filmov
tv
38 - How Inheritance Affects Java Serialization - Theory
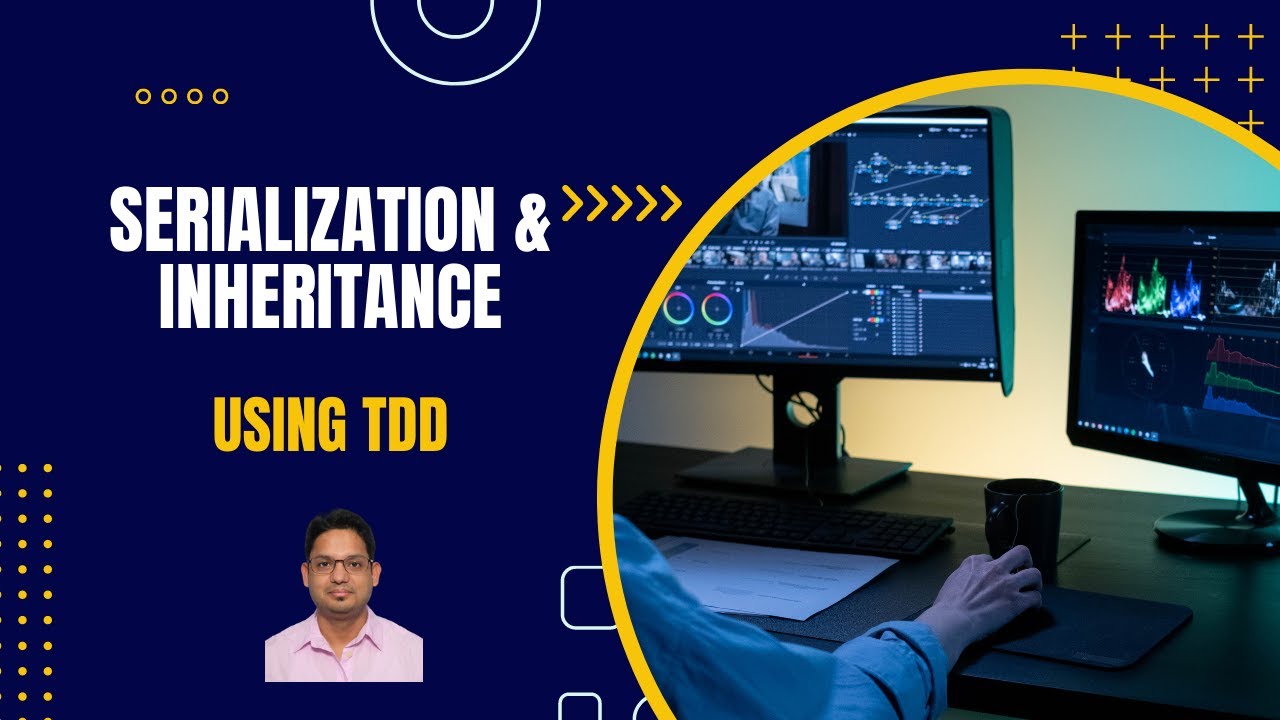
Показать описание
@backstreetbrogrammer
--------------------------------------------------------------------------------
Chapter 16 - How Inheritance Affects Java Serialization - Theory
--------------------------------------------------------------------------------
If a superclass is Serializable, then all subclasses are automatically Serializable without having to explicitly mark the subclass as Serializable.
If a class does NOT explicitly extend any other class and does NOT implement Serializable, then we can confirm that the class is NOT serializable as class Object does NOT implement Serializable.
Now suppose a subclass implements Serializable but the super class does NOT. How is this going to affect serialization?
When an object is constructed using new (as opposed to being deserialized), following things happen in this sequence:
1. All instance variables are assigned default values - like int as 0, double as 0D, boolean as false , String as null, etc.
2. The constructor is invoked, which immediately invokes the superclass constructor OR another overloaded constructor, until one of the overloaded constructors invokes the superclass constructor.
3. All superclass constructors complete.
4. Instance variables that are initialized as part of their declaration are assigned their initial value overriding the default values they’re given prior to the superclass constructors completing.
5. The constructor completes.
BUT, these things do NOT happen when an object is deserialized.
If the constructor were invoked, and/or variables were assigned the values given in their declarations, the object we are trying to restore would revert to its original state, rather than coming back reflecting the changes in its state that happened sometime after it was created.
In other words, we want only the values saved as part of the serialized state of the object to be reassigned.
If the superclass is not Serializable, the instance variables in the subclass (which implements Serializable) will be serialized and deserialized correctly, but the inherited variables from the non-serializable superclass will come back with their default/initially assigned values rather than the values they had at the time of serialization.
Thus, if a subclass implements Serializable but the super class does NOT, then any instance variables INHERITED from that superclass will be reset to the values they were given during the original construction of the object. This is because the non-serializable class constructor WILL run.
In fact, every constructor ABOVE the first non-serializable class constructor will also run in the inheritance tree.
#java #javadevelopers #javaprogramming #javaserialization
--------------------------------------------------------------------------------
Chapter 16 - How Inheritance Affects Java Serialization - Theory
--------------------------------------------------------------------------------
If a superclass is Serializable, then all subclasses are automatically Serializable without having to explicitly mark the subclass as Serializable.
If a class does NOT explicitly extend any other class and does NOT implement Serializable, then we can confirm that the class is NOT serializable as class Object does NOT implement Serializable.
Now suppose a subclass implements Serializable but the super class does NOT. How is this going to affect serialization?
When an object is constructed using new (as opposed to being deserialized), following things happen in this sequence:
1. All instance variables are assigned default values - like int as 0, double as 0D, boolean as false , String as null, etc.
2. The constructor is invoked, which immediately invokes the superclass constructor OR another overloaded constructor, until one of the overloaded constructors invokes the superclass constructor.
3. All superclass constructors complete.
4. Instance variables that are initialized as part of their declaration are assigned their initial value overriding the default values they’re given prior to the superclass constructors completing.
5. The constructor completes.
BUT, these things do NOT happen when an object is deserialized.
If the constructor were invoked, and/or variables were assigned the values given in their declarations, the object we are trying to restore would revert to its original state, rather than coming back reflecting the changes in its state that happened sometime after it was created.
In other words, we want only the values saved as part of the serialized state of the object to be reassigned.
If the superclass is not Serializable, the instance variables in the subclass (which implements Serializable) will be serialized and deserialized correctly, but the inherited variables from the non-serializable superclass will come back with their default/initially assigned values rather than the values they had at the time of serialization.
Thus, if a subclass implements Serializable but the super class does NOT, then any instance variables INHERITED from that superclass will be reset to the values they were given during the original construction of the object. This is because the non-serializable class constructor WILL run.
In fact, every constructor ABOVE the first non-serializable class constructor will also run in the inheritance tree.
#java #javadevelopers #javaprogramming #javaserialization