filmov
tv
Authorization in ASP NET Core
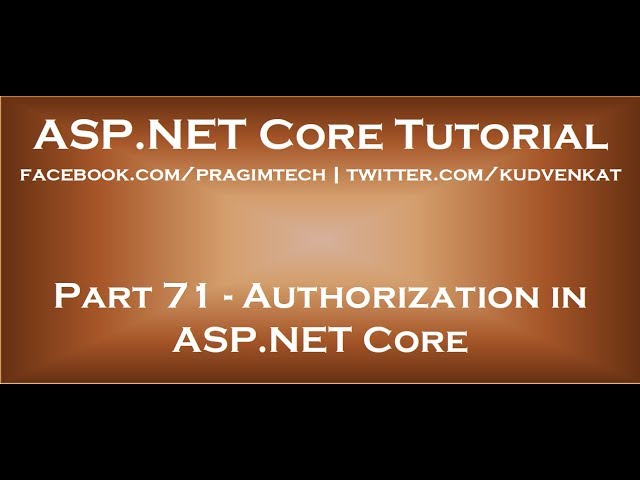
Показать описание
In this video we will discuss, authorization in ASP.NET Core.
Text version of the video
Healthy diet is very important for both body and mind. We want to inspire you to cook and eat healthy. If you like Aarvi Kitchen recipes, please support by sharing, subscribing and liking.
Slides
ASP.NET Core Text Articles & Slides
ASP.NET Core Tutorial
Angular, JavaScript, jQuery, Dot Net & SQL Playlists
What is Authorization in ASP.NET Core
Authentication is the process of identifying who the user is.
Authorization is the process of identifying what the user can and cannot do.
For example, if the logged in user is an administrator he may be able to Create, Read, Update and Delete orders, where as a normal user may only view orders but not Create, Update or Delete orders.
Authorization in ASP.NET Core MVC is controlled through the AuthorizeAttribute
Authorize Attribute in ASP.NET Core
When the Authorize Attribute is used in it's simplest form, without any parameters, it only checks if the user is authenticated.
Authorize Attribute Example
As the Authorize attribute is applied on the Controller, it is applicable to all the action methods in the controller. The user must be logged in, to access any of the controller action methods.
[Authorize]
public class HomeController : Controller
{
public ViewResult Details(int? id)
{
}
public ViewResult Create()
{
}
public ViewResult Edit(int id)
{
}
}
Authorize attribute can be applied on individual action methods as well. In the example below, only the Details action method is protected from anonymous access.
public class HomeController : Controller
{
[Authorize]
public ViewResult Details(int? id)
{
}
public ViewResult Create()
{
}
public ViewResult Edit(int id)
{
}
}
AllowAnonymous Attribute in ASP.NET Core
As the name implies, AllowAnonymous attribute allows anonymous access. We generally use this attribute in combination with the Authorize attribute.
AllowAnonymous Attribute Example
As the Authorize attribute is applied at the controller level, all the action methods in the controller are protected from anonymous access. However, since the Details action methos is decorated with AllowAnonymous attribute, it will be allowed anonymous access.
[Authorize]
public class HomeController : Controller
{
[AllowAnonymous]
public ViewResult Details(int? id)
{
}
public ViewResult Create()
{
}
public ViewResult Edit(int id)
{
}
}
Please note: If you apply [AllowAnonymous] attribute at the controller level, any [Authorize] attribute attributes on the same controller (or on any action within it) is ignored.
Apply Authorize attribute globally
To apply [Authorize] attribute globally on all controlls and controller actions throught your application modify the code in ConfigureServices method of the Startup class.
public void ConfigureServices(IServiceCollection services)
{
// Other Code
services.AddMvc(config =] {
var policy = new AuthorizationPolicyBuilder()
.RequireAuthenticatedUser()
.Build();
config.Filters.Add(new AuthorizeFilter(policy));
});
// Other Code
}
AuthorizationPolicyBuilder is in Microsoft.AspNetCore.Authorization namespace
AuthorizeFilter is in Microsoft.AspNetCore.Mvc.Authorization namespace
If you do not have [AllowAnonymous] attribute on the Login actions of the account controller you will get the following error because the application is stuck in an infinite loop.
HTTP Error 404.15 - Not Found
The request filtering module is configured to deny a request where the query string is too long.
Most likely causes:
Request filtering is configured on the Web server to deny the request because the query string is too long.
You try to access /Account/login
Since the [Authorize] attribute is applied globally, you cannot access the URL /Account/login
To login you have to go to /Account/login
So the application is stuck in this infinite loop and every time we are redirected, the query string ?ReturnUrl=/Account/Login is appended to the URL
This is the reason we get the error - Web server denied the request because the query string is too long.
To fix this error, decorate Login() actions in the AccountController with [AllowAnonymous] attribute.
Text version of the video
Healthy diet is very important for both body and mind. We want to inspire you to cook and eat healthy. If you like Aarvi Kitchen recipes, please support by sharing, subscribing and liking.
Slides
ASP.NET Core Text Articles & Slides
ASP.NET Core Tutorial
Angular, JavaScript, jQuery, Dot Net & SQL Playlists
What is Authorization in ASP.NET Core
Authentication is the process of identifying who the user is.
Authorization is the process of identifying what the user can and cannot do.
For example, if the logged in user is an administrator he may be able to Create, Read, Update and Delete orders, where as a normal user may only view orders but not Create, Update or Delete orders.
Authorization in ASP.NET Core MVC is controlled through the AuthorizeAttribute
Authorize Attribute in ASP.NET Core
When the Authorize Attribute is used in it's simplest form, without any parameters, it only checks if the user is authenticated.
Authorize Attribute Example
As the Authorize attribute is applied on the Controller, it is applicable to all the action methods in the controller. The user must be logged in, to access any of the controller action methods.
[Authorize]
public class HomeController : Controller
{
public ViewResult Details(int? id)
{
}
public ViewResult Create()
{
}
public ViewResult Edit(int id)
{
}
}
Authorize attribute can be applied on individual action methods as well. In the example below, only the Details action method is protected from anonymous access.
public class HomeController : Controller
{
[Authorize]
public ViewResult Details(int? id)
{
}
public ViewResult Create()
{
}
public ViewResult Edit(int id)
{
}
}
AllowAnonymous Attribute in ASP.NET Core
As the name implies, AllowAnonymous attribute allows anonymous access. We generally use this attribute in combination with the Authorize attribute.
AllowAnonymous Attribute Example
As the Authorize attribute is applied at the controller level, all the action methods in the controller are protected from anonymous access. However, since the Details action methos is decorated with AllowAnonymous attribute, it will be allowed anonymous access.
[Authorize]
public class HomeController : Controller
{
[AllowAnonymous]
public ViewResult Details(int? id)
{
}
public ViewResult Create()
{
}
public ViewResult Edit(int id)
{
}
}
Please note: If you apply [AllowAnonymous] attribute at the controller level, any [Authorize] attribute attributes on the same controller (or on any action within it) is ignored.
Apply Authorize attribute globally
To apply [Authorize] attribute globally on all controlls and controller actions throught your application modify the code in ConfigureServices method of the Startup class.
public void ConfigureServices(IServiceCollection services)
{
// Other Code
services.AddMvc(config =] {
var policy = new AuthorizationPolicyBuilder()
.RequireAuthenticatedUser()
.Build();
config.Filters.Add(new AuthorizeFilter(policy));
});
// Other Code
}
AuthorizationPolicyBuilder is in Microsoft.AspNetCore.Authorization namespace
AuthorizeFilter is in Microsoft.AspNetCore.Mvc.Authorization namespace
If you do not have [AllowAnonymous] attribute on the Login actions of the account controller you will get the following error because the application is stuck in an infinite loop.
HTTP Error 404.15 - Not Found
The request filtering module is configured to deny a request where the query string is too long.
Most likely causes:
Request filtering is configured on the Web server to deny the request because the query string is too long.
You try to access /Account/login
Since the [Authorize] attribute is applied globally, you cannot access the URL /Account/login
To login you have to go to /Account/login
So the application is stuck in this infinite loop and every time we are redirected, the query string ?ReturnUrl=/Account/Login is appended to the URL
This is the reason we get the error - Web server denied the request because the query string is too long.
To fix this error, decorate Login() actions in the AccountController with [AllowAnonymous] attribute.
Комментарии