filmov
tv
Coding Math: Episode 13 - Friction
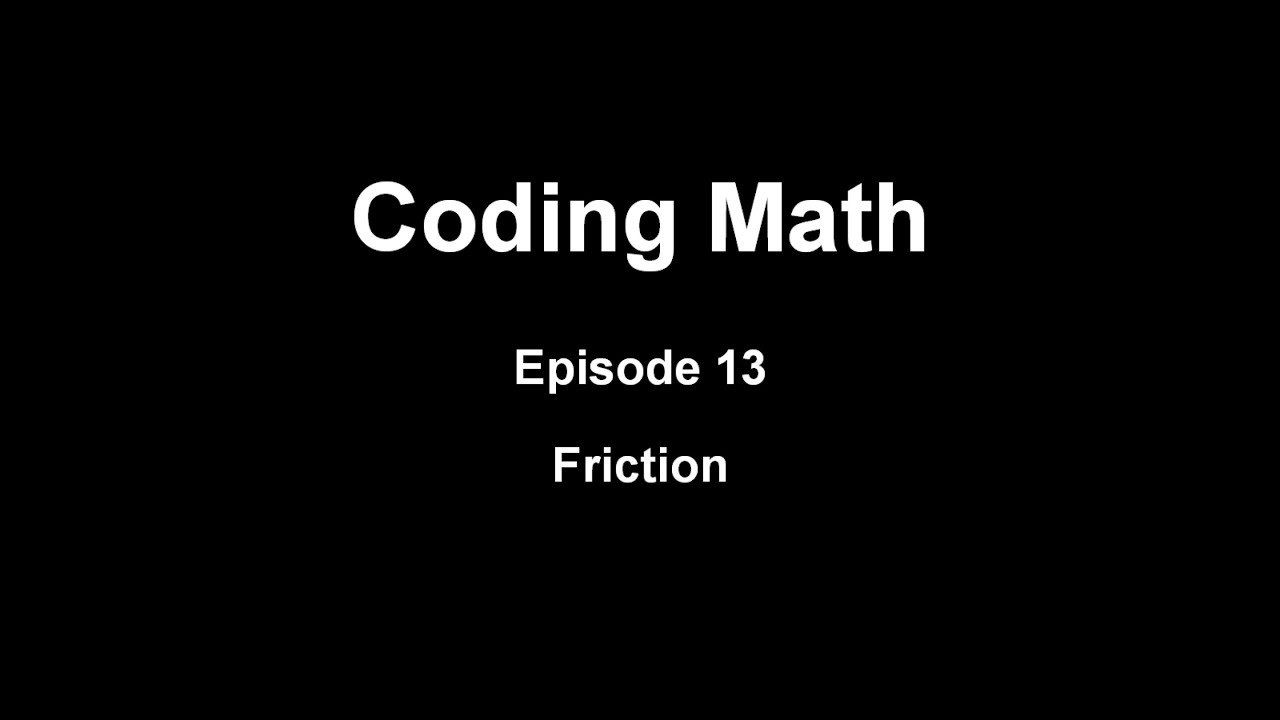
Показать описание
Continuing the basic physics for programmers series, this time we look at friction, two ways to accomplish it, one right, one wrong, and the pros and cons of each.
Coding Math: Episode 13 - Friction
Coding Math: Episode 14 - Collision Detection
Coding Math: Episode 12 - Edge Handling
Coding Math: Episode 15 - Springs Part 1
Genius IQ Test math puzzle🔥
Coding Math: Episode 16 - Springs Part 2
Math is easy - POPPY PLAYTIME CHAPTER 3 | AUSTRIAN ANIMATION
Math Shorts Episode 13 - Long Division
Day 13: Claw Contraption (Advent of Code 2024, Haskell)
Convert Hindu Arabic to Roman Numerals/Roman Number Trick #math #shorts #artikipathshala #shortsfeed
Amazing Math Hacks
Speed Mental Math Exercise #shorts
Coding Math: Episode 26 - 2D and 3D Coordinate Rotation
The Most Wholesome Math Equation
Genius math hack 🗒️ #shorts
This Made Me Fail 5th Grade Math!
Math is so easy | GARTEN OF BANBAN | GH'S ANIMATION
Nastya and dad play with cat
MATH GENIUS - POPPY PLAYTIME CHAPTER 3 | GH'S ANIMATION
Coding Math: Application 1 - Ballistics Part 1
#shorts || area formulas✍️ || Basic Math formula || #reet #ssc #maths #education #viral #math
The Hardest Math Test
Coding Math: Episode 17 - Particles - Optimization
Coding Dojo JavaScript (Episode 13) — for...of, Math.max, filter()
Комментарии