filmov
tv
How to Handle KeyError in Python with If Statements
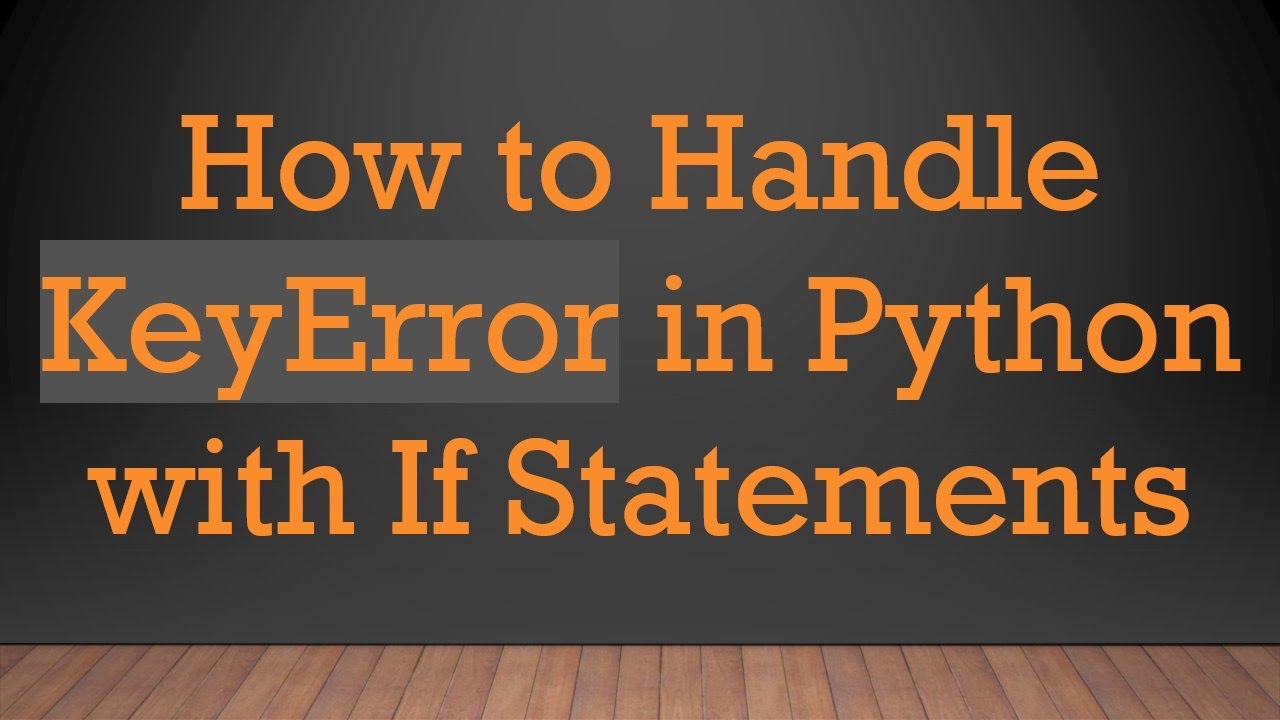
Показать описание
Learn how to handle `KeyError` in Python efficiently using if statements to check key existence and dynamically assign values in dictionaries.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Handle KeyError in Python
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Handling KeyError in Python: A Simple Guide
As a Python programmer, one of the challenges you might face is dealing with KeyError. This error occurs when you attempt to access a dictionary key that does not exist. In this article, we'll explore how to elegantly handle KeyError using conditional statements (if's) to ensure that your code runs smoothly, even when keys are missing. Specifically, we’ll look at a scenario involving a nested dictionary and how to default to a specific value when a key isn't found.
Understanding the Problem
Imagine you have a dictionary called counts_stats that contains various game statistics. You want to extract values related to game modes, specifically for team and solo modes in SPEED_UHC. Here’s a snippet of code that could lead to a KeyError:
[[See Video to Reveal this Text or Code Snippet]]
The challenge arises when the key team_normal does not exist within the nested structure of your dictionary. Instead of letting the program crash with a KeyError, you want to assign a default value if the key is absent.
The Solution: Using if Statements
To handle this situation, you can simply check if the desired key exists before trying to access it. Here's how you can do that:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Solution
Key Checking:
We use an if statement to check if team_normal is present in the dictionary. We can do this by using the in keyword combined with the keys() method.
Conditional Assignment:
If the key does not exist ('team_normal' not in ...), assign a default value of your choice to speed_uhc_team.
If the key exists, proceed to assign the value from the dictionary to speed_uhc_team.
Default Value:
The variable my_choice_value can be any value you decide beforehand. It acts as a fallback solution whenever the key is missing.
Example Usage
Consider the following example where you have a dictionary called counts_stats and you want to fetch the values for both team and solo modes:
[[See Video to Reveal this Text or Code Snippet]]
In the above code:
If team_normal is missing, speed_uhc_team will be set to 0, and you won't encounter a KeyError.
If it exists, you'll retrieve its actual value seamlessly.
Conclusion
Handling KeyError in Python can be straightforward when you check for the existence of keys in dictionaries before accessing them. This practice not only prevents your program from crashing but also allows for dynamic assignment based on the availability of keys. Implementing such checks improves the robustness of your code while making sure you have sensible defaults for missing data.
Adopt these strategies in your Python coding to enhance your error handling, leading to cleaner and more efficient code!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Handle KeyError in Python
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Handling KeyError in Python: A Simple Guide
As a Python programmer, one of the challenges you might face is dealing with KeyError. This error occurs when you attempt to access a dictionary key that does not exist. In this article, we'll explore how to elegantly handle KeyError using conditional statements (if's) to ensure that your code runs smoothly, even when keys are missing. Specifically, we’ll look at a scenario involving a nested dictionary and how to default to a specific value when a key isn't found.
Understanding the Problem
Imagine you have a dictionary called counts_stats that contains various game statistics. You want to extract values related to game modes, specifically for team and solo modes in SPEED_UHC. Here’s a snippet of code that could lead to a KeyError:
[[See Video to Reveal this Text or Code Snippet]]
The challenge arises when the key team_normal does not exist within the nested structure of your dictionary. Instead of letting the program crash with a KeyError, you want to assign a default value if the key is absent.
The Solution: Using if Statements
To handle this situation, you can simply check if the desired key exists before trying to access it. Here's how you can do that:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Solution
Key Checking:
We use an if statement to check if team_normal is present in the dictionary. We can do this by using the in keyword combined with the keys() method.
Conditional Assignment:
If the key does not exist ('team_normal' not in ...), assign a default value of your choice to speed_uhc_team.
If the key exists, proceed to assign the value from the dictionary to speed_uhc_team.
Default Value:
The variable my_choice_value can be any value you decide beforehand. It acts as a fallback solution whenever the key is missing.
Example Usage
Consider the following example where you have a dictionary called counts_stats and you want to fetch the values for both team and solo modes:
[[See Video to Reveal this Text or Code Snippet]]
In the above code:
If team_normal is missing, speed_uhc_team will be set to 0, and you won't encounter a KeyError.
If it exists, you'll retrieve its actual value seamlessly.
Conclusion
Handling KeyError in Python can be straightforward when you check for the existence of keys in dictionaries before accessing them. This practice not only prevents your program from crashing but also allows for dynamic assignment based on the availability of keys. Implementing such checks improves the robustness of your code while making sure you have sensible defaults for missing data.
Adopt these strategies in your Python coding to enhance your error handling, leading to cleaner and more efficient code!