filmov
tv
Inheritance & polymorphism 💰👯♀ - How To Create Your Own ERC20 Cryptocurrency Token in 2021
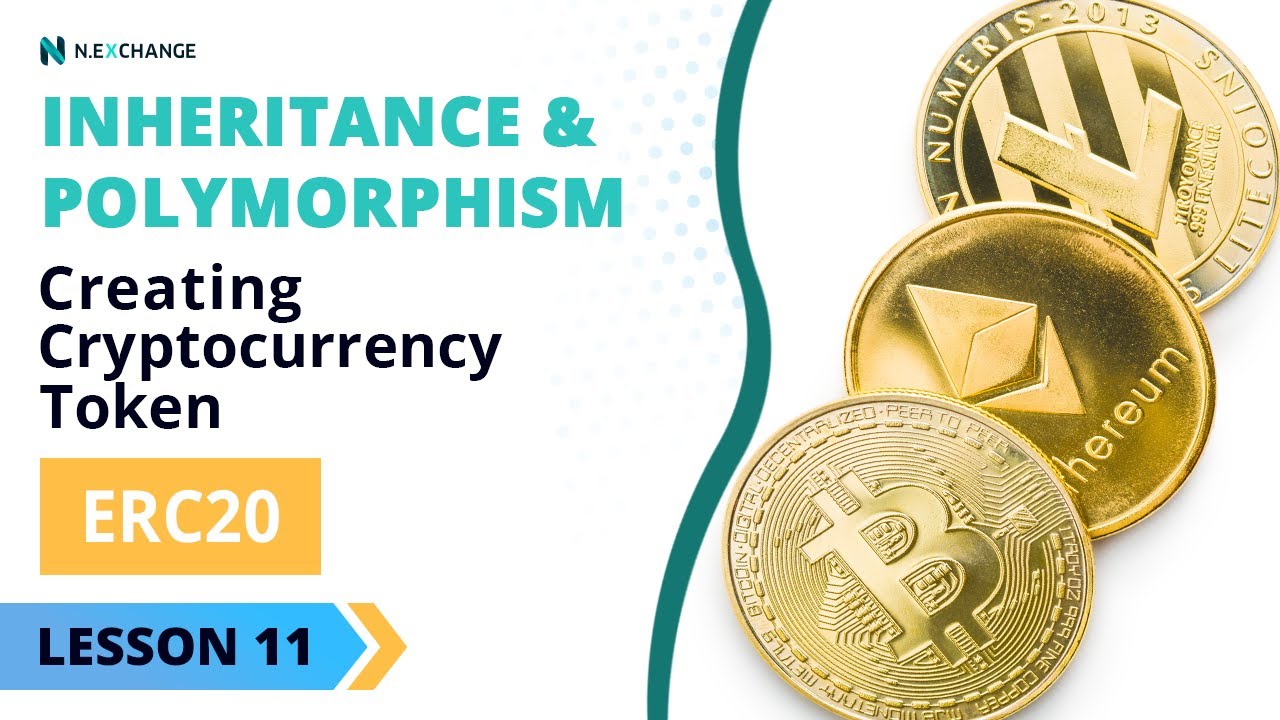
Показать описание
Visit our live chat to learn more about building your own Token and Exchange
🚀🚀🚀
[Free ERC20 Token Example]
Free examples of:
- ERC20 token contract
- Crowd sale contract
[Creators]
[Contest]
More details soon! Subscribe to stay updated!
How to create your own cryptocurrency token? -Smart contract development with Ethereum & Solidity
This crash course will teach you how to create and deploy your own smart contract in only 13 short lessons.
We will cover:
1. Intro
2. Required packages
3. Setup
4. Data types
5. Operators
6. Functions
7. Visibility
8. ERC20 Token Standard Interface (Yay!)
9.1. Implementing Your Own ERC20 Token (Hurray!)
9.2. Compiling & Deploying Your Own ERC20 Token (testnet) 🚀
10. ERC20 Token - Logging and Events
11. Inheritance and Polymorphism
12. Exceptions
13. Security Concerns
14.Deploying Your Own Token on The Ethereum Mainnet Network
[Lesson 1 - Intro]
[Solidity]
Solidity is a programming language for writing smart contracts that run on Ethereum Virtual Machine on Blockchain. It is a contract-oriented, high-level language.
[Before learning Solidity]
Prerequisites:
- JavaScript (variable assignments, loops, arrays, functions)
- Object-oriented programming concepts (classes, methods, static and instance variables)
- Command-line usage
Lesson 11 - Inheritance and polymorphism
In classical object-oriented programming inheritance refers to a case where subclass uses the structure and behavior of a superclass.
Polymorphism refers to changing the behavior of a superclass in the subclass.
The same principles apply to inheritance and polymorphism when developing Ethereum smart contracts, and the general inheritance system is very similar to Python’s or Java's, especially concerning multiple inheritance.
When a contract inherits from multiple contracts, only a single contract is created on the blockchain, and the code from all the base contracts is copied into the created contract.
Full example
pragma solidity ^0.4.18;
contract Owned {
address owner;
function Owned() {
}
}
// Use "is" to derive from another contract. Derived
// contracts can access all non-private members including
// internal functions and state variables. These cannot be
// accessed externally via `this`, though.
contract Mortal is Owned {
function kill() {
}
}
// Car has all the functionality of mortal contract which
// in turns holds all functionality for the owner contract
contract Car {
string make;
function Car(string _make) {
make = _make;
}
function getMake() public view returns(string) { return make; }
function makeNoise() public pure returns(string) { return "VROOOOM!!!"; }
}
contract Thing {
string color;
uint cost;
function Thing(string _color, uint _cost) {
color = _color;
cost = _cost;
}
function getColor() public view returns (string) { return color; }
function getCost() public view returns (uint) { return cost; }
}
contract ToyotaCar is Mortal, Thing, Car {
// Due to inheritance we inherit all the properties of Mortal, Thing and Car contracts
function ToyotaCar(string _color, uint _cost) Thing(_color, _cost) Car("Toyota") {
}
function setColor(string _color) { color = _color; }
// We can override Car methods via polymorphism
function makeNoise() public pure returns(string) { return "HAROOOM!!!"; }
}
Комментарии