filmov
tv
Switch Statement Alternative
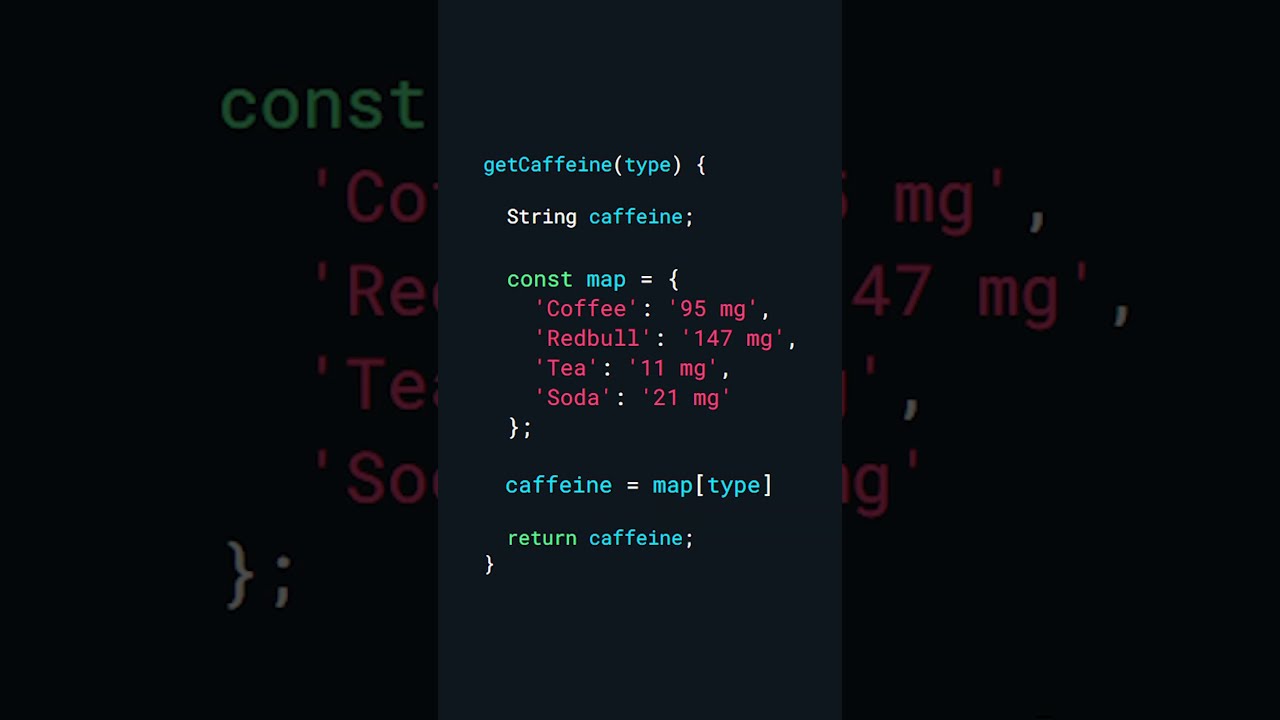
Показать описание
To simplify switch statements using an object literal technique, you can create an object where the keys represent the cases you want to handle, and the values are functions that should be executed for each case.
Here's a step-by-step guide on how to replace switch statements using an object literal technique:
Define an object where the keys correspond to the cases you want to handle.
For each key (case), assign a function or value that should be executed or returned when that case is encountered.
Instead of using a switch statement, you can use the object to look up and execute the appropriate function based on the case.
Here's an example:
// Traditional switch statement
function processFruit(fruit) {
switch (fruit) {
case "apple":
return "A fruit that is red or green.";
case "banana":
return "A fruit that is yellow.";
case "orange":
return "A fruit that is orange.";
default:
return "Unknown fruit.";
}
}
// Object literal
const fruitInfo = {
apple: "A fruit that is red or green.",
banana: "A fruit that is yellow.",
orange: "A fruit that is orange.",
default: "Unknown fruit.",
};
function processFruit(fruit) {
// Use the object literal to look up the information
}
#FlutterMapp
#Flutter
#coding
Here's a step-by-step guide on how to replace switch statements using an object literal technique:
Define an object where the keys correspond to the cases you want to handle.
For each key (case), assign a function or value that should be executed or returned when that case is encountered.
Instead of using a switch statement, you can use the object to look up and execute the appropriate function based on the case.
Here's an example:
// Traditional switch statement
function processFruit(fruit) {
switch (fruit) {
case "apple":
return "A fruit that is red or green.";
case "banana":
return "A fruit that is yellow.";
case "orange":
return "A fruit that is orange.";
default:
return "Unknown fruit.";
}
}
// Object literal
const fruitInfo = {
apple: "A fruit that is red or green.",
banana: "A fruit that is yellow.",
orange: "A fruit that is orange.",
default: "Unknown fruit.",
};
function processFruit(fruit) {
// Use the object literal to look up the information
}
#FlutterMapp
#Flutter
#coding
Комментарии