filmov
tv
Adding and Deleting values from ordereddict | Python 4 You | Lecture 274
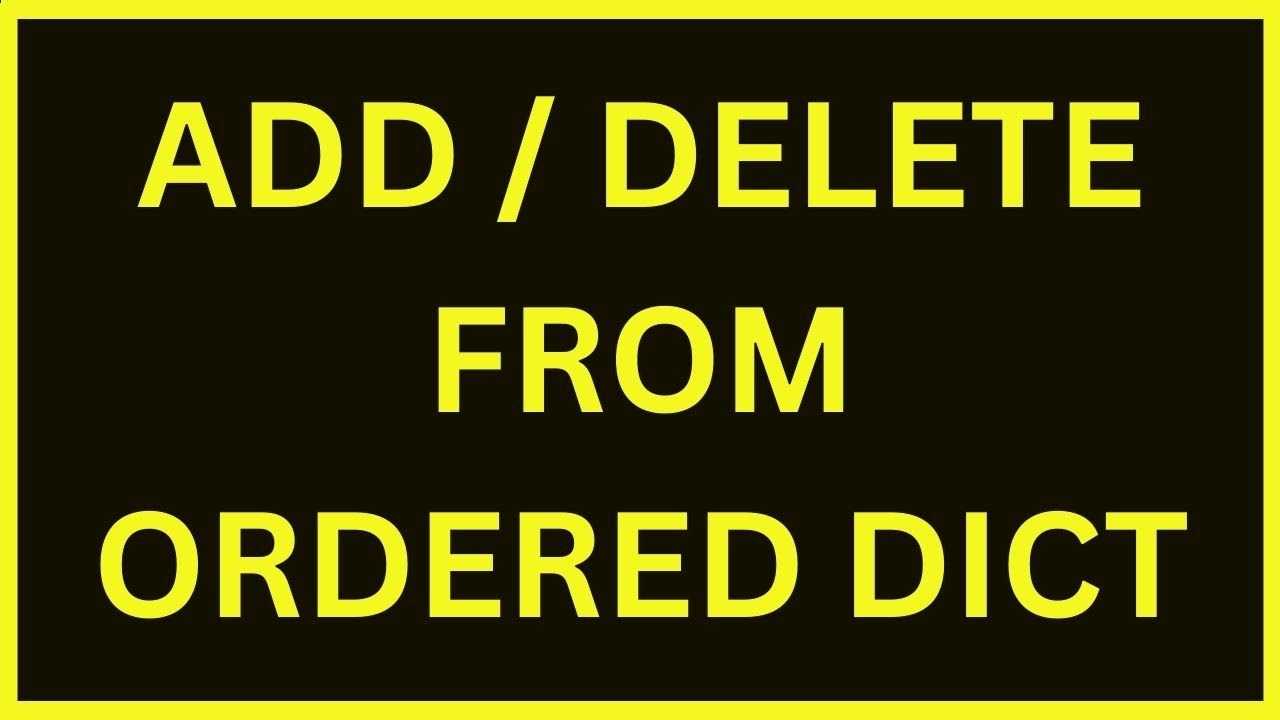
Показать описание
Adding and Deleting Values from Python OrderedDict
Introduction:
Python's OrderedDict, a subclass of the standard dictionary, is a versatile data structure that not only preserves the order of elements but also allows for dynamic modification. In this article, we'll explore the essential techniques for adding and deleting values from an OrderedDict. Whether you're a seasoned developer or a Python enthusiast, mastering these operations can significantly enhance your ability to manage ordered collections.
Adding Values to an OrderedDict:
Adding values to an OrderedDict is a straightforward process, offering flexibility in accommodating new key-value pairs while maintaining the desired order.
