filmov
tv
Don’t Use the Wrong LINQ Methods
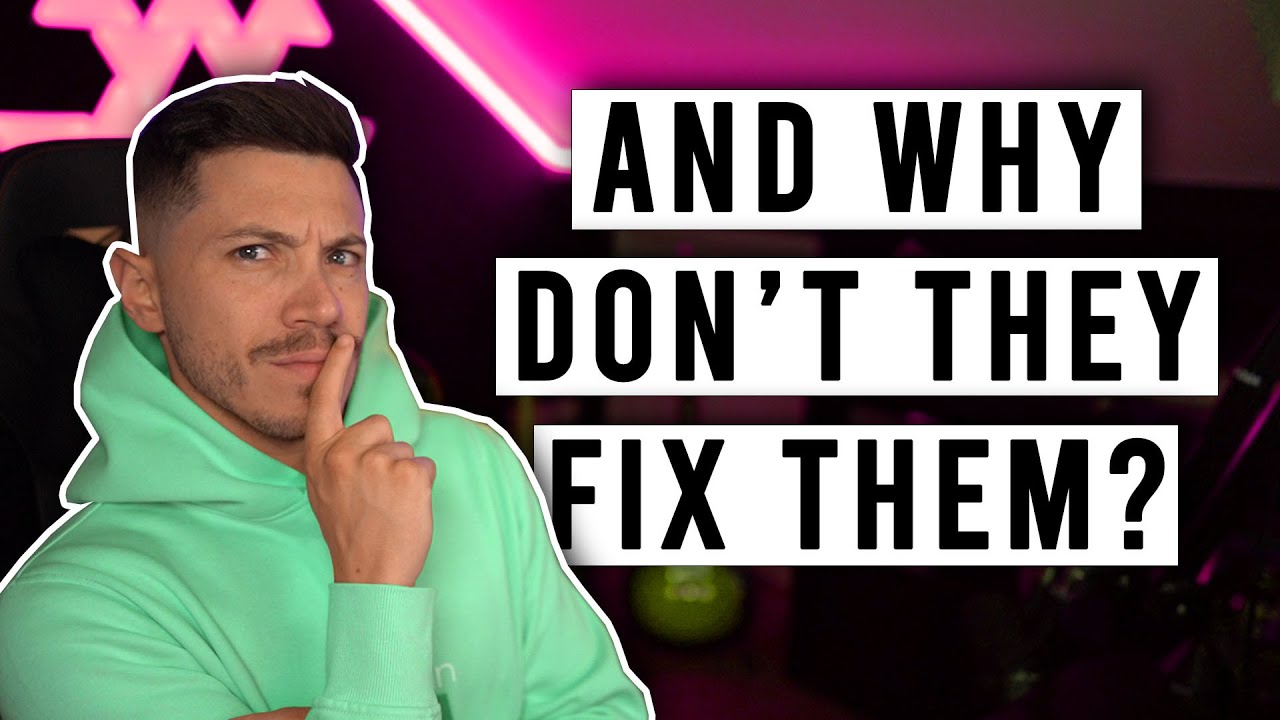
Показать описание
Hello, everybody, I'm Nick, and in this video, I will show you the difference between two LINQ-associated methods that look exactly the same but perform very differently.
Don't forget to comment, like and subscribe :)
Social Media:
#csharp #dotnet
Don’t Use the Wrong LINQ Methods
'Stop Using LINQ in .NET!'
Avoid these common mistakes with C#'s LINQ
When LINQ is not LINQ
The C# keyword you can ONLY use in LINQ, but carefully
When LINQ Makes You Write Worse .NET Code
'Always Use Any over Count in LINQ' | Code Cop #008
Which Shaft Flex Should YOU Use...Don't Go Wrong! (so many do)
How to Use Distinct in LINQ C# for Unique Data Retrieval Explained
Stop using LINQ to order your primitive collections in C#
Stop Using FirstOrDefault in .NET! | Code Cop #021
The biggest performance TRAP of LINQ in C# | .NET Tips 4
WHY IS LANA LOCKED UP IN DRESS TO IMPRESS?!
LINQ for Beginners 🚀 Full Course
Make Your LINQ Up to 10x Faster!
Must Watch 7 LINQ C# best practices in ASP.NET Core MVC (2 Minutes Quick Tips)
Should LINQ Be Banned from C#!?
SingleOrDefault or FirstOrDefault? When LINQ might harm you
What are the advantages & disadvantages of LINQ ?
Fixing the IQueryable Conversion Error in Your LINQ Query
Ling Fast Hand Tutorial 😱 - Mobile Legends
Lina took over.. DTI LANA LORE #roblox #dti
AVOID These MISTAKES In Black Myth Wukong 🤯👀
20 Dumb Rookie Mistakes to AVOID in Las Vegas! 😵
Комментарии