filmov
tv
Insertion Sort in Python
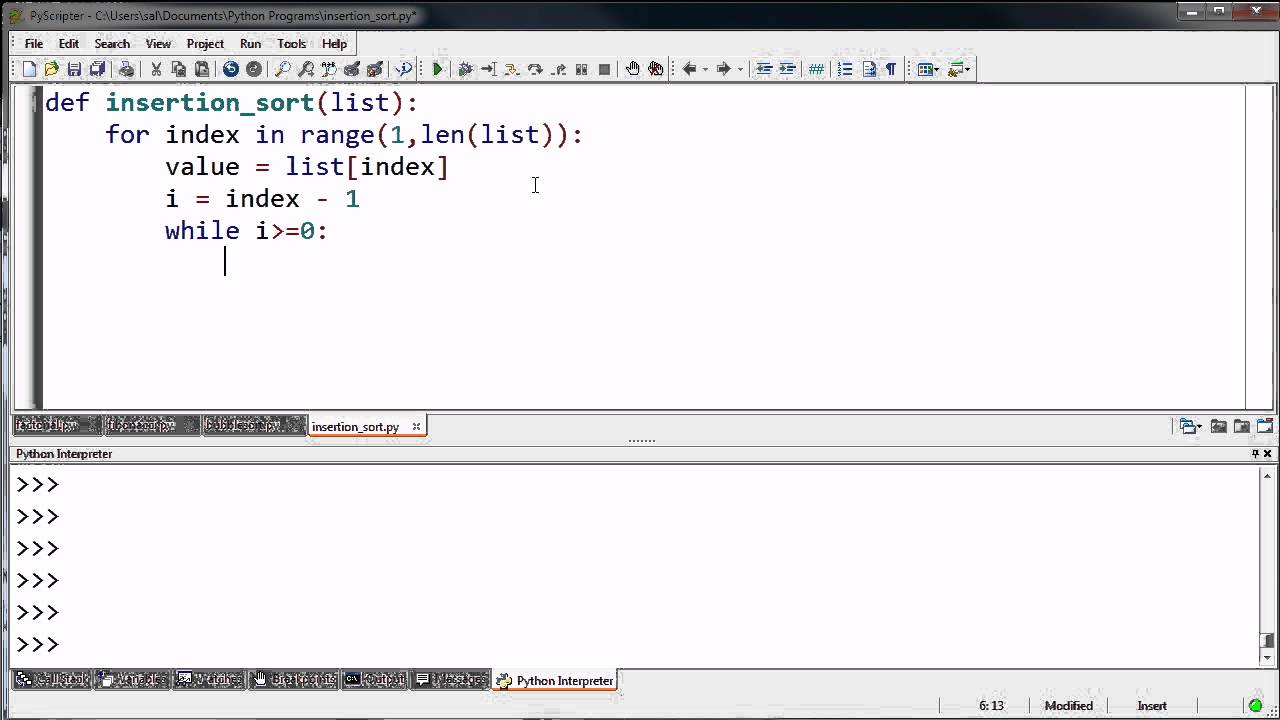
Показать описание
Basic implementation of insertion sort algorithm
Insertion Sort In Python Explained (With Example And Code)
Insertion Sort in Python
Insertion Sort Algorithm Explained (Full Code Included) - Python Algorithm Series for Beginners
Insertion Sort - Data Structures & Algorithms Tutorial Python #16
Insertion Sort in 2 min (Python)
Python Program #39 - Insertion Sort Algorithm in Python
Lec-44: INSERTION SORT in PYTHON 🐍 | DSA Concepts in Python 🐍
Learn Insertion Sort in 7 minutes 🧩
chal chal ke dikha🚶- Insertion Sort - BigOcodes #coding #algorithms #dsa #insertionsort
Insertion Sort mit Python
Insertion Sort List - Leetcode 147 - Python
#71 Python Tutorial for Beginners | Selection Sort using Python
Insertion sort in 2 minutes
Insertion Sort Python Tutorial for Beginners
Insertion Sort | DSA
Insertion Sort | Python Example
Python: Insertion Sort algorithm
python Insertion Sort algorithm
Insertion Sort Algorithm Made Simple [Sorting Algorithms]
Insertion sort algorithm explained in Python
Insertion Sort: Background & Python Code
insertion sort in python
Insertion Sort Code | DSA
Insertion Sort in Python | Class 12 Computer Science |
Комментарии