filmov
tv
Advanced Inheritance & Polymorphism - example with Array of Objects (in-depth Data Structures & OOP)
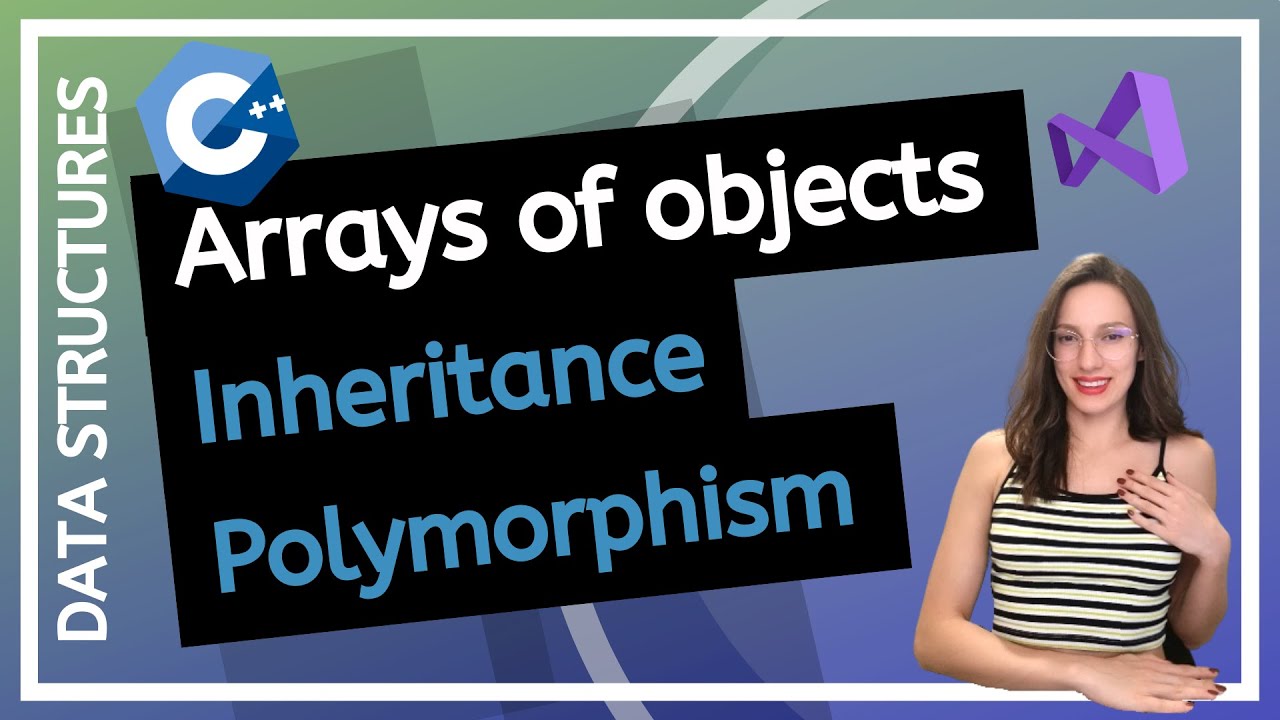
Показать описание
This video provides an in-depth explanation of the relationship between Arrays of objects and OOP concepts such as Inheritance and Polymorphism. It is a programming tutorial designed to teach you about data structures, object-oriented programming, and best practices for writing code. Through a practical example, you will learn how to create arrays that hold a collection of different objects. By the end of this coding exercise, you will have a solid understanding of how to implement parent-child relationships between classes and write reusable code. Additionally, you will learn how to implement polymorphic behavior and write efficient code.
To put your knowledge into practice, I have also prepared a practical task for you. This hands-on challenge will ensure that you understand these concepts and are able to use them in your own programming projects.
📚 Learn how to solve problems and build projects with these Free E-Books ⬇️
Experience the power of practical learning, gain career-ready skills, and start building real applications!
This is a step-by-step course designed to take you from beginner to expert in no time!
💰 Here is a coupon to save 10% on your first payment (CODEBEAUTY_YT10).
Use it quickly, because it will be available for a limited time.
I use it to enhance the performance, features, and support for C, C#, and C++ development in Visual Studio.
It is a powerful, secure text editor designed specifically for programmers.
However, please don't feel obligated to do so. I appreciate every one of you, and I will continue to share valuable content with you regardless of whether you choose to support me in this way. Thank you for being part of the Code Beauty community! ❤️😇
Related videos:
CONTENTS:
00:00 - What you will learn in this video?
02:06 - Explaining initial code
03:33 - What is Inheritance?
03:52 - Inheritance implementation
06:48 - What are virtual methods and abstract classes?
09:37 - What is polymorphism?
10:39 - Polymorphism implementation
15:23 - Memory deallocation
16:26 - How to deallocate memory?
17:48 - Static code analyzer
18:35 - Practical task for you
21:18 - Bloopers
Add me on:
*******CODE IS IN THE COMMENTS*******
To put your knowledge into practice, I have also prepared a practical task for you. This hands-on challenge will ensure that you understand these concepts and are able to use them in your own programming projects.
📚 Learn how to solve problems and build projects with these Free E-Books ⬇️
Experience the power of practical learning, gain career-ready skills, and start building real applications!
This is a step-by-step course designed to take you from beginner to expert in no time!
💰 Here is a coupon to save 10% on your first payment (CODEBEAUTY_YT10).
Use it quickly, because it will be available for a limited time.
I use it to enhance the performance, features, and support for C, C#, and C++ development in Visual Studio.
It is a powerful, secure text editor designed specifically for programmers.
However, please don't feel obligated to do so. I appreciate every one of you, and I will continue to share valuable content with you regardless of whether you choose to support me in this way. Thank you for being part of the Code Beauty community! ❤️😇
Related videos:
CONTENTS:
00:00 - What you will learn in this video?
02:06 - Explaining initial code
03:33 - What is Inheritance?
03:52 - Inheritance implementation
06:48 - What are virtual methods and abstract classes?
09:37 - What is polymorphism?
10:39 - Polymorphism implementation
15:23 - Memory deallocation
16:26 - How to deallocate memory?
17:48 - Static code analyzer
18:35 - Practical task for you
21:18 - Bloopers
Add me on:
*******CODE IS IN THE COMMENTS*******
Комментарии