Method 1: Using Direct Assignment
You can add a new key-value pair to an OrderedDict by directly assigning a value to a key.
from collections import OrderedDict
# Create an OrderedDict
my_ordered_dict = OrderedDict([('apple', 3), ('banana', 2), ('orange', 1)])
# Add a new value
my_ordered_dict['pear'] = 4
# Display the updated OrderedDict
print(my_ordered_dict)
The output will now include the newly added key-value pair:
OrderedDict([('apple', 3), ('banana', 2), ('orange', 1), ('pear', 4)])
Method 2: Using the update() Method
The update() method allows you to add multiple key-value pairs at once.
# Using the update() method
# Display the updated OrderedDict
print(my_ordered_dict)
The output now reflects the additional entries:
OrderedDict([('apple', 3), ('banana', 2), ('orange', 1), ('pear', 4), ('grape', 5), ('kiwi', 2)])
Deleting Values from an OrderedDict:
Removing values from an OrderedDict is equally important, especially when dealing with dynamic datasets or changing requirements.
Method 1: Using the pop() Method
The pop() method removes a key and its associated value from the OrderedDict. This method also returns the value associated with the removed key.
# Removing 'banana' from the OrderedDict
# Display the updated OrderedDict and the removed value
print(f"Removed value: {removed_value}")
print(my_ordered_dict)
The output shows the removed value and the updated OrderedDict:
Removed value: 2
OrderedDict([('apple', 3), ('orange', 1), ('pear', 4), ('grape', 5), ('kiwi', 2)])
Method 2: Using the popitem() Method
The popitem() method removes and returns the last key-value pair added to the OrderedDict. This method is useful when the order of removal is not critical.
# Removing the last item from the OrderedDict
# Display the updated OrderedDict and the removed item
print(f"Removed item: {removed_item}")
print(my_ordered_dict)
The output displays the removed item and the updated OrderedDict:
Removed item: ('kiwi', 2)
OrderedDict([('apple', 3), ('orange', 1), ('pear', 4), ('grape', 5)])
Practical Considerations:
Maintaining Order:
When adding or deleting values from an OrderedDict, it's crucial to consider the order in which elements are processed. This aligns with the primary purpose of using an OrderedDict.
Error Handling:
Implement error handling to manage scenarios where keys are not present during deletion, ensuring your code remains robust and error-free.
Conclusion:
Fine-tuning the order of elements in an OrderedDict by adding or deleting values is an essential skill for Python developers. Whether you're working on configuration management, data serialization, or any other application where order matters, mastering these operations can greatly enhance the precision and reliability of your code.
As you integrate these techniques into your Python projects, you'll find that the OrderedDict becomes an even more powerful tool for managing ordered collections with ease and flexibility.
People also search for these keywords:
ordered dict, python dict, delete value from a dictionary, key and value in dictionary python, update value of a key from a dictionary, python dictionary key value, value, values, dict in python, delete item from dictionary, pyhon collections ordered dictionary, python dictionary values, how to take dictionary input from user, python dict to json, python dict update, index value, how to delete columns from python dataframe, value of a dictionary
Useful Queries:
Adding and deleting values from ordereddict python
Adding and deleting values from ordereddict example
python ordereddict
ordereddict popitem last=false
convert dict to ordereddict
ordereddict add item
ordereddict vs dict
ordereddict move to end
#python4 #pythontutorial #pythonprogramming #python3 #viral #trending #pythonforbeginners #pythonlectures #pythonprograms #pythonlatest #rehanblogger #python4you #pythonlatestversion #pythonlatestversion Learn python3.12.0 and latest version of python3.13. If you are searching for python3.13.0 lessons, you are at the right place as this course will be very helpful for python learners or python beginners.
Introduction:
Python's OrderedDict, a subclass of the standard dictionary, is a versatile data structure that not only preserves the order of elements but also allows for dynamic modification. In this article, we'll explore the essential techniques for adding and deleting values from an OrderedDict. Whether you're a seasoned developer or a Python enthusiast, mastering these operations can significantly enhance your ability to manage ordered collections.
