filmov
tv
How to Effectively Check for Existing Rows in Postgres with Golang: A Simple Solution
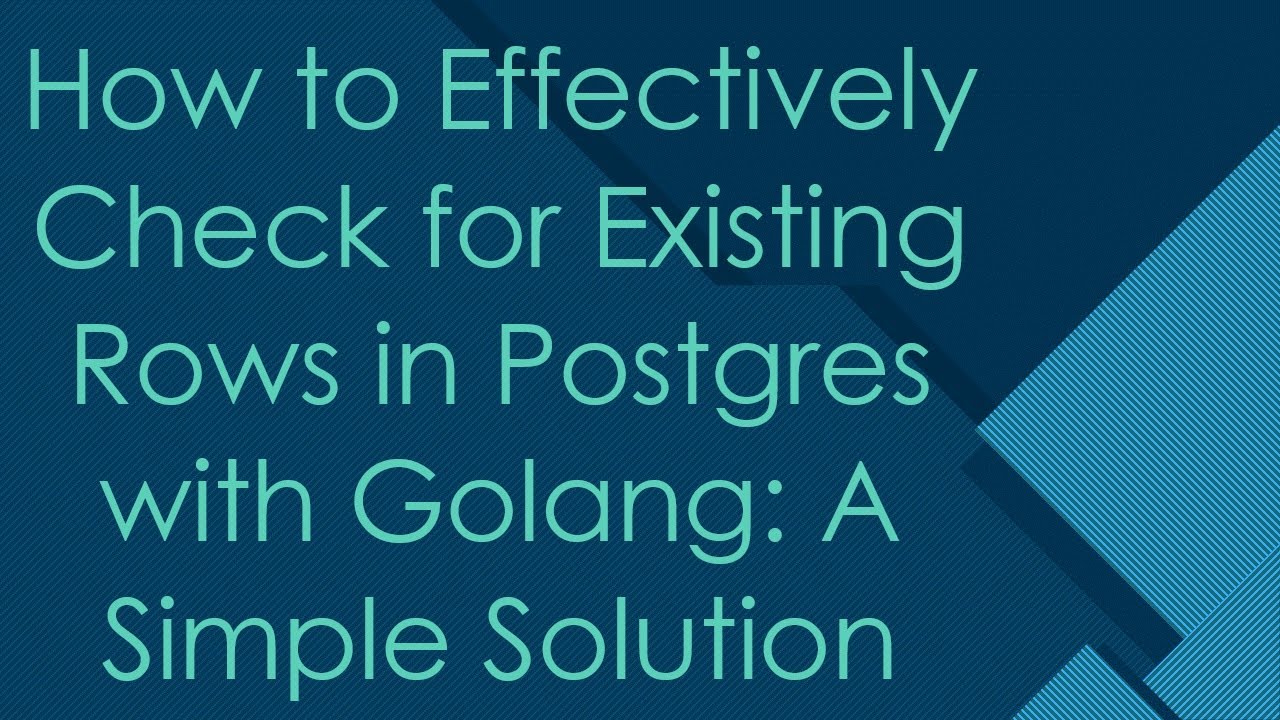
Показать описание
Discover how to resolve the issue of checking for existing rows in Postgres when using Golang for your Telegram bot registration process. Learn how to implement a unique index to simplify your database interactions.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: failed to check if row with value exists In Postgres with Golang
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Checking for Existing Users in PostgreSQL with Golang
Creating a registration feature in your application can often present challenges, especially when working with databases. If you're using Golang to develop a Telegram bot and PostgreSQL as a database, you may encounter situations where you need to verify the existence of user data. This is particularly important to ensure that users are not registered multiple times.
In this guide, we'll explore a common issue faced by developers: checking if a user's UUID (Universal Unique Identifier) already exists in the database. We'll break down the problem and provide a concise solution that will streamline your registration logic.
