it comes to programming, dealing with Roman numerals might require conversion to more conventional numerical representations. In Java, you can accomplish this conversion through a systematic approach. Let's delve into the process step by step:
Step 1: Define the Roman Numeral to Integer Mapping
To convert Roman numerals to integers, you first need to define a mapping between the individual Roman numeral characters and their corresponding integer values. Here's a typical mapping:
'I' -> 1
'V' -> 5
'X' -> 10
'L' -> 50
'C' -> 100
'D' -> 500
'M' -> 1000
Step 2: Iterate through the Roman Numeral String
Next, you'll iterate through the Roman numeral string character by character. You'll compare each character with its successor to determine if it represents a subtraction or addition.
Step 3: Calculate the Integer Value
During the iteration, you'll compare each character with its successor. If the value of the current character is less than the value of the next character, it indicates subtraction. Otherwise, it indicates addition.
Step 4: Handle Subtraction Cases
When subtraction occurs, you'll subtract the value of the current character from the value of the next character and add this result to the total integer value.
Step 5: Handle Addition Cases
For addition, you'll simply add the value of the current character to the total integer value.
Step 6: Return the Final Integer Value
Once you've iterated through the entire Roman numeral string, you'll have calculated the corresponding integer value. Return this value as the result.
Implementation Example
Here's a sample Java code snippet implementing the above steps:
[[See Video to Reveal this Text or Code Snippet]]
This Java program defines a RomanToIntegerConverter class with a romanToInt method to convert Roman numerals to integers. The main method demonstrates how to use this class to convert a Roman numeral string to its corresponding integer value.
By following these steps and utilizing the provided code example, you can efficiently convert Roman numerals to integers in Java, enabling seamless integration of historical number representations into modern programming contexts.