Adding Values to an OrderedDict:
Adding values to an OrderedDict is a straightforward process, offering flexibility in accommodating new key-value pairs while maintaining the desired order.
Method 1: Using Direct Assignment
You can add a new key-value pair to an OrderedDict by directly assigning a value to a key.
from collections import OrderedDict
# Create an OrderedDict
my_ordered_dict = OrderedDict([('apple', 3), ('banana', 2), ('orange', 1)])
# Add a new value
my_ordered_dict['pear'] = 4
# Display the updated OrderedDict
print(my_ordered_dict)
The output will now include the newly added key-value pair:
OrderedDict([('apple', 3), ('banana', 2), ('orange', 1), ('pear', 4)])
Method 2: Using the update() Method
The update() method allows you to add multiple key-value pairs at once.
# Using the update() method
# Display the updated OrderedDict
print(my_ordered_dict)
The output now reflects the additional entries:
OrderedDict([('apple', 3), ('banana', 2), ('orange', 1), ('pear', 4), ('grape', 5), ('kiwi', 2)])
Deleting Values from an OrderedDict:
Removing values from an OrderedDict is equally important, especially when dealing with dynamic datasets or changing requirements.
Method 1: Using the pop() Method
The pop() method removes a key and its associated value from the OrderedDict. This method also returns the value associated with the removed key.
# Removing 'banana' from the OrderedDict
# Display the updated OrderedDict and the removed value
print(f"Removed value: {removed_value}")
print(my_ordered_dict)
The output shows the removed value and the updated OrderedDict:
Removed value: 2
OrderedDict([('apple', 3), ('orange', 1), ('pear', 4), ('grape', 5), ('kiwi', 2)])
Method 2: Using the popitem() Method
The popitem() method removes and returns the last key-value pair added to the OrderedDict. This method is useful when the order of removal is not critical.
# Removing the last item from the OrderedDict
# Display the updated OrderedDict and the removed item
print(f"Removed item: {removed_item}")
print(my_ordered_dict)
The output displays the removed item and the updated OrderedDict:
Removed item: ('kiwi', 2)
OrderedDict([('apple', 3), ('orange', 1), ('pear', 4), ('grape', 5)])
Practical Considerations:
Maintaining Order:
When adding or deleting values from an OrderedDict, it's crucial to consider the order in which elements are processed. This aligns with the primary purpose of using an OrderedDict.
Error Handling:
Implement error handling to manage scenarios where keys are not present during deletion, ensuring your code remains robust and error-free.
Conclusion:
Fine-tuning the order of elements in an OrderedDict by adding or deleting values is an essential skill for Python developers. Whether you're working on configuration management, data serialization, or any other application where order matters, mastering these operations can greatly enhance the precision and reliability of your code.
As you integrate these techniques into your Python projects, you'll find that the OrderedDict becomes an even more powerful tool for managing ordered collections with ease and flexibility.
People also search for these keywords:
ordered dict, python dict, delete value from a dictionary, key and value in dictionary python, update value of a key from a dictionary, python dictionary key value, value, values, dict in python, delete item from dictionary, pyhon collections ordered dictionary, python dictionary values, how to take dictionary input from user, python dict to json, python dict update, index value, how to delete columns from python dataframe, value of a dictionary
Useful Queries:
Adding and deleting values from ordereddict python
Adding and deleting values from ordereddict example
python ordereddict
ordereddict popitem last=false
convert dict to ordereddict
ordereddict add item
ordereddict vs dict
ordereddict move to end
#python4 #pythontutorial #pythonprogramming #python3 #viral #trending #pythonforbeginners #pythonlectures #pythonprograms #pythonlatest #rehanblogger #python4you #pythonlatestversion #pythonlatestversion Learn python3.12.0 and latest version of python3.13. If you are searching for python3.13.0 lessons, you are at the right place as this course will be very helpful for python learners or python beginners.
Комментарии