The Problem
In your bot, when a user sends the message "register," the bot is supposed to check if the user's UUID is already present in the database. If it doesn't exist, the bot will add a new entry for that user. However, you've encountered a problem where the bot is adding duplicate rows for the same UUID.
Here's a simplified flow of the code:
User sends the message "register."
The code checks if the UUID exists using the IsUserInDB function.
If the UUID is not found, it adds a new user entry.
However, upon subsequent registrations, even if the UUID exists, your function seems to wrongly return false, leading to duplicate entries.
The Current Implementation
Your function setup looks something like this:
[[See Video to Reveal this Text or Code Snippet]]
This code successfully checks for the existence of a user the first time, but fails on subsequent checks.
The Solution
Instead of manually checking if a UUID exists before inserting it into the database, a more effective approach is to use a unique index on the uuid column in your users table.
Implementing a Unique Index
To ensure that there are no duplicate UUIDs in your users table, you can create a unique index like this:
[[See Video to Reveal this Text or Code Snippet]]
How This Helps
Automatic Handling of Duplicates: Once you create a unique index, PostgreSQL will automatically handle the uniqueness of UUIDs for you. If you attempt to insert a UUID that already exists, an error will be returned without the need for a prior check.
Code Simplification: You can modify your AddUserToDB function to focus solely on adding the user without any checks beforehand:
[[See Video to Reveal this Text or Code Snippet]]
Error Handling: In your bot's logic, you can handle the error from the insertion attempt instead of checking the existence first:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By implementing a unique index on your users table, you simplify your database interactions and ensure data integrity. This solution not only resolves the issue of duplicate entries but also streamlines your code, making it cleaner and more efficient.
Embrace the power of unique constraints in PostgreSQL to enhance the reliability of your applications!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: failed to check if row with value exists In Postgres with Golang
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Checking for Existing Users in PostgreSQL with Golang
Creating a registration feature in your application can often present challenges, especially when working with databases. If you're using Golang to develop a Telegram bot and PostgreSQL as a database, you may encounter situations where you need to verify the existence of user data. This is particularly important to ensure that users are not registered multiple times.
In this guide, we'll explore a common issue faced by developers: checking if a user's UUID (Universal Unique Identifier) already exists in the database. We'll break down the problem and provide a concise solution that will streamline your registration logic.
The Problem
In your bot, when a user sends the message "register," the bot is supposed to check if the user's UUID is already present in the database. If it doesn't exist, the bot will add a new entry for that user. However, you've encountered a problem where the bot is adding duplicate rows for the same UUID.
Here's a simplified flow of the code:
User sends the message "register."
The code checks if the UUID exists using the IsUserInDB function.
If the UUID is not found, it adds a new user entry.
However, upon subsequent registrations, even if the UUID exists, your function seems to wrongly return false, leading to duplicate entries.
The Current Implementation
Your function setup looks something like this:
[[See Video to Reveal this Text or Code Snippet]]
This code successfully checks for the existence of a user the first time, but fails on subsequent checks.
The Solution
Instead of manually checking if a UUID exists before inserting it into the database, a more effective approach is to use a unique index on the uuid column in your users table.
Implementing a Unique Index
To ensure that there are no duplicate UUIDs in your users table, you can create a unique index like this:
[[See Video to Reveal this Text or Code Snippet]]
How This Helps
Automatic Handling of Duplicates: Once you create a unique index, PostgreSQL will automatically handle the uniqueness of UUIDs for you. If you attempt to insert a UUID that already exists, an error will be returned without the need for a prior check.
Code Simplification: You can modify your AddUserToDB function to focus solely on adding the user without any checks beforehand:
[[See Video to Reveal this Text or Code Snippet]]
Error Handling: In your bot's logic, you can handle the error from the insertion attempt instead of checking the existence first:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By implementing a unique index on your users table, you simplify your database interactions and ensure data integrity. This solution not only resolves the issue of duplicate entries but also streamlines your code, making it cleaner and more efficient.
Embrace the power of unique constraints in PostgreSQL to enhance the reliability of your applications!