filmov
tv
How to Handle KeyError in Python Functions
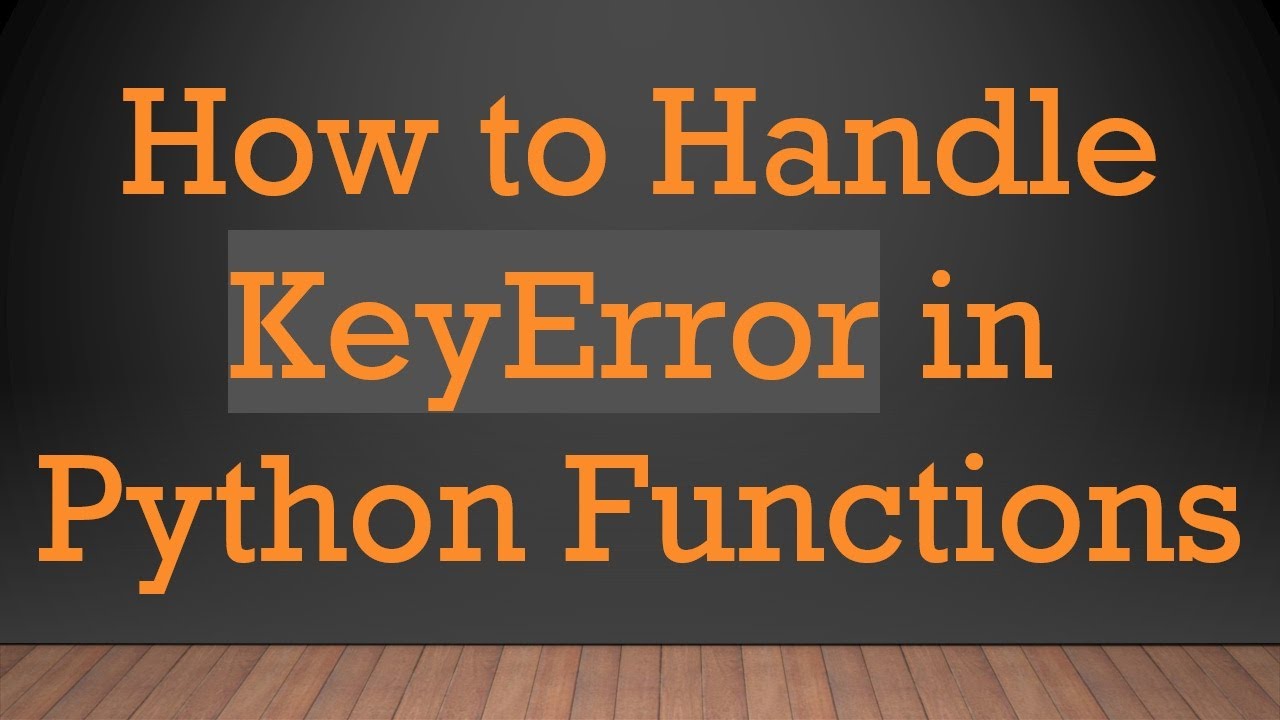
Показать описание
Learn how to pass a dictionary into a function while handling KeyErrors effectively using Python's `get()` method.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How can I pass a dictionary name into a function and handle KeyError inside the function?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Handle KeyError in Python Functions: A Simple Guide
When working with Python dictionaries, running into a KeyError is a common challenge that many developers face. This type of error occurs when you try to access a key that doesn’t exist in the dictionary. But don’t worry! In this guide, we will explore how to pass a name of a dictionary into a function and effectively handle KeyError to avoid interruptions in your program.
The Problem
Consider the following scenario: you have a function for printing specific content based on the keys of a dictionary. You want to call this function with dynamic data but encounter KeyError if the key being accessed does not exist in the dictionary.
Example Code
Here’s a basic outline of what your function might look like:
[[See Video to Reveal this Text or Code Snippet]]
In this case, you're trying to access a particular key from the dictionary slot while iterating through row. But if the key is not present, a KeyError will raise an exception before the function executes.
The Solution
The key to solving this problem is restructuring your function slightly. By using Python's built-in get() method, you can safely attempt to retrieve a value from the dictionary without raising a KeyError. If the key doesn’t exist, you can provide an alternative value to return.
Revised Function
Here is how you can rewrite your function:
[[See Video to Reveal this Text or Code Snippet]]
How it Works
Using get() Method:
The get() method of a dictionary attempts to retrieve the value for a given key. If the key is found, it returns the corresponding value. If not, it returns a specified alternative value instead of throwing an error.
This means when you call print_boardrow, it will look up the given key and print the value or the alternative content:
[[See Video to Reveal this Text or Code Snippet]]
Code Explanation
Parameters:
row: This is the dictionary passed to the function.
key: This is the key you want to access in the dictionary.
alt_content: This is the content that you want to display if the key isn't found.
Output: If the key exists in the dictionary, it prints the associated value. If the key doesn’t exist, it prints the alternative content you provided.
Benefits
Error-Free Execution: By using the get() method, you eliminate the chance of a KeyError, leading to smoother execution of your program.
Readability: This approach makes your code cleaner and easier to read, which is always a plus when maintaining or sharing code with others.
Conclusion
Handling KeyError in Python functions doesn’t have to be a headache. By restructuring your code to utilize the get() method when accessing dictionary keys, you can ensure your program runs smoothly without unexpected interruptions.
Embrace the elegance of Python, and start incorporating these strategies into your own coding practices!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How can I pass a dictionary name into a function and handle KeyError inside the function?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Handle KeyError in Python Functions: A Simple Guide
When working with Python dictionaries, running into a KeyError is a common challenge that many developers face. This type of error occurs when you try to access a key that doesn’t exist in the dictionary. But don’t worry! In this guide, we will explore how to pass a name of a dictionary into a function and effectively handle KeyError to avoid interruptions in your program.
The Problem
Consider the following scenario: you have a function for printing specific content based on the keys of a dictionary. You want to call this function with dynamic data but encounter KeyError if the key being accessed does not exist in the dictionary.
Example Code
Here’s a basic outline of what your function might look like:
[[See Video to Reveal this Text or Code Snippet]]
In this case, you're trying to access a particular key from the dictionary slot while iterating through row. But if the key is not present, a KeyError will raise an exception before the function executes.
The Solution
The key to solving this problem is restructuring your function slightly. By using Python's built-in get() method, you can safely attempt to retrieve a value from the dictionary without raising a KeyError. If the key doesn’t exist, you can provide an alternative value to return.
Revised Function
Here is how you can rewrite your function:
[[See Video to Reveal this Text or Code Snippet]]
How it Works
Using get() Method:
The get() method of a dictionary attempts to retrieve the value for a given key. If the key is found, it returns the corresponding value. If not, it returns a specified alternative value instead of throwing an error.
This means when you call print_boardrow, it will look up the given key and print the value or the alternative content:
[[See Video to Reveal this Text or Code Snippet]]
Code Explanation
Parameters:
row: This is the dictionary passed to the function.
key: This is the key you want to access in the dictionary.
alt_content: This is the content that you want to display if the key isn't found.
Output: If the key exists in the dictionary, it prints the associated value. If the key doesn’t exist, it prints the alternative content you provided.
Benefits
Error-Free Execution: By using the get() method, you eliminate the chance of a KeyError, leading to smoother execution of your program.
Readability: This approach makes your code cleaner and easier to read, which is always a plus when maintaining or sharing code with others.
Conclusion
Handling KeyError in Python functions doesn’t have to be a headache. By restructuring your code to utilize the get() method when accessing dictionary keys, you can ensure your program runs smoothly without unexpected interruptions.
Embrace the elegance of Python, and start incorporating these strategies into your own coding